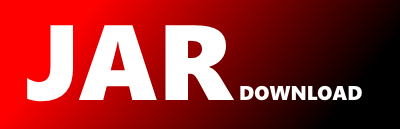
com.github.antelopeframework.web.EscapedXmlUtil Maven / Gradle / Ivy
package com.github.antelopeframework.web;
import java.io.IOException;
import javax.servlet.jsp.JspWriter;
/**
* {@link org.apache.taglibs.standard.tag.common.core.Util}
* @author yangzhi
*
*/
public class EscapedXmlUtil {
public static final int HIGHEST_SPECIAL = '>';
public static char[][] specialCharactersRepresentation = new char[HIGHEST_SPECIAL + 1][];
static {
specialCharactersRepresentation['&'] = "&".toCharArray();
specialCharactersRepresentation['<'] = "<".toCharArray();
specialCharactersRepresentation['>'] = ">".toCharArray();
specialCharactersRepresentation['"'] = """.toCharArray();
specialCharactersRepresentation['\''] = "'".toCharArray();
}
/**
*
* @param buffer
* @return
*/
public static String escapeXml(String buffer) {
if (buffer == null) {
return null;
}
int start = 0;
int length = buffer.length();
char[] arrayBuffer = buffer.toCharArray();
StringBuffer escapedBuffer = null;
for (int i = 0; i < length; i++) {
char c = arrayBuffer[i];
if (c <= HIGHEST_SPECIAL) {
char[] escaped = specialCharactersRepresentation[c];
if (escaped != null) {
// create StringBuffer to hold escaped xml string
if (start == 0) {
escapedBuffer = new StringBuffer(length + 5);
}
// add unescaped portion
if (start < i) {
escapedBuffer.append(arrayBuffer,start,i-start);
}
start = i + 1;
// add escaped xml
escapedBuffer.append(escaped);
}
}
}
// no xml escaping was necessary
if (start == 0) {
return buffer;
}
// add rest of unescaped portion
if (start < length) {
escapedBuffer.append(arrayBuffer,start,length-start);
}
return escapedBuffer.toString();
}
/**
*
* @param buffer
* @param length
* @param w
* @throws IOException
*/
public static void write(char[] buffer, int length, JspWriter w) throws IOException {
int start = 0;
for (int i = 0; i < length; i++) {
char c = buffer[i];
if (c <= HIGHEST_SPECIAL) {
char[] escaped = specialCharactersRepresentation[c];
if (escaped != null) {
// add unescaped portion
if (start < i) {
w.write(buffer, start, i - start);
}
// add escaped xml
w.write(escaped);
start = i + 1;
}
}
}
// add rest of unescaped portion
if (start < length) {
w.write(buffer, start, length - start);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy