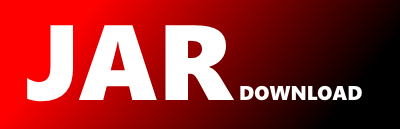
org.semanticweb.owlapi.model.OWLEntity Maven / Gradle / Ivy
The newest version!
/*
* This file is part of the OWL API.
*
* The contents of this file are subject to the LGPL License, Version 3.0.
*
* Copyright (C) 2011, The University of Manchester
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program. If not, see http://www.gnu.org/licenses/.
*
*
* Alternatively, the contents of this file may be used under the terms of the Apache License, Version 2.0
* in which case, the provisions of the Apache License Version 2.0 are applicable instead of those above.
*
* Copyright 2011, University of Manchester
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.semanticweb.owlapi.model;
import java.util.Set;
/**
* Author: Matthew Horridge
* The University Of Manchester
* Bio-Health Informatics Group
* Date: 24-Oct-2006
*
* Represents Entities in the OWL 2 Specification.
*
*/
public interface OWLEntity extends OWLObject, OWLNamedObject {
/**
* Gets the entity type for this entity
* @return The entity type
*/
EntityType> getEntityType();
/** Gets an entity that has the same IRI as this entity but is of the
* specified type.
*
* @param entityType
* The type of the entity to obtain. This entity is not affected
* in any way.
* @return An entity that has the same IRI as this entity and is of the
* specified type
* @deprecated use a datafactory instead */
@Deprecated
E getOWLEntity(EntityType entityType);
/**
* Tests to see if this entity is of the specified type
* @param entityType The entity type
* @return true
if this entity is of the specified type, otherwise false
.
*/
boolean isType(EntityType> entityType);
/**
* Gets the annotations for this entity. These are deemed to be annotations in annotation assertion
* axioms that have a subject that is an IRI that is equal to the IRI of this entity.
* @param ontology The ontology to be examined for annotation assertion axioms
* @return The annotations that participate directly in an annotation assertion whose subject is an
* IRI corresponding to the IRI of this entity.
*/
Set getAnnotations(OWLOntology ontology);
/**
* Obtains the annotations on this entity where the annotation has the specified
* annotation property.
* @param ontology The ontology to examine for annotation axioms
* @param annotationProperty The annotation property
* @return A set of OWLAnnotation
objects that have the specified
* URI.
*/
Set getAnnotations(OWLOntology ontology, OWLAnnotationProperty annotationProperty);
/**
* @param ontology the ontology to use
* @return the annotation assertion axioms about this entity in the provided ontology
*/
Set getAnnotationAssertionAxioms(OWLOntology ontology);
/** Determines if this entity is a built in entity. The entity is a built in
* entity if it is:
*
* - a class and the URI corresponds to owl:Thing or owl:Nothing
* - an object property and the URI corresponds to owl:topObjectProperty
* or owl:bottomObjectProperty
* - a data property and the URI corresponds to owl:topDataProperty or
* owl:bottomDataProperty
* - a datatype and the IRI is rdfs:Literal or is in the OWL 2 datatype
* map or is rdf:PlainLiteral
* - an annotation property and the URI is in the set of built in
* annotation property URIs, i.e. one of:
*
* - rdfs:label
* - rdfs:comment
* - rdfs:seeAlso
* - rdfs:isDefinedBy
* - owl:deprecated
* - owl:priorVersion
* - owl:backwardCompatibleWith
* - owl:incompatibleWith
*
*
*
*
* @return true
if this entity is a built in entity, or
* false
if this entity is not a builtin entity. */
boolean isBuiltIn();
/**
* A convenience method that determines if this entity is an OWLClass
* @return true
if this entity is an OWLClass, otherwise false
*/
boolean isOWLClass();
/**
* A convenience method that obtains this entity as an OWLClass (in order to
* avoid explicit casting).
* @return The entity as an OWLClass.
* @throws OWLRuntimeException if this entity is not an OWLClass (check with the
* isOWLClass method first).
*/
OWLClass asOWLClass();
/**
* A convenience method that determines if this entity is an OWLObjectProperty
* @return true
if this entity is an OWLObjectProperty, otherwise false
*/
boolean isOWLObjectProperty();
/**
* A convenience method that obtains this entity as an OWLObjectProperty (in order to
* avoid explicit casting).
* @return The entity as an OWLObjectProperty.
* @throws OWLRuntimeException if this entity is not an OWLObjectProperty (check with the
* isOWLObjectProperty method first).
*/
OWLObjectProperty asOWLObjectProperty();
/**
* A convenience method that determines if this entity is an OWLDataProperty
* @return true
if this entity is an OWLDataProperty, otherwise false
*/
boolean isOWLDataProperty();
/**
* A convenience method that obtains this entity as an OWLDataProperty (in order to
* avoid explicit casting).
* @return The entity as an OWLDataProperty.
* @throws OWLRuntimeException if this entity is not an OWLDataProperty (check with the
* isOWLDataProperty method first).
*/
OWLDataProperty asOWLDataProperty();
/**
* A convenience method that determines if this entity is an OWLNamedIndividual
* @return true
if this entity is an OWLNamedIndividual, otherwise false
*/
boolean isOWLNamedIndividual();
/**
* A convenience method that obtains this entity as an OWLNamedIndividual (in order to
* avoid explicit casting).
* @return The entity as an OWLNamedIndividual.
* @throws OWLRuntimeException if this entity is not an OWLIndividual (check with the
* isOWLIndividual method first).
*/
OWLNamedIndividual asOWLNamedIndividual();
/**
* A convenience method that determines if this entity is an OWLDatatype
* @return true
if this entity is an OWLDatatype, otherwise false
*/
boolean isOWLDatatype();
/**
* A convenience method that obtains this entity as an OWLDatatype (in order to
* avoid explicit casting).
* @return The entity as an OWLDatatype.
* @throws OWLRuntimeException if this entity is not an OWLDatatype (check with the
* isOWLDatatype method first).
*/
OWLDatatype asOWLDatatype();
/**
* A convenience method that determines if this entity is an OWLAnnotationProperty
* @return true
if this entity is an OWLAnnotationProperty, otherwise false
*/
boolean isOWLAnnotationProperty();
/**
* A convenience method that obtains this entity as an OWLAnnotationProperty (in order to
* avoid explicit casting).
* @return The entity as an OWLAnnotationProperty.
* @throws OWLRuntimeException if this entity is not an OWLAnnotationProperty
*/
OWLAnnotationProperty asOWLAnnotationProperty();
/**
* Returns a string representation that can be used as the ID of this entity. This is the toString
* representation of the IRI
* @return A string representing the toString of the IRI of this entity.
*/
String toStringID();
/**
* Gets the axioms in the specified ontology that contain this entity in their signature.
* @param ontology The ontology that will be searched for axioms
* @return The axioms in the specified ontology whose signature contains this entity.
*/
Set getReferencingAxioms(OWLOntology ontology);
/**
* Gets the axioms in the specified ontology and possibly its imports closure that contain this entity in their
* signature.
* @param ontology The ontology that will be searched for axioms
* @param includeImports If true
then axioms in the imports closure will also be returned, if
* false
then only the axioms in the specified ontology will be returned.
* @return The axioms in the specified ontology whose signature contains this entity.
*/
Set getReferencingAxioms(OWLOntology ontology, boolean includeImports);
@SuppressWarnings("javadoc")
void accept(OWLEntityVisitor visitor);
@SuppressWarnings("javadoc")
O accept(OWLEntityVisitorEx visitor);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy