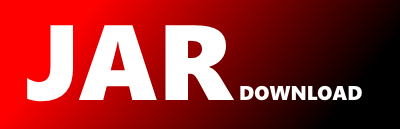
com.clarkparsia.pellet.sparqldl.model.CoreImpl Maven / Gradle / Ivy
// Copyright (c) 2006 - 2008, Clark & Parsia, LLC.
// This source code is available under the terms of the Affero General Public
// License v3.
//
// Please see LICENSE.txt for full license terms, including the availability of
// proprietary exceptions.
// Questions, comments, or requests for clarification: [email protected]
package com.clarkparsia.pellet.sparqldl.model;
import java.util.ArrayList;
import java.util.Collection;
import java.util.List;
import org.mindswap.pellet.utils.ATermUtils;
import aterm.ATermAppl;
/**
*
* Title: Implementation of the Core of undistinguished variables.
*
*
* Description:
*
*
* Copyright: Copyright (c) 2007
*
*
* Company: Clark & Parsia, LLC.
*
*
* @author Petr Kremen
*/
public class CoreImpl extends QueryAtomImpl implements Core {
private List distVars = null;
private List consts = null;
private Collection undistVars = null;
private Collection atoms;
public CoreImpl(final List arguments, final Collection uv,
final Collection atoms) {
super( QueryPredicate.UndistVarCore, arguments );
this.atoms = atoms;
this.undistVars = uv;
}
private void setup() {
distVars = new ArrayList();
consts = new ArrayList();
for( final ATermAppl a : arguments ) {
if( ATermUtils.isVar( a ) ) {
distVars.add( a );
}
else {
consts.add( a );
}
}
}
/*
* (non-Javadoc)
* @see org.mindswap.pellet.sparqldl.model.CoreIF#getConstants()
*/
public Collection getConstants() {
if( consts == null ) {
setup();
}
return consts;
}
/*
* (non-Javadoc)
* @see org.mindswap.pellet.sparqldl.model.CoreIF#getDistVars()
*/
public Collection getDistVars() {
if( distVars == null ) {
setup();
}
return distVars;
}
/*
* (non-Javadoc)
* @see org.mindswap.pellet.sparqldl.model.CoreIF#getUndistVars()
*/
public Collection getUndistVars() {
if( undistVars == null ) {
setup();
}
return undistVars;
}
/*
* (non-Javadoc)
* @see
* org.mindswap.pellet.sparqldl.model.CoreIF#apply(org.mindswap.pellet.sparqldl
* .model.ResultBinding)
*/
public QueryAtom apply(final ResultBinding binding) {
if( isGround() ) {
return this;
}
final List newArguments = new ArrayList();
for( final ATermAppl a : arguments ) {
if( binding.isBound( a ) ) {
newArguments.add( binding.getValue( a ) );
}
else {
newArguments.add( a );
}
}
final List newAtoms = new ArrayList();
for( final QueryAtom a : atoms ) {
newAtoms.add( a.apply( binding ) );
}
return new CoreImpl( newArguments, undistVars, newAtoms );
}
@Override
public int hashCode() {
return arguments.hashCode();
}
@Override
public boolean equals(Object obj) {
if( this == obj )
return true;
if( obj == null )
return false;
if( getClass() != obj.getClass() )
return false;
final CoreImpl other = (CoreImpl) obj;
return arguments.equals( other.arguments );
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy