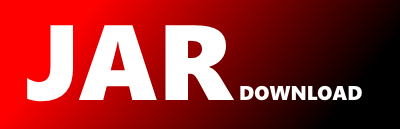
com.almondtools.util.graph.ReversePostOrderWorklistTraversal Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of rexlex Show documentation
Show all versions of rexlex Show documentation
Regular expression matchers, searcher, lexers based on deterministic finite automata
package com.almondtools.util.graph;
import java.util.HashSet;
import java.util.Iterator;
import java.util.LinkedHashSet;
import java.util.LinkedList;
import java.util.List;
import java.util.Set;
public abstract class ReversePostOrderWorklistTraversal extends AbstractTraversal implements Traversal {
private Class clazz;
private Set> visited;
private List> ordered;
private Set> worklist;
public ReversePostOrderWorklistTraversal(Graph graph, Class clazz) {
super(graph);
this.clazz = clazz;
this.visited = new HashSet>();
this.ordered = new LinkedList>();
}
@Override
public void traverse() {
super.traverse();
this.worklist = new LinkedHashSet>();
worklist.addAll(getGraph().getNodes());
while (!worklist.isEmpty()) {
if (worklist.size() == 1) {
Iterator> iterator = worklist.iterator();
GraphNode node = iterator.next();
iterator.remove();
visitGraphNodeWorklist(node);
} else {
for (GraphNode node : ordered) {
if (worklist.contains(node)) {
worklist.remove(node);
visitGraphNodeWorklist(node);
}
}
}
}
}
private void visitGraphNodeWorklist(GraphNode node) {
V oldData = getData(node, clazz);
visitGraphNode(node);
V newData = getData(node, clazz);
if (oldData == newData) {
return;
} else if (oldData == null) {
worklist.addAll(node.getSuccessors());
} else if (newData == null) {
worklist.addAll(node.getSuccessors());
} else if (oldData.equals(newData)) {
return;
} else {
worklist.addAll(node.getSuccessors());
}
}
@Override
public void traverseNode(GraphNode node) {
if (!visited.contains(node)) {
visited.add(node);
for (GraphNode next : node.getSuccessors()) {
next.apply(this);
}
record(node);
}
}
private void record(GraphNode node) {
ordered.add(0, node);
}
public abstract void visitGraphNode(GraphNode node);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy