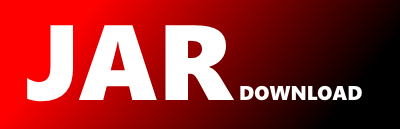
org.semanticweb.owlapi.model.OWLOntology Maven / Gradle / Ivy
The newest version!
/*
* This file is part of the OWL API.
*
* The contents of this file are subject to the LGPL License, Version 3.0.
*
* Copyright (C) 2011, The University of Manchester
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program. If not, see http://www.gnu.org/licenses/.
*
*
* Alternatively, the contents of this file may be used under the terms of the Apache License, Version 2.0
* in which case, the provisions of the Apache License Version 2.0 are applicable instead of those above.
*
* Copyright 2011, University of Manchester
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.semanticweb.owlapi.model;
import java.util.Set;
/**
* Author: Matthew Horridge
The University Of Manchester
Bio-Health Informatics Group Date: 24-Oct-2006
*
* Represents an OWL 2 Ontology in the OWL 2 specification.
*
* An OWLOntology
consists of a possibly empty set of {@link org.semanticweb.owlapi.model.OWLAxiom}s
* and a possibly empty set of {@link OWLAnnotation}s. An ontology can have an ontology IRI which can be used to
* identify the ontology. If it has an ontology IRI then it may also have an ontology version IRI. Since OWL 2, an
* ontology need not have an ontology IRI. (See the OWL
* 2 Structural Specification).
*
* An ontology cannot be modified directly. Changes must be applied via its OWLOntologyManager
.
*/
public interface OWLOntology extends OWLObject {
// XXX when the interfce changes, uncomment this
//void accept(OWLNamedObjectVisitor visitor);
/**
* Gets the manager that created this ontology. The manager is used by various methods on OWLOntology
* to resolve imports
*
* @return The manager that created this ontology.
*/
OWLOntologyManager getOWLOntologyManager();
/**
* Gets the identity of this ontology (i.e. ontology IRI + version IRI).
*
* @return The ID of this ontology.
*/
OWLOntologyID getOntologyID();
/**
* Determines whether or not this ontology is anonymous. An ontology is anonymous if it does not have an ontology
* IRI.
*
* @return true
if this ontology is anonymous, otherwise false
*/
boolean isAnonymous();
/**
* Gets the annotations on this ontology.
*
* @return A set of annotations on this ontology. The set returned will be a copy - modifying the set will have
* no effect on the annotations in this ontology, similarly, any changes that affect the annotations on this
* ontology will not change the returned set.
*/
Set getAnnotations();
//////////////////////////////////////////////////////////////////////////////////////////////
//
// Imported ontologies
//
//////////////////////////////////////////////////////////////////////////////////////////////
/**
* Gets the set of document IRIs that are directly imported by this ontology.
* This corresponds to the IRIs defined by the directlyImportsDocument association as discussed in Section 3.4 of the
* OWL 2 Structural specification.
*
* @return The set of directlyImportsDocument IRIs.
*
* @throws UnknownOWLOntologyException If this ontology is no longer managed by its manager because it was
* removed from the manager.
*/
Set getDirectImportsDocuments() throws UnknownOWLOntologyException;
/**
* Gets the set of loaded ontologies that this ontology is related to via the
* directlyImports relation. See Section 3.4 of the OWL 2 specification for the definition of the directlyImports
* relation.
*
* Note that there may be fewer ontologies in the set returned by this method than there are IRIs in the set returned by the
* {@link #getDirectImportsDocuments()} method. This will be the case if some of the ontologies that are directly imported by this ontology
* are not loaded for what ever reason.
*
*
* @return A set of ontologies such that for this ontology O, and each ontology O' in the set, (O, O') is in the
* directlyImports relation.
*
* @throws UnknownOWLOntologyException If this ontology is no longer managed by its manager because it was removed
* from the manager.
*/
Set getDirectImports() throws UnknownOWLOntologyException;
/**
* Gets the set of loaded ontologies that this ontology is related to via the
* transitive closure of the directlyImports relation.
*
* For example, if this ontology imports ontology B, and ontology B imports ontology C, then this method will return the set consisting of
* ontology B and ontology C.
*
*
* @return The set of ontologies that this ontology is related to via the transitive closure of the directlyImports
* relation. The set that is returned is a copy - it will not be updated if
* the ontology changes. It is therefore safe to apply changes to this ontology while iterating over this set.
*
* @throws UnknownOWLOntologyException if this ontology is no longer managed by its manager because it was removed
* from the manager.
*/
Set getImports() throws UnknownOWLOntologyException;
/**
* Gets the set of loaded ontologies that this ontology is related to via the
* reflexive transitive closure of the directlyImports relation
* as defined in Section 3.4 of the OWL 2 Structural Specification. (i.e. The set returned
* includes all ontologies returned by the {@link #getImports()} method plus this ontology.)
*
*
* For example, if this ontology imports ontology B, and ontology B imports ontology C, then this method will return the set consisting of
* this ontology, ontology B and ontology C.
*
*
* @return The set of ontologies in the reflexive transitive closure of the directlyImports relation.
*
* @throws UnknownOWLOntologyException If this ontology is no longer managed by its manager because it was removed
* from the manager.
*/
Set getImportsClosure() throws UnknownOWLOntologyException;
/**
* Gets the set of imports declarations for this ontology. The set returned represents the set of IRIs that
* correspond to the set of IRIs in an ontology's directlyImportsDocuments (see Section 3 in the OWL 2 structural
* specification).
*
* @return The set of imports declarations that correspond to the set of ontology document IRIs that are directly
* imported by this ontology.
* The set that is returned is a copy - it will not be updated if the ontology changes. It is therefore
* safe to apply changes to this ontology while iterating over this set.
*/
Set getImportsDeclarations();
///////////////////////////////////////////////////////////////////////////////////////////////
//
// Methods to retrive class, property and individual axioms
//
///////////////////////////////////////////////////////////////////////////////////////////////
/**
* Determines if this ontology is empty - an ontology is empty if it does not contain
* any axioms (i.e. {@link #getAxioms()} returns the empty set), and it does not have any annotations (i.e.
* {@link #getAnnotations()} returns the empty set).
*
* @return true
if the ontology is empty, otherwise false
.
*/
boolean isEmpty();
/**
* Retrieves all of the axioms in this ontology. Note that to test whether or not this ontology is empty (i.e. contains
* no axioms, the isEmpty method is preferred over getAxioms().isEmpty(). )
*
* @return The set of all axioms in this ontology, including logical axioms and annotation axioms. The set that is
* returned is a copy of the axioms in the ontology - it will not be updated if the ontology changes. It is
* recommended that the containsAxiom
method is used to determine whether or not this ontology
* contains a particular axiom rather than using getAxioms().contains().
*/
Set getAxioms();
/**
* Gets the number of axioms in this ontology.
*
* @return The number of axioms in this ontology.
*/
int getAxiomCount();
/**
* Gets all of the axioms in the ontology that affect the logical meaning of the ontology. In other words, this
* method returns all axioms that are not annotation axioms, or declaration axioms.
*
* @return A set of axioms which are of the type OWLLogicalAxiom
The set that is returned is a copy of
* the axioms in the ontology - it will not be updated if the ontology changes.
*/
Set getLogicalAxioms();
/**
* Gets the number of logical axioms in this ontology.
*
* @return The number of axioms in this ontology.
*/
int getLogicalAxiomCount();
/**
* Gets the axioms which are of the specified type.
*
* @param axiomType The type of axioms to be retrived.
* @return A set containing the axioms which are of the specified type. The set that is returned is a copy of the
* axioms in the ontology - it will not be updated if the ontology changes.
*/
Set getAxioms(AxiomType axiomType);
/**
* Gets the axioms which are of the specified type.
*
* @param axiomType The type of axioms to be retrived.
* @param includeImportsClosure if true
then axioms of the specified type will also be retrieved from
* the imports closure of this ontology, if false
then axioms of the specified type will only
* be retrieved from this ontology.
* @return A set containing the axioms which are of the specified type. The set that is returned is a copy of the
* axioms in the ontology (and its imports closure) - it will not be updated if the ontology changes.
*/
Set getAxioms(AxiomType axiomType, boolean includeImportsClosure);
/**
* Gets the axioms that form the TBox for this ontology, i.e., the ones whose type is in the AxiomType::TBoxAxiomTypes
*
*
* @param includeImportsClosure if true
then axioms of the specified type will also be retrieved from
* the imports closure of this ontology, if false
then axioms of the specified type will only
* be retrieved from this ontology.
* @return A set containing the axioms which are of the specified type. The set that is returned is a copy of the
* axioms in the ontology (and its imports closure) - it will not be updated if the ontology changes.
*/
Set getTBoxAxioms(boolean includeImportsClosure);
/**
* Gets the axioms that form the ABox for this ontology, i.e., the ones whose type is in the AxiomType::ABoxAxiomTypes
*
* @param includeImportsClosure if true
then axioms of the specified type will also be retrieved from
* the imports closure of this ontology, if false
then axioms of the specified type will only
* be retrieved from this ontology.
* @return A set containing the axioms which are of the specified type. The set that is returned is a copy of the
* axioms in the ontology (and its imports closure) - it will not be updated if the ontology changes.
*/
Set getABoxAxioms(boolean includeImportsClosure);
/**
* Gets the axioms that form the RBox for this ontology, i.e., the ones whose type is in the AxiomType::RBoxAxiomTypes
*
* @param includeImportsClosure if true
then axioms of the specified type will also be retrieved from
* the imports closure of this ontology, if false
then axioms of the specified type will only
* be retrieved from this ontology.
* @return A set containing the axioms which are of the specified type. The set that is returned is a copy of the
* axioms in the ontology (and its imports closure) - it will not be updated if the ontology changes.
*/
Set getRBoxAxioms(boolean includeImportsClosure);
/**
* Gets the axiom count of a specific type of axiom
*
* @param axiomType The type of axiom to count
* @return The number of the specified types of axioms in this ontology
*/
int getAxiomCount(AxiomType axiomType);
/**
* Gets the axiom count of a specific type of axiom, possibly in the imports closure of this ontology
*
* @param axiomType The type of axiom to count
* @param includeImportsClosure Specifies that the imports closure should be included when counting axioms
* @return The number of the specified types of axioms in this ontology
*/
int getAxiomCount(AxiomType axiomType, boolean includeImportsClosure);
/**
* Determines if this ontology contains the specified axiom.
*
* @param axiom The axiom to test for.
* @return true
if the ontology contains the specified axioms, or false
if the ontology
* doesn't contain the specified axiom.
*/
boolean containsAxiom(OWLAxiom axiom);
/**
* Determines if this ontology, and possibly the imports closure, contains the specified axiom.
*
* @param axiom The axiom to test for.
* @param includeImportsClosure if true
the imports closure of this ontology will be searched for the
* specific axiom, if false
just this ontology will be searched.
* @return true
if the ontology contains the specified axioms, or false
if the ontology
* doesn't contain the specified axiom.
*/
boolean containsAxiom(OWLAxiom axiom, boolean includeImportsClosure);
/**
* Determines if this ontology contains the specified axiom, but ignoring any annotations on this
* axiom. For example, if the ontology contains SubClassOf(Annotation(p V) A B)
then this method
* will return true
if the ontology contains SubClassOf(A B)
or
* SubClassOf(Annotation(q S) A B)
for any annotation property q
and any annotation
* value S
.
*
* @param axiom The axiom to test for.
* @return true
if this ontology contains this axiom with or without annotations.
*/
boolean containsAxiomIgnoreAnnotations(OWLAxiom axiom);
/**
* Gets the set of axioms contained in this ontology that have the same "logical structure" as the specified axiom.
*
* @param axiom The axiom that specifies the logical structure of the axioms to retrieve. If this axiom is annotated
* then the annotations are ignored.
* @return A set of axioms such that for any two axioms, axiomA
and
* axiomB
in the set, axiomA.getAxiomWithoutAnnotations()
is equal to
* axiomB.getAxiomWithoutAnnotations()
. The specified axiom will be contained in the set.
*/
Set getAxiomsIgnoreAnnotations(OWLAxiom axiom);
/**
* Gets the set of axioms contained in this ontology that have the same "logical structure" as the specified axiom, possibly searching
* the imports closure of this ontology.
*
* @param axiom The axiom that specifies the logical structure of the axioms to retrieve. If this axiom is annotated
* then the annotations are ignored.
* @param includeImportsClosure if true
then axioms in the imports closure of this ontology are returned,
* if false
only axioms in this ontology will be returned.
* @return A set of axioms such that for any two axioms, axiomA
and
* axiomB
in the set, axiomA.getAxiomWithoutAnnotations()
is equal to
* axiomB.getAxiomWithoutAnnotations()
. The specified axiom will be contained in the set.
*/
Set getAxiomsIgnoreAnnotations(OWLAxiom axiom, boolean includeImportsClosure);
/**
* Determines if this ontology and possibly its imports closure contains the specified axiom but
* ignoring any annotations on this axiom. For example, if the ontology contains
* SubClassOf(Annotation(p V) A B)
then this method
* will return true
if the ontology contains SubClassOf(A B)
or
* SubClassOf(Annotation(q S) A B)
for any annotation property q
and any annotation
* value S
.
*
* @param axiom The axiom to test for.
* @param includeImportsClosure if true
the imports closure of this ontology will be searched for the
* specified axiom. If false
only this ontology will be searched for the specifed axiom.
* @return true
if this ontology contains this axiom with or without annotations.
*/
boolean containsAxiomIgnoreAnnotations(OWLAxiom axiom, boolean includeImportsClosure);
/**
* Gets the set of general axioms in this ontology. This includes: - Subclass axioms that have a complex
* class as the subclass
- Equivalent class axioms that don't contain any named classes
* (
OWLClass
es) - Disjoint class axioms that don't contain any named classes
* (
OWLClass
es)
*
* @return The set that is returned is a copy of the axioms in the ontology - it will not be updated if the ontology
* changes. It is therefore safe to apply changes to this ontology while iterating over this set.
*/
Set getGeneralClassAxioms();
//////////////////////////////////////////////////////////////////////////////////////////////
//
// References/usage
//
//////////////////////////////////////////////////////////////////////////////////////////////
/**
* Gets the entities that are in the signature of this ontology. The signature of an ontology is the set of
* entities that are used to build axioms and annotations in the ontology.
* (See The OWL 2 Structural Specification)
*
* @return A set of OWLEntity
objects. The set that is returned is a copy - it will not be updated if
* the ontology changes. It is therefore safe to apply changes to this ontology while iterating over this
* set.
* @see #getClassesInSignature()
* @see #getObjectPropertiesInSignature()
* @see #getDataPropertiesInSignature()
* @see #getIndividualsInSignature()
*/
@Override
Set getSignature();
/**
* Gets the entities that are in the signature of this ontology. The signature of an ontology is the set of
* entities that are used to build axioms and annotations in the ontology.
* (See The OWL 2 Structural Specification)
*
* @param includeImportsClosure Specifies whether or not the returned set of entities should represent the signature
* of just this ontology, or the signature of the imports closure of this ontology.
* @return A set of OWLEntity
objects. The set that is returned is a copy - it will not be updated if
* the ontology changes. It is therefore safe to apply changes to this ontology while iterating over this
* set.
* @see #getClassesInSignature()
* @see #getObjectPropertiesInSignature()
* @see #getDataPropertiesInSignature()
* @see #getIndividualsInSignature()
*/
Set getSignature(boolean includeImportsClosure);
/**
* Gets the classes that are in the signature of this ontology.
* @see #getSignature()
* @return A set of named classes, which are referenced by any axiom in this ontology. The set that is returned is a
* copy - it will not be updated if the ontology changes. It is therefore safe to apply changes to this
* ontology while iterating over this set.
*/
@Override
Set getClassesInSignature();
/**
* Gets the classes that are in the signature of this ontology, and possibly the imports closure of this
* ontology.
*
* @param includeImportsClosure Specifies whether classes should be drawn from the signature of just this ontology or the
* imports closure of this ontology. If true
then the set of classes returned will correspond to the union
* of the classes in the signatures of the ontologies in the imports closure of this ontology. If false
* then the set of classes returned will correspond to the classes that are in the signature of this just this ontology.
* @return A set of classes that are in the signature of this ontology and possibly the union of the signatures of
* the ontologies in the imports closure of this ontology. The set that is returned is a
* copy - it will not be updated if the ontology changes. It is therefore safe to apply changes to this
* ontology while iterating over this set.
*/
Set getClassesInSignature(boolean includeImportsClosure);
/**
* Gets the object properties that are in the signature of this ontology.
* @see #getSignature()
* @return A set of object properties which are in the signature of this ontology. The set that is returned
* is a copy - it will not be updated if the ontology changes. It is therefore safe to apply changes to
* this ontology while iterating over this set.
*/
@Override
Set getObjectPropertiesInSignature();
/**
* Gets the object properties that are in the signature of this ontology, and possibly the imports closure of this
* ontology.
*
* @param includeImportsClosure Specifies whether object properties should be drawn from the signature of just this ontology or the
* imports closure of this ontology. If true
then the set of object properties returned will correspond to the union
* of the object properties in the signatures of the ontologies in the imports closure of this ontology. If false
* then the set of object properties returned will correspond to the object properties that are in the signature of this just this ontology.
* @return A set of object properties that are in the signature of this ontology and possibly the union of the signatures of
* the ontologies in the imports closure of this ontology. The set that is returned is a
* copy - it will not be updated if the ontology changes. It is therefore safe to apply changes to this
* ontology while iterating over this set.
*/
Set getObjectPropertiesInSignature(boolean includeImportsClosure);
/**
* Gets the data properties that are in the signature of this ontology.
* @see #getSignature()
* @return A set of data properties, which are in the signature of this ontology. The set that is returned is
* a copy - it will not be updated if the ontology changes. It is therefore safe to apply changes to this
* ontology while iterating over this set.
*/
@Override
Set getDataPropertiesInSignature();
/**
* Gets the data properties that are in the signature of this ontology, and possibly the imports closure of this
* ontology.
*
* @param includeImportsClosure Specifies whether data properties should be drawn from the signature of just this ontology or the
* imports closure of this ontology. If true
then the set of data properties returned will correspond to the union
* of the data properties in the signatures of the ontologies in the imports closure of this ontology. If false
* then the set of data properties returned will correspond to the data properties that are in the signature of this just this ontology.
* @return A set of data properties that are in the signature of this ontology and possibly the union of the signatures of
* the ontologies in the imports closure of this ontology. The set that is returned is a
* copy - it will not be updated if the ontology changes. It is therefore safe to apply changes to this
* ontology while iterating over this set.
*/
Set getDataPropertiesInSignature(boolean includeImportsClosure);
/**
* Gets the individuals that are in the signature of this ontology.
* @see #getSignature()
* @return A set of individuals, which are in the signature of this ontology. The set that is returned is a
* copy - it will not be updated if the ontology changes. It is therefore safe to apply changes to this
* ontology while iterating over this set.
*/
@Override
Set getIndividualsInSignature();
/**
* Gets the individuals that are in the signature of this ontology, and possibly the imports closure of this
* ontology.
* @see #getSignature()
* @param includeImportsClosure Specifies whether individuals should be drawn from the signature of just this ontology or the
* imports closure of this ontology. If true
then the set of individuals returned will correspond to the union
* of the individuals in the signatures of the ontologies in the imports closure of this ontology. If false
* then the set of individuals returned will correspond to the individuals that are in the signature of this just this ontology.
* @return A set of individuals that are in the signature of this ontology and possibly the union of the signatures of
* the ontologies in the imports closure of this ontology. The set that is returned is a
* copy - it will not be updated if the ontology changes. It is therefore safe to apply changes to this
* ontology while iterating over this set.
*/
Set getIndividualsInSignature(boolean includeImportsClosure);
/**
* Gets the referenced anonymous individuals
*
* @return The set of referenced anonymous individuals
*/
Set getReferencedAnonymousIndividuals();
/**
* Gets the datatypes that are in the signature of this ontology.
* @see #getSignature()
* @return A set of datatypes, which are in the signature of this ontology. The set that is returned is a
* copy - it will not be updated if the ontology changes. It is therefore safe to apply changes to this
* ontology while iterating over this set.
*/
@Override
Set getDatatypesInSignature();
/**
* Gets the datatypes that are in the signature of this ontology, and possibly the imports closure of this
* ontology.
* @see #getSignature()
* @param includeImportsClosure Specifies whether datatypes should be drawn from the signature of just this ontology or the
* imports closure of this ontology. If true
then the set of datatypes returned will correspond to the union
* of the datatypes in the signatures of the ontologies in the imports closure of this ontology. If false
* then the set of datatypes returned will correspond to the datatypes that are in the signature of this just this ontology.
* @return A set of datatypes that are in the signature of this ontology and possibly the union of the signatures of
* the ontologies in the imports closure of this ontology. The set that is returned is a
* copy - it will not be updated if the ontology changes. It is therefore safe to apply changes to this
* ontology while iterating over this set.
*/
Set getDatatypesInSignature(boolean includeImportsClosure);
/**
* Gets the annotation properties that are in the signature of this ontology.
* @see #getSignature()
* @return A set of annotation properties, which are in the signature of this ontology. The set that is returned is a
* copy - it will not be updated if the ontology changes. It is therefore safe to apply changes to this
* ontology while iterating over this set.
*/
Set getAnnotationPropertiesInSignature();
/**
* Gets the axioms where the specified entity appears in the signature of the axiom. The set that is returned,
* contains all axioms that directly reference the specified entity.
*
* @param owlEntity The entity that should be directly referred to by an axiom that appears in the results set.
* @return The set that is returned is a copy - it will not be updated if the ontology changes. It is therefore
* safe to apply changes to this ontology while iterating over this set.
*/
Set getReferencingAxioms(OWLEntity owlEntity);
/**
* Gets the axioms where the specified entity appears in the signature of the axiom. The set that is returned,
* contains all axioms that directly reference the specified entity.
*
* @param owlEntity The entity that should be directly referred to by an axiom that appears in the results set.
* @param includeImportsClosure Specifies if the axioms returned should just be from this ontology, or from the
* imports closure of this ontology. If true
the axioms returned will be from the imports closure
* of this ontology, if false
the axioms returned will just be from this ontology.
* @return The set that is returned is a copy - it will not be updated if the ontology changes. It is therefore
* safe to apply changes to this ontology while iterating over this set.
*/
Set getReferencingAxioms(OWLEntity owlEntity, boolean includeImportsClosure);
/**
* Gets the axioms that reference the specified anonymous individual
*
* @param individual The individual
* @return The axioms that reference the specified anonymous individual
*/
Set getReferencingAxioms(OWLAnonymousIndividual individual);
/**
* Determines if the signature of the ontology contains the specified entity.
*
* @param owlEntity The entity
* @return true
if the signature of this ontology contains owlEntity
, otherwise
* false
.
*/
boolean containsEntityInSignature(OWLEntity owlEntity);
/**
* Determines if the signature of this ontology, and possibly the signature of any of the ontologies in the imports
* closure of this ontology, contains the specified entity.
*
* @param owlEntity The entity
* @param includeImportsClosure Specifies whether the imports closure should be examined for the entity reference
* or not.
* @return true
if the ontology contains a reference to the specified entity, otherwise
* false
The set that is returned is a copy - it will not be updated if the ontology changes.
* It is therefore safe to apply changes to this ontology while iterating over this set.
*/
boolean containsEntityInSignature(OWLEntity owlEntity, boolean includeImportsClosure);
/**
* Determines if the signature of this ontology contains a class, object property, data property, named individual,
* annotation property or datatype with the specified IRI.
* @param entityIRI The IRI to test for.
* @return true
if the signature of this ontology contains a class, object property, data property,
* named individual, annotation property or datatype with the specified IRI.
*/
boolean containsEntityInSignature(IRI entityIRI);
/**
* Determines if the signature of this ontology and possibly its imports closure contains a class, object property,
* data property, named individual, annotation property or datatype with the specified IRI.
* @param entityIRI The IRI to test for.
* @param includeImportsClosure Specifies whether the imports closure of this ontology should be examined or not.
* @return If includeImportsClosure=true
then returns
* true
if the signature of this ontology or the signature of an ontology in the imports closure of this
* ontology contains a class, object property, data property,
* named individual, annotation property or datatype with the specified IRI. If includeImportsClosure=false
* then returns true
if the signature of this ontology contains a class, object property, data property,
* named individual, annotation property or datatype with the specified IRI.
*/
boolean containsEntityInSignature(IRI entityIRI, boolean includeImportsClosure);
/**
* Determines if this ontology declares an entity i.e. it contains a declaration axiom for the specified entity.
*
* @param owlEntity The entity to be tested for
* @return true
if the ontology contains a declaration for the specified entity, otherwise
* false
.
*/
boolean isDeclared(OWLEntity owlEntity);
/**
* Determines if this ontology or its imports closure declares an entity i.e.
* contains a declaration axiom for the specified entity.
*
* @param owlEntity The entity to be tested for
* @param includeImportsClosure true
if the imports closure of this ontology should be examined,
* false
if just this ontology should be examined.
* @return true
if the ontology or its imports closure contains a declaration for the specified entity, otherwise
* false
.
*/
boolean isDeclared(OWLEntity owlEntity, boolean includeImportsClosure);
//////////////////////////////////////////////////////////////////////////////////////////////////
//
// Access by IRI
//
//////////////////////////////////////////////////////////////////////////////////////////////////
/**
* Determines if the signature of this ontology contains an OWLClass with the specified IRI.
*
* @param owlClassIRI The IRI of the OWLClass to check for.
* @return true
if the signature of this ontology contains an OWLClass that has owlClassIRI
as
* its IRI, otherwise false
.
*/
boolean containsClassInSignature(IRI owlClassIRI);
/**
* Determines if the signature of this ontology, or possibly the signature of one of the ontologies in the imports
* closure of this ontology, contains an OWLClass that has the specified IRI.
* @param owlClassIRI The IRI of the class to check for
* @param includeImportsClosure true
if the signature of the ontologies in the imports closure of this
* ontology should be checked, false
if just the signature of this ontology should be chekced.
* @return If includeImportsClosure=true
then returns true
if there is an OWLClass with
* owlClassIRI
as its IRI in the signature of at least one ontology in the imports clousre of this ontology
* and false
if this is not the case. If includeImportsClosure=false
then returns true
* if the signature of this ontology contains an OWLClass that has owlClassIRI
as its IRI and false
* if the signature of this ontology does not contain a class with owlClassIRI
as its IRI.
*/
boolean containsClassInSignature(IRI owlClassIRI, boolean includeImportsClosure);
/**
* Determines if the signature of this ontology contains an OWLObjectProperty with the specified IRI.
*
* @param owlObjectPropertyIRI The IRI of the OWLObjectProperty to check for.
* @return true
if the signature of this ontology contains an OWLObjectProperty that has owlObjectPropertyIRI
as
* its IRI, otherwise false
.
*/
boolean containsObjectPropertyInSignature(IRI owlObjectPropertyIRI);
/**
* Determines if the signature of this ontology, or possibly the signature of one of the ontologies in the imports
* closure of this ontology, contains an OWLObjectProperty that has the specified IRI.
* @param owlObjectPropertyIRI The IRI of the OWLObjectProperty to check for
* @param includeImportsClosure true
if the signature of the ontologies in the imports closure of this
* ontology should be checked, false
if just the signature of this ontology should be chekced.
* @return If includeImportsClosure=true
then returns true
if there is an OWLObjectProperty with
* owlObjectPropertyIRI
as its IRI in the signature of at least one ontology in the imports clousre of this ontology
* and false
if this is not the case. If includeImportsClosure=false
then returns true
* if the signature of this ontology contains an OWLObjectProperty that has owlObjectPropertyIRI
as its IRI and false
* if the signature of this ontology does not contain a class with owlObjectPropertyIRI
as its IRI.
*/
boolean containsObjectPropertyInSignature(IRI owlObjectPropertyIRI, boolean includeImportsClosure);
/**
* Determines if the signature of this ontology contains an OWLDataProperty with the specified IRI.
*
* @param owlDataPropertyIRI The IRI of the OWLDataProperty to check for.
* @return true
if the signature of this ontology contains an OWLDataProperty that has owlDataPropertyIRI
as
* its IRI, otherwise false
.
*/
boolean containsDataPropertyInSignature(IRI owlDataPropertyIRI);
/**
* Determines if the signature of this ontology, or possibly the signature of one of the ontologies in the imports
* closure of this ontology, contains an OWLDataProperty that has the specified IRI.
* @param owlDataPropertyIRI The IRI of the OWLDataProperty to check for
* @param includeImportsClosure true
if the signature of the ontologies in the imports closure of this
* ontology should be checked, false
if just the signature of this ontology should be chekced.
* @return If includeImportsClosure=true
then returns true
if there is an OWLDataProperty with
* owlDataPropertyIRI
as its IRI in the signature of at least one ontology in the imports clousre of this ontology
* and false
if this is not the case. If includeImportsClosure=false
then returns true
* if the signature of this ontology contains an OWLDataProperty that has owlDataPropertyIRI
as its IRI and false
* if the signature of this ontology does not contain a class with owlDataPropertyIRI
as its IRI.
*/
boolean containsDataPropertyInSignature(IRI owlDataPropertyIRI, boolean includeImportsClosure);
/**
* Determines if the signature of this ontology contains an OWLAnnotationProperty with the specified IRI.
*
* @param owlAnnotationPropertyIRI The IRI of the OWLAnnotationProperty to check for.
* @return true
if the signature of this ontology contains an OWLAnnotationProperty that has owlAnnotationPropertyIRI
as
* its IRI, otherwise false
.
*/
boolean containsAnnotationPropertyInSignature(IRI owlAnnotationPropertyIRI);
/**
* Determines if the signature of this ontology, or possibly the signature of one of the ontologies in the imports
* closure of this ontology, contains an OWLAnnotationProperty that has the specified IRI.
* @param owlAnnotationPropertyIRI The IRI of the OWLAnnotationProperty to check for
* @param includeImportsClosure true
if the signature of the ontologies in the imports closure of this
* ontology should be checked, false
if just the signature of this ontology should be chekced.
* @return If includeImportsClosure=true
then returns true
if there is an OWLAnnotationProperty with
* owlAnnotationPropertyIRI
as its IRI in the signature of at least one ontology in the imports clousre of this ontology
* and false
if this is not the case. If includeImportsClosure=false
then returns true
* if the signature of this ontology contains an OWLAnnotationProperty that has owlAnnotationPropertyIRI
as its IRI and false
* if the signature of this ontology does not contain a class with owlAnnotationPropertyIRI
as its IRI.
*/
boolean containsAnnotationPropertyInSignature(IRI owlAnnotationPropertyIRI, boolean includeImportsClosure);
/**
* Determines if the signature of this ontology contains an OWLNamedIndividual with the specified IRI.
*
* @param owlIndividualIRI The IRI of the OWLNamedIndividual to check for.
* @return true
if the signature of this ontology contains an OWLNamedIndividual that has owlIndividualIRI
as
* its IRI, otherwise false
.
*/
boolean containsIndividualInSignature(IRI owlIndividualIRI);
/**
* Determines if the signature of this ontology, or possibly the signature of one of the ontologies in the imports
* closure of this ontology, contains an OWLNamedIndividual that has the specified IRI.
* @param owlIndividualIRI The IRI of the OWLNamedIndividual to check for
* @param includeImportsClosure true
if the signature of the ontologies in the imports closure of this
* ontology should be checked, false
if just the signature of this ontology should be chekced.
* @return If includeImportsClosure=true
then returns true
if there is an OWLNamedIndividual with
* owlIndividualIRI
as its IRI in the signature of at least one ontology in the imports closure of this ontology
* and false
if this is not the case. If includeImportsClosure=false
then returns true
* if the signature of this ontology contains an OWLNamedIndividual that has owlIndividualIRI
as its IRI and false
* if the signature of this ontology does not contain a class with owlIndividualIRI
as its IRI.
*/
boolean containsIndividualInSignature(IRI owlIndividualIRI, boolean includeImportsClosure);
/**
* Determines if the signature of this ontology contains an OWLDatatype with the specified IRI.
*
* @param owlDatatypeIRI The IRI of the OWLDatatype to check for.
* @return true
if the signature of this ontology contains an OWLDatatype that has owlDatatypeIRI
as
* its IRI, otherwise false
.
*/
boolean containsDatatypeInSignature(IRI owlDatatypeIRI);
/**
* Determines if the signature of this ontology, or possibly the signature of one of the ontologies in the imports
* closure of this ontology, contains an OWLDatatype that has the specified IRI.
* @param owlDatatypeIRI The IRI of the OWLDatatype to check for
* @param includeImportsClosure true
if the signature of the ontologies in the imports closure of this
* ontology should be checked, false
if just the signature of this ontology should be chekced.
* @return If includeImportsClosure=true
then returns true
if there is an OWLDatatype with
* owlDatatypeIRI
as its IRI in the signature of at least one ontology in the imports closure of this ontology
* and false
if this is not the case. If includeImportsClosure=false
then returns true
* if the signature of this ontology contains an OWLDatatype that has owlDatatypeIRI
as its IRI and false
* if the signature of this ontology does not contain a class with owlDatatypeIRI
as its IRI.
*/
boolean containsDatatypeInSignature(IRI owlDatatypeIRI, boolean includeImportsClosure);
/**
* Gets the entities in the signature of this ontology that have the specified IRI.
*
* @param iri The IRI of the entities to be retrieved.
* @return A set of entities that are in the signature of this ontology that have the specified IRI. The
* set will be empty if there are no entities in the signature of this ontology with the specified IRI.
*/
Set getEntitiesInSignature(IRI iri);
/**
* Gets the entities in the signature of this ontology, and possibly the signature of the imports closure of this
* ontology, that have the specified IRI.
*
* @param iri The IRI of the entitied to be retrieved.
* @param includeImportsClosure Specifies if the signatures of the ontologies in the imports closure of this ontology
* should also be taken into account
* @return If includeImportsClosure=true
then returns a set of entities that are in the signature of this
* ontology or the signature of an ontology in the imports closure of this ontology that have iri
as their
* IRI. If includeImportsClosure=false
then returns the entities in the signature of just this ontology
* that have iri
as their IRI.
*/
Set getEntitiesInSignature(IRI iri, boolean includeImportsClosure);
//////////////////////////////////////////////////////////////////////////////////////////////
//
// Axioms that form part of a description of a named entity
//
//////////////////////////////////////////////////////////////////////////////////////////////
/**
* Gets the axioms that form the definition/description of a class.
*
* @param cls The class whose describing axioms are to be retrieved.
* @return A set of class axioms that describe the class. This set includes
* - Subclass axioms where the
* subclass is equal to the specified class
*
* - Equivalent class axioms where the specified class is an
* operand in the equivalent class axiom
- Disjoint class axioms where the specified class is an
* operand in the disjoint class axiom
- Disjoint union axioms, where the specified class is the
* named class that is equivalent to the disjoint union
The set that is returned is a copy - it
* will not be updated if the ontology changes. It is therefore safe to apply changes to this ontology
* while iterating over this set.
*/
Set getAxioms(OWLClass cls);
/**
* Gets the axioms that form the definition/description of an object property.
*
* @param prop The property whose defining axioms are to be retrieved.
* @return A set of object property axioms that includes - Sub-property axioms where the sub property is
* equal to the specified property
- Equivalent property axioms where the axiom contains the
* specified property
- Equivalent property axioms that contain the inverse of the specified
* property
- Disjoint property axioms that contain the specified property
- Domain axioms
* that specify a domain of the specified property
- Range axioms that specify a range of the
* specified property
- Any property characteristic axiom (i.e. Functional, Symmetric, Reflexive
* etc.) whose subject is the specified property
- Inverse properties axioms that contain the
* specified property
The set that is returned is a copy - it will not be updated if the ontology
* changes. It is therefore safe to apply changes to this ontology while iterating over this set.
*/
Set getAxioms(OWLObjectPropertyExpression prop);
/**
* Gets the axioms that form the definition/description of a data property.
*
* @param prop The property whose defining axioms are to be retrieved.
* @return A set of data property axioms that includes - Sub-property axioms where the sub property is equal
* to the specified property
- Equivalent property axioms where the axiom contains the specified
* property
- Disjoint property axioms that contain the specified property
- Domain axioms
* that specify a domain of the specified property
- Range axioms that specify a range of the
* specified property
- Any property characteristic axiom (i.e. Functional, Symmetric, Reflexive
* etc.) whose subject is the specified property
The set that is returned is a copy - it will not
* be updated if the ontology changes. It is therefore safe to apply changes to this ontology while
* iterating over this set.
*/
Set getAxioms(OWLDataProperty prop);
/**
* Gets the axioms that form the definition/description of an individual
*
* @param individual The individual whose defining axioms are to be retrieved.
* @return A set of individual axioms that includes - Individual type assertions that assert the type of the
* specified individual
- Same individuals axioms that contain the specified individual
* - Different individuals axioms that contain the specified individual
- Object property assertion
* axioms whose subject is the specified individual
- Data property assertion axioms whose subject is
* the specified individual
- Negative object property assertion axioms whose subject is the
* specified individual
- Negative data property assertion axioms whose subject is the specified
* individual
The set that is returned is a copy - it will not be updated if the ontology
* changes.
*/
Set getAxioms(OWLIndividual individual);
/**
* Gets the axioms that form the definition/description of an annotation property.
*
* @param property The property whose definition axioms are to be retrieved
* @return A set of axioms that includes - Annotation subpropertyOf axioms where the specified property is
* the sub property
- Annotation property domain axioms that specify a domain for the specified property
* - Annotation property range axioms that specify a range for the specified property
*/
Set getAxioms(OWLAnnotationProperty property);
/**
* Gets the datatype definition axioms for the specified datatype
*
* @param datatype The datatype
* @return The set of datatype definition axioms for the specified datatype
*/
Set getAxioms(OWLDatatype datatype);
//////////////////////////////////////////////////////////////////////////////////////////////
//
// Annotation axioms
//
//////////////////////////////////////////////////////////////////////////////////////////////
/**
* Gets the SubAnnotationPropertyOfAxiom
s where the specified property is the sub-property.
* @param subProperty The sub-property of the axioms to be retrieved.
* @return A set of OWLSubAnnotationPropertyOfAxiom
s such that the sub-property is equal
* to subProperty
.
*/
Set getSubAnnotationPropertyOfAxioms(OWLAnnotationProperty subProperty);
/**
* Gets the OWLAnnotationPropertyDomainAxiom
s where the specified property is the property
* in the domain axiom.
* @param property The property that the axiom specifies a domain for.
* @return A set of OWLAnnotationPropertyDomainAxiom
s such that the property is equal
* to property
.
*/
Set getAnnotationPropertyDomainAxioms(OWLAnnotationProperty property);
/**
* Gets the OWLAnnotationPropertyRangeAxiom
s where the specified property is the property
* in the range axiom.
* @param property The property that the axiom specifies a range for.
* @return A set of OWLAnnotationPropertyRangeAxiom
s such that the property is equal
* to property
.
*/
Set getAnnotationPropertyRangeAxioms(OWLAnnotationProperty property);
//////////////////////////////////////////////////////////////////////////////////////////////
//
// Various methods that provide axioms relating to specific entities that allow
// frame style views to be composed for a particular entity. Such functionality is
// useful for ontology editors and browsers.
//
//////////////////////////////////////////////////////////////////////////////////////////////
/**
* Gets the declaration axioms for specified entity.
*
* @param subject The entity that is the subject of the set of returned axioms.
* @return The set of declaration axioms. Note that this set will be a copy and will not be updated if the ontology
* changes. It is therefore safe to iterate over this set while making changes to the ontology.
*/
Set getDeclarationAxioms(OWLEntity subject);
/**
* Gets the axioms that annotate the specified entity.
*
* @param entity The entity whose annotations are to be retrieved.
* @return The set of entity annotation axioms. Note that this set will be a copy and will not be updated if the
* ontology changes. It is therefore safe to iterate over this set while making changes to the ontology.
*/
Set getAnnotationAssertionAxioms(OWLAnnotationSubject entity);
//////////////////////////////////////////////////////////////////////////////////////////////
//
// Classes
//
//////////////////////////////////////////////////////////////////////////////////////////////
/**
* Gets all of the subclass axioms where the left hand side (the subclass) is equal to the specified class.
*
* @param cls The class that is equal to the left hand side of the axiom (subclass).
* @return The set that is returned is a copy - it will not be updated if the ontology changes. It is therefore
* safe to apply changes to this ontology while iterating over this set.
*/
Set getSubClassAxiomsForSubClass(OWLClass cls);
/**
* Gets all of the subclass axioms where the right hand side (the superclass) is equal to the specified class.
*
* @param cls The class
* @return The set that is returned is a copy - it will not be updated if the ontology changes. It is therefore
* safe to apply changes to this ontology while iterating over this set.
*/
Set getSubClassAxiomsForSuperClass(OWLClass cls);
/**
* Gets all of the equivalent axioms in this ontology that contain the specified class as an operand.
*
* @param cls The class
* @return A set of equivalent class axioms that contain the specified class as an operand. The set that is
* returned is a copy - it will not be updated if the ontology changes. It is therefore safe to apply
* changes to this ontology while iterating over this set.
*/
Set getEquivalentClassesAxioms(OWLClass cls);
/**
* Gets the set of disjoint class axioms that contain the specified class as an operand.
*
* @param cls The class that should be contained in the set of disjoint class axioms that will be returned.
* @return The set of disjoint axioms that contain the specified class. The set that is returned is a copy - it
* will not be updated if the ontology changes. It is therefore safe to apply changes to this ontology
* while iterating over this set.
*/
Set getDisjointClassesAxioms(OWLClass cls);
/**
* Gets the set of disjoint union axioms that have the specified class as the named class that is equivalent to the
* disjoint union of operands. For example, if the ontology contained the axiom DisjointUnion(A, propP some C, D,
* E) this axiom would be returned for class A (but not for D or E).
*
* @param owlClass The class that indexes the axioms to be retrieved.
* @return The set that is returned is a copy - it will not be updated if the ontology changes. It is therefore
* safe to apply changes to this ontology while iterating over this set.
*/
Set getDisjointUnionAxioms(OWLClass owlClass);
/**
* Gets the has key axioms that have the specified class as their subject.
*
* @param cls The subject of the has key axioms
* @return The set of has key axioms that have cls as their subject. The set that is returned is a copy -
* it will not be updated if the ontology changes. It is therefore safe to apply changes to this ontology
* while iterating over this set.
*/
Set getHasKeyAxioms(OWLClass cls);
//////////////////////////////////////////////////////////////////////////////////////////////
//
// Object properties
//
//////////////////////////////////////////////////////////////////////////////////////////////
/**
* Gets the {@link org.semanticweb.owlapi.model.OWLSubObjectPropertyOfAxiom}s where the sub-property is equal to the specified property.
*
* @param subProperty The property which is equal to the sub property of the retrived axioms.
* @return A set of {@link org.semanticweb.owlapi.model.OWLSubObjectPropertyOfAxiom}s such that each axiom in the set is of the form
* SubObjectPropertyOf(subProperty, pe)
.
* The set that is returned is a copy - it will not be updated if the ontology changes. It is therefore
* safe to apply changes to this ontology while iterating over this set.
*/
Set getObjectSubPropertyAxiomsForSubProperty(OWLObjectPropertyExpression subProperty);
/**
* Gets the {@link org.semanticweb.owlapi.model.OWLSubObjectPropertyOfAxiom}s where the super-property (returned by
* {@link OWLSubObjectPropertyOfAxiom#getSuperProperty()}) is equal to the specified property.
*
* @param superProperty The property which is equal to the super-property of the retrived axioms.
* @return A set of {@link org.semanticweb.owlapi.model.OWLSubObjectPropertyOfAxiom}s such that each axiom in the set is of the form
* SubObjectPropertyOf(pe, superProperty)
.
* The set that is returned is a copy - it will not be updated if the ontology changes. It is therefore
* safe to apply changes to this ontology while iterating over this set.
*/
Set getObjectSubPropertyAxiomsForSuperProperty(OWLObjectPropertyExpression superProperty);
/**
* Gets the {@link org.semanticweb.owlapi.model.OWLObjectPropertyDomainAxiom}s where the property (returned by
* {@link org.semanticweb.owlapi.model.OWLObjectPropertyRangeAxiom#getProperty()}) is equal to the specified property.
*
* @param property The property which is equal to the property of the retrived axioms.
* @return A set of {@link org.semanticweb.owlapi.model.OWLObjectPropertyDomainAxiom}s such that each axiom in the
* set is of the form ObjectPropertyDomain(pe, ce)
. The set that is returned is a copy - it will not
* be updated if the ontology changes. It is therefore safe to apply changes to this ontology while iterating over
* this set.
*/
Set getObjectPropertyDomainAxioms(OWLObjectPropertyExpression property);
/**
* Gets the {@link org.semanticweb.owlapi.model.OWLObjectPropertyRangeAxiom}s where the property (returned by
* {@link org.semanticweb.owlapi.model.OWLObjectPropertyRangeAxiom#getProperty()}) is equal to the
* specified property.
* @param property The property which is equal to the property of the retieved axioms.
* @return A set of {@link org.semanticweb.owlapi.model.OWLObjectPropertyRangeAxiom}s such that each axiom in the
* set is of the form ObjectPropertyRange(property, ce)
. The set that is returned is a copy - it will not
* be updated if the ontology changes. It is therefore safe to apply changes to this ontology while iterating over
* this set.
*/
Set getObjectPropertyRangeAxioms(OWLObjectPropertyExpression property);
/**
* Gets the {@link OWLInverseObjectPropertiesAxiom}s where the specified property
* is contained in the set returned by {@link org.semanticweb.owlapi.model.OWLInverseObjectPropertiesAxiom#getProperties()}.
* @param property The property which is equal to the property of the retrieved axioms.
* @return A set of {@link OWLInverseObjectPropertiesAxiom}s such that each axiom in the
* set is of the form InverseObjectProperties(property, pe)
or InverseObjectProperties(pe, property)
.
* The set that is returned is a copy - it will not be updated if the ontology changes. It is therefore safe to
* apply changes to this ontology while iterating over this set.
*/
Set getInverseObjectPropertyAxioms(OWLObjectPropertyExpression property);
/**
* Gets the {@link org.semanticweb.owlapi.model.OWLEquivalentObjectPropertiesAxiom}s that make the specified property
* equivalent to some other object property expression(s).
* @param property The property that the retrieved axioms make equivalent to some other property expressions. For each
* axiom retrieved the set of properties returned by {@link OWLEquivalentObjectPropertiesAxiom#getProperties()} will
* contain property.
* @return A set of {@link org.semanticweb.owlapi.model.OWLEquivalentObjectPropertiesAxiom}s such that each axiom
* in the set is of the form EquivalentObjectProperties(pe0, ..., property, ..., pen)
. The set that is
* returned is a copy - it will not be updated if the ontology changes. It is therefore safe to
* apply changes to this ontology while iterating over this set.
*/
Set getEquivalentObjectPropertiesAxioms(OWLObjectPropertyExpression property);
/**
* Gets the {@link org.semanticweb.owlapi.model.OWLDisjointObjectPropertiesAxiom}s that make the specified property
* disjoint with some other object property expression(s).
* @param property The property that the retrieved axioms makes disjoint to some other property expressions. For each
* axiom retrieved the set of properties returned by {@link OWLDisjointObjectPropertiesAxiom#getProperties()} will
* contain property.
* @return A set of {@link org.semanticweb.owlapi.model.OWLDisjointObjectPropertiesAxiom}s such that each axiom
* in the set is of the form DisjointObjectProperties(pe0, ..., property, ..., pen)
. The set that is
* returned is a copy - it will not be updated if the ontology changes. It is therefore safe to
* apply changes to this ontology while iterating over this set.
*/
Set getDisjointObjectPropertiesAxioms(OWLObjectPropertyExpression property);
/**
* Gets the {@link org.semanticweb.owlapi.model.OWLFunctionalObjectPropertyAxiom}s contained in this ontology that
* make the specified object property functional.
* @param property The property (returned by {@link org.semanticweb.owlapi.model.OWLFunctionalObjectPropertyAxiom#getProperty()})
* that is made functional by the axioms.
* @return A set of {@link org.semanticweb.owlapi.model.OWLFunctionalObjectPropertyAxiom}s such that each axiom in the
* set is of the form FunctionalObjectProperty(property)
.
*/
Set getFunctionalObjectPropertyAxioms(OWLObjectPropertyExpression property);
/**
* Gets the {@link OWLInverseFunctionalObjectPropertyAxiom}s contained in this ontology that
* make the specified object property inverse functional.
* @param property The property (returned by {@link org.semanticweb.owlapi.model.OWLInverseFunctionalObjectPropertyAxiom#getProperty()})
* that is made inverse functional by the axioms.
* @return A set of {@link org.semanticweb.owlapi.model.OWLFunctionalObjectPropertyAxiom}s such that each axiom in the
* set is of the form InverseFunctionalObjectProperty(property)
.
*/
Set getInverseFunctionalObjectPropertyAxioms(OWLObjectPropertyExpression property);
/**
* Gets the {@link org.semanticweb.owlapi.model.OWLSymmetricObjectPropertyAxiom}s contained in this ontology that
* make the specified object property symmetric.
* @param property The property (returned by {@link org.semanticweb.owlapi.model.OWLSymmetricObjectPropertyAxiom#getProperty()})
* that is made symmetric by the axioms.
* @return A set of {@link org.semanticweb.owlapi.model.OWLSymmetricObjectPropertyAxiom}s such that each axiom in the
* set is of the form SymmetricObjectProperty(property)
.
*/
Set getSymmetricObjectPropertyAxioms(OWLObjectPropertyExpression property);
/**
* Gets the {@link org.semanticweb.owlapi.model.OWLAsymmetricObjectPropertyAxiom}s contained in this ontology that
* make the specified object property asymmetric.
* @param property The property (returned by {@link org.semanticweb.owlapi.model.OWLAsymmetricObjectPropertyAxiom#getProperty()})
* that is made asymmetric by the axioms.
* @return A set of {@link org.semanticweb.owlapi.model.OWLAsymmetricObjectPropertyAxiom}s such that each axiom in the
* set is of the form AsymmetricObjectProperty(property)
.
*/
Set getAsymmetricObjectPropertyAxioms(OWLObjectPropertyExpression property);
/**
* Gets the {@link org.semanticweb.owlapi.model.OWLReflexiveObjectPropertyAxiom}s contained in this ontology that
* make the specified object property reflexive.
* @param property The property (returned by {@link org.semanticweb.owlapi.model.OWLReflexiveObjectPropertyAxiom#getProperty()})
* that is made reflexive by the axioms.
* @return A set of {@link org.semanticweb.owlapi.model.OWLReflexiveObjectPropertyAxiom}s such that each axiom in the
* set is of the form ReflexiveObjectProperty(property)
.
*/
Set getReflexiveObjectPropertyAxioms(OWLObjectPropertyExpression property);
/**
* Gets the {@link org.semanticweb.owlapi.model.OWLIrreflexiveObjectPropertyAxiom}s contained in this ontology that
* make the specified object property irreflexive.
* @param property The property (returned by {@link org.semanticweb.owlapi.model.OWLIrreflexiveObjectPropertyAxiom#getProperty()})
* that is made irreflexive by the axioms.
* @return A set of {@link org.semanticweb.owlapi.model.OWLIrreflexiveObjectPropertyAxiom}s such that each axiom in the
* set is of the form IrreflexiveObjectProperty(property)
.
*/
Set getIrreflexiveObjectPropertyAxioms(OWLObjectPropertyExpression property);
/**
* Gets the {@link org.semanticweb.owlapi.model.OWLTransitiveObjectPropertyAxiom}s contained in this ontology that
* make the specified object property transitive.
* @param property The property (returned by {@link org.semanticweb.owlapi.model.OWLTransitiveObjectPropertyAxiom#getProperty()})
* that is made transitive by the axioms.
* @return A set of {@link org.semanticweb.owlapi.model.OWLTransitiveObjectPropertyAxiom}s such that each axiom in the
* set is of the form TransitiveObjectProperty(property)
.
*/
Set getTransitiveObjectPropertyAxioms(OWLObjectPropertyExpression property);
//////////////////////////////////////////////////////////////////////////////////////////////
//
// Data properties
//
//////////////////////////////////////////////////////////////////////////////////////////////
/**
* Gets the {@link org.semanticweb.owlapi.model.OWLSubDataPropertyOfAxiom}s where the sub-property is equal to the specified property.
*
* @param subProperty The property which is equal to the sub property of the retrived axioms.
* @return A set of {@link org.semanticweb.owlapi.model.OWLSubDataPropertyOfAxiom}s such that each axiom in the set is of the form
* SubDataPropertyOf(subProperty, pe)
.
* The set that is returned is a copy - it will not be updated if the ontology changes. It is therefore
* safe to apply changes to this ontology while iterating over this set.
*/
Set getDataSubPropertyAxiomsForSubProperty(OWLDataProperty subProperty);
/**
* Gets the {@link org.semanticweb.owlapi.model.OWLSubDataPropertyOfAxiom}s where the super-property (returned by
* {@link OWLSubDataPropertyOfAxiom#getSuperProperty()}) is equal to the specified property.
*
* @param superProperty The property which is equal to the super-property of the retrived axioms.
* @return A set of {@link org.semanticweb.owlapi.model.OWLSubDataPropertyOfAxiom}s such that each axiom in the set is of the form
* SubDataPropertyOf(pe, superProperty)
.
* The set that is returned is a copy - it will not be updated if the ontology changes. It is therefore
* safe to apply changes to this ontology while iterating over this set.
*/
Set getDataSubPropertyAxiomsForSuperProperty(OWLDataPropertyExpression superProperty);
/**
* Gets the {@link org.semanticweb.owlapi.model.OWLDataPropertyDomainAxiom}s where the property (returned by
* {@link org.semanticweb.owlapi.model.OWLDataPropertyRangeAxiom#getProperty()}) is equal to the specified property.
*
* @param property The property which is equal to the property of the retrived axioms.
* @return A set of {@link org.semanticweb.owlapi.model.OWLDataPropertyDomainAxiom}s such that each axiom in the
* set is of the form DataPropertyDomain(pe, ce)
. The set that is returned is a copy - it will not
* be updated if the ontology changes. It is therefore safe to apply changes to this ontology while iterating over
* this set.
*/
Set getDataPropertyDomainAxioms(OWLDataProperty property);
/**
* Gets the {@link org.semanticweb.owlapi.model.OWLDataPropertyRangeAxiom}s where the property (returned by
* {@link org.semanticweb.owlapi.model.OWLDataPropertyRangeAxiom#getProperty()}) is equal to the
* specified property.
* @param property The property which is equal to the property of the retieved axioms.
* @return A set of {@link org.semanticweb.owlapi.model.OWLDataPropertyRangeAxiom}s such that each axiom in the
* set is of the form DataPropertyRange(property, ce)
. The set that is returned is a copy - it will not
* be updated if the ontology changes. It is therefore safe to apply changes to this ontology while iterating over
* this set.
*/
Set getDataPropertyRangeAxioms(OWLDataProperty property);
/**
* Gets the {@link org.semanticweb.owlapi.model.OWLEquivalentDataPropertiesAxiom}s that make the specified property
* equivalent to some other data property expression(s).
* @param property The property that the retrieved axioms make equivalent to some other property expressions. For each
* axiom retrieved the set of properties returned by {@link OWLEquivalentDataPropertiesAxiom#getProperties()} will
* contain property.
* @return A set of {@link org.semanticweb.owlapi.model.OWLEquivalentDataPropertiesAxiom}s such that each axiom
* in the set is of the form EquivalentDataProperties(pe0, ..., property, ..., pen)
. The set that is
* returned is a copy - it will not be updated if the ontology changes. It is therefore safe to
* apply changes to this ontology while iterating over this set.
*/
Set getEquivalentDataPropertiesAxioms(OWLDataProperty property);
/**
* Gets the {@link org.semanticweb.owlapi.model.OWLDisjointDataPropertiesAxiom}s that make the specified property
* disjoint with some other data property expression(s).
* @param property The property that the retrieved axioms makes disjoint to some other property expressions. For each
* axiom retrieved the set of properties returned by {@link OWLDisjointDataPropertiesAxiom#getProperties()} will
* contain property.
* @return A set of {@link org.semanticweb.owlapi.model.OWLDisjointDataPropertiesAxiom}s such that each axiom
* in the set is of the form DisjointDataProperties(pe0, ..., property, ..., pen)
. The set that is
* returned is a copy - it will not be updated if the ontology changes. It is therefore safe to
* apply changes to this ontology while iterating over this set.
*/
Set getDisjointDataPropertiesAxioms(OWLDataProperty property);
/**
* Gets the {@link org.semanticweb.owlapi.model.OWLFunctionalDataPropertyAxiom}s contained in this ontology that
* make the specified data property functional.
* @param property The property (returned by {@link org.semanticweb.owlapi.model.OWLFunctionalDataPropertyAxiom#getProperty()})
* that is made functional by the axioms.
* @return A set of {@link org.semanticweb.owlapi.model.OWLFunctionalDataPropertyAxiom}s such that each axiom in the
* set is of the form FunctionalDataProperty(property)
. The set that is
* returned is a copy - it will not be updated if the ontology changes. It is therefore safe to
* apply changes to this ontology while iterating over this set.
*/
Set getFunctionalDataPropertyAxioms(OWLDataPropertyExpression property);
//////////////////////////////////////////////////////////////////////////////////////////////
//
// Individuals
//
//////////////////////////////////////////////////////////////////////////////////////////////
/**
* Gets the {@link org.semanticweb.owlapi.model.OWLClassAssertionAxiom}s contained in this ontology that
* make the specified individual
an instance of some class expression.
* @param individual The individual that the returned axioms make an instance of some class expression.
* @return A set of {@link org.semanticweb.owlapi.model.OWLClassAssertionAxiom}s such that each axiom in the set
* is of the form ClassAssertion(ce, individual)
(for each axiom {@link OWLClassAssertionAxiom#getIndividual()} is
* equal to individual
). The set that is
* returned is a copy - it will not be updated if the ontology changes. It is therefore safe to
* apply changes to this ontology while iterating over this set.
*/
Set getClassAssertionAxioms(OWLIndividual individual);
/**
* Gets the {@link org.semanticweb.owlapi.model.OWLClassAssertionAxiom}s contained in this ontology that make the
* specified class expression, ce
, a type for some individual.
* @param ce The class expression that the returned axioms make a type for some individual.
* @return A set of {@link org.semanticweb.owlapi.model.OWLClassAssertionAxiom}s such that each axiom in the set
* is of the form ClassAssertion(ce, ind)
(for each axiom {@link OWLClassAssertionAxiom#getClassExpression()} is
* equal to ce
). The set that is
* returned is a copy - it will not be updated if the ontology changes. It is therefore safe to
* apply changes to this ontology while iterating over this set.
*/
Set getClassAssertionAxioms(OWLClassExpression ce);
/**
* Gets the {@link org.semanticweb.owlapi.model.OWLDataPropertyAssertionAxiom}s contained in this ontology that
* have the specified individual
as the subject of the axiom.
* @param individual The individual that the returned axioms have as a subject.
* @return A set of {@link org.semanticweb.owlapi.model.OWLDataPropertyAssertionAxiom}s such that each axiom in
* the set is of the form DataPropertyAssertion(dp, individual, l)
(for each axiom {@link OWLDataPropertyAssertionAxiom#getSubject()} is
* equal to individual
). The set that is
* returned is a copy - it will not be updated if the ontology changes. It is therefore safe to
* apply changes to this ontology while iterating over this set.
*/
Set getDataPropertyAssertionAxioms(OWLIndividual individual);
/**
* Gets the {@link org.semanticweb.owlapi.model.OWLObjectPropertyAssertionAxiom}s contained in this ontology that
* have the specified individual
as the subject of the axiom.
* @param individual The individual that the returned axioms have as a subject.
* @return A set of {@link org.semanticweb.owlapi.model.OWLObjectPropertyAssertionAxiom}s such that each axiom in
* the set is of the form ObjectPropertyAssertion(dp, individual, obj)
(for each axiom {@link OWLObjectPropertyAssertionAxiom#getSubject()} is
* equal to individual
). The set that is
* returned is a copy - it will not be updated if the ontology changes. It is therefore safe to
* apply changes to this ontology while iterating over this set.
*/
Set getObjectPropertyAssertionAxioms(OWLIndividual individual);
/**
* Gets the {@link org.semanticweb.owlapi.model.OWLNegativeObjectPropertyAssertionAxiom}s contained in this ontology that
* have the specified individual
as the subject of the axiom.
* @param individual The individual that the returned axioms have as a subject.
* @return A set of {@link org.semanticweb.owlapi.model.OWLNegativeObjectPropertyAssertionAxiom}s such that each axiom in
* the set is of the form NegativeObjectPropertyAssertion(dp, individual, obj)
(for each axiom {@link OWLNegativeObjectPropertyAssertionAxiom#getSubject()} is
* equal to individual
). The set that is
* returned is a copy - it will not be updated if the ontology changes. It is therefore safe to
* apply changes to this ontology while iterating over this set.
*/
Set getNegativeObjectPropertyAssertionAxioms(OWLIndividual individual);
/**
* Gets the {@link org.semanticweb.owlapi.model.OWLNegativeDataPropertyAssertionAxiom}s contained in this ontology that
* have the specified individual
as the subject of the axiom.
* @param individual The individual that the returned axioms have as a subject.
* @return A set of {@link org.semanticweb.owlapi.model.OWLNegativeDataPropertyAssertionAxiom}s such that each axiom in
* the set is of the form NegativeDataPropertyAssertion(dp, individual, obj)
(for each axiom {@link OWLNegativeDataPropertyAssertionAxiom#getSubject()} is
* equal to individual
). The set that is
* returned is a copy - it will not be updated if the ontology changes. It is therefore safe to
* apply changes to this ontology while iterating over this set.
*/
Set getNegativeDataPropertyAssertionAxioms(OWLIndividual individual);
/**
* Gets the {@link org.semanticweb.owlapi.model.OWLSameIndividualAxiom}s contained in this ontology that make
* the specified individual
the same as some other individual.
* @param individual The individual that the returned axioms make the same as some other individual.
* @return A set of {@link org.semanticweb.owlapi.model.OWLSameIndividualAxiom}s such that each axiom in the set
* is of the form SameIndividual(individual, ind, ...)
(for each axiom returned {@link OWLSameIndividualAxiom#getIndividuals()}
* contains individual
. The set that is
* returned is a copy - it will not be updated if the ontology changes. It is therefore safe to
* apply changes to this ontology while iterating over this set.
*/
Set getSameIndividualAxioms(OWLIndividual individual);
/**
* Gets the {@link org.semanticweb.owlapi.model.OWLDifferentIndividualsAxiom}s contained in this ontology that make
* the specified individual
different to some other individual.
* @param individual The individual that the returned axioms make the different as some other individual.
* @return A set of {@link org.semanticweb.owlapi.model.OWLDifferentIndividualsAxiom}s such that each axiom in the set
* is of the form DifferentIndividuals(individual, ind, ...)
(for each axiom returned {@link OWLDifferentIndividualsAxiom#getIndividuals()}
* contains individual
. The set that is
* returned is a copy - it will not be updated if the ontology changes. It is therefore safe to
* apply changes to this ontology while iterating over this set.
*/
Set getDifferentIndividualAxioms(OWLIndividual individual);
/**
* Gets the {@link org.semanticweb.owlapi.model.OWLDatatypeDefinitionAxiom}s contained in this ontology that provide
* a definition for the specified datatype.
* @param datatype The datatype for which the returned axioms provide a definition.
* @return A set of {@link org.semanticweb.owlapi.model.OWLDatatypeDefinitionAxiom}s such that for each axiom in the
* set {@link OWLDatatypeDefinitionAxiom#getDatatype()} is equal to datatype
. The set that is
* returned is a copy - it will not be updated if the ontology changes. It is therefore safe to
* apply changes to this ontology while iterating over this set.
*/
Set getDatatypeDefinitions(OWLDatatype datatype);
// XXX when the interface changes, uncomment this
// /** @param entity
// * entyty to check
// * @return true if entity is referenced */
// boolean containsReference(OWLClass entity);
//
// /** @param entity
// * entyty to check
// * @return true if entity is referenced */
// boolean containsReference(OWLObjectProperty entity);
//
// /** @param entity
// * entyty to check
// * @return true if entity is referenced */
// boolean containsReference(OWLDataProperty entity);
//
// /** @param entity
// * entyty to check
// * @return true if entity is referenced */
// boolean containsReference(OWLNamedIndividual entity);
//
// /** @param entity
// * entyty to check
// * @return true if entity is referenced */
// boolean containsReference(OWLDatatype entity);
//
// /** @param entity
// * entyty to check
// * @return true if entity is referenced */
// boolean containsReference(OWLAnnotationProperty propentityerty);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy