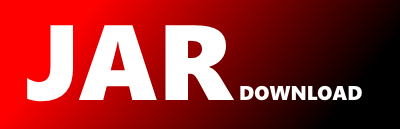
com.github.antelopeframework.launcher.SpringBootstrap Maven / Gradle / Ivy
package com.github.antelopeframework.launcher;
import lombok.extern.slf4j.Slf4j;
import org.apache.commons.lang3.StringUtils;
import org.apache.commons.lang3.builder.ToStringBuilder;
import com.github.antelopeframework.spring.ApplicationContextContainer;
import com.github.antelopeframework.util.component.AbstractLifeCycle;
@Slf4j
public class SpringBootstrap extends AbstractLifeCycle {
public final static String CONFIG_LOCATION_KEY = "spring-config-locations";
private String[] configLocations;
private ApplicationContextContainer springContainer;
private SpringBootstrap(String[] configLocations) {
if (configLocations == null) {
String locations = System.getProperty(CONFIG_LOCATION_KEY);
if (StringUtils.isEmpty(locations)) {
throw new IllegalArgumentException("请配置 spring-config-locations 参数.");
}
this.configLocations = locations.split(",");
return;
}
this.configLocations = configLocations;
}
@Override
public void doStart() throws Exception {
springContainer = new ApplicationContextContainer(configLocations);
springContainer.start();
}
@Override
public void doStop() throws Exception {
try {
if (springContainer != null) {
springContainer.stop();
springContainer = null;
}
} catch (Throwable e) {
log.error(e.getMessage(), e);
}
}
public static void main(String[] args) {
final SpringBootstrap server = new SpringBootstrap(null);
try {
Runtime.getRuntime().addShutdownHook(new Thread() {
public void run() {
try {
server.stop();
log.info("Server stopped!");
} catch (Throwable t) {
log.error(t.getMessage(), t);
}
synchronized (SpringBootstrap.class) {
SpringBootstrap.class.notify();
}
}
});
server.start();
} catch (Exception e) {
e.printStackTrace();
log.error(e.getMessage(), e);
System.exit(1);
}
synchronized (SpringBootstrap.class) {
while (server.isRunning()) {
try {
SpringBootstrap.class.wait();
} catch (Throwable e) {
e.printStackTrace();
}
}
}
}
@Override
public String toString() {
return new ToStringBuilder(this)
.append(configLocations)
.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy