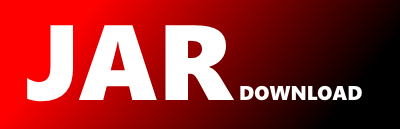
com.github.antelopeframework.spring.SpringContextHolder Maven / Gradle / Ivy
The newest version!
package com.github.antelopeframework.spring;
import java.util.Locale;
import java.util.Map;
import org.springframework.beans.BeansException;
import org.springframework.beans.factory.NoSuchBeanDefinitionException;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.beans.factory.config.AutowireCapableBeanFactory;
import org.springframework.context.ApplicationContext;
import org.springframework.context.ApplicationContextAware;
/**
* 以静态变量保存Spring ApplicationContext, 可在任何代码任何地方任何时候中取出ApplicaitonContext.
*
* @author yangzhi
*/
public class SpringContextHolder implements ApplicationContextAware {
static ApplicationContext applicationContext;
/**
* 实现ApplicationContextAware接口的context注入函数, 将其存入静态变量.
*/
@Autowired
public void setApplicationContext(ApplicationContext applicationContext) {
SpringContextHolder.applicationContext = applicationContext; // NOSONAR
}
/**
* 取得存储在静态变量中的ApplicationContext.
*/
public static ApplicationContext getApplicationContext() {
checkApplicationContext();
return applicationContext;
}
/**
* http://stackoverflow.com/questions/310271/injecting-beans-into-a-class-
* outside-the-spring-managed-context
*
* @param existingBean
* @param autowireMode
* @param dependencyCheck
*/
public void autowireBeanProperties(Object existingBean, int autowireMode,
boolean dependencyCheck) {
getApplicationContext().getAutowireCapableBeanFactory()
.autowireBeanProperties(existingBean, autowireMode,
dependencyCheck);
}
/**
* AutowireCapableBeanFactory.AUTOWIRE_BY_TYPE
*
* @param existingBean
*/
public static void autowireBeanProperties(Object existingBean) {
getApplicationContext().getAutowireCapableBeanFactory()
.autowireBeanProperties(existingBean,
AutowireCapableBeanFactory.AUTOWIRE_BY_TYPE, true);
}
/**
* 从静态变量ApplicationContext中取得Bean, 自动转型为所赋值对象的类型.
*/
@SuppressWarnings("unchecked")
public static T getBean(String name) {
checkApplicationContext();
return (T) applicationContext.getBean(name);
}
/**
* 从静态变量ApplicationContext中取得Bean, 自动转型为所赋值对象的类型. 如果有多个Bean符合Class, 取出第一个.
*/
public static T getBean(Class requiredType) {
checkApplicationContext();
return applicationContext.getBean(requiredType);
}
/**
* 获取类型为requiredType的对象
* 如果bean不能被类型转换,相应的异常将会被抛出(BeanNotOfRequiredTypeException)
*
* @param name bean注册名
* @param requiredType 返回对象类型
* @return Object 返回requiredType类型对象
* @throws BeansException
*/
public static T getBean(String name, Class requiredType) throws BeansException {
return applicationContext.getBean(name, requiredType);
}
/**
*
* @param requiredType
* @return
*/
public static Map getBeans(Class requiredType) {
return applicationContext.getBeansOfType(requiredType);
}
/**
* 如果BeanFactory包含一个与所给名称匹配的bean定义,则返回true
*
* @param name
* @return boolean
*/
public static boolean containsBean(String name) {
return applicationContext.containsBean(name);
}
/**
* 判断以给定名字注册的bean定义是一个singleton还是一个prototype。
* 如果与给定名字相应的bean定义没有被找到,将会抛出一个异常(NoSuchBeanDefinitionException)
*
* @param name
* @return boolean
* @throws NoSuchBeanDefinitionException
*/
public static boolean isSingleton(String name) throws NoSuchBeanDefinitionException {
return applicationContext.isSingleton(name);
}
/**
* @param name
* @return Class 注册对象的类型
* @throws NoSuchBeanDefinitionException
*/
public static Class> getType(String name) throws NoSuchBeanDefinitionException {
return applicationContext.getType(name);
}
/**
* 如果给定的bean名字在bean定义中有别名,则返回这些别名
*
* @param name
* @return
* @throws NoSuchBeanDefinitionException
*/
public static String[] getAliases(String name) throws NoSuchBeanDefinitionException {
return applicationContext.getAliases(name);
}
/**
* spring 国际化,获取 message
*
* @param code message key
* @param params 替换message中的{0},{1}
* @return
*/
public static String getMessage(String code, Object... params) {
return applicationContext.getMessage(code, params, code, Locale.getDefault());
}
/**
* spring 国际化,获取enum message信息
* 尝试取得 enum.getClass().getName()+@+enum.name();
*
* @param e a enum of Type Enum
* @return
*/
public static String getMessage(Enum> e) {
if (e == null) {
return "";
}
return getMessage(e.getClass().getName() + "@" + e.name());
}
/**
* 清除applicationContext静态变量.
*/
public static void cleanApplicationContext() {
applicationContext = null;
}
private static void checkApplicationContext() {
if (applicationContext == null) {
throw new IllegalStateException("applicaitonContext未注入,请在applicationContext.xml中定义SpringContextHolder");
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy