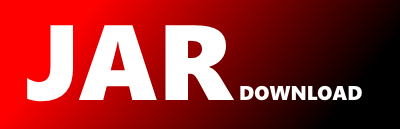
com.github.antelopeframework.dynamicproperty.MapConfiguration Maven / Gradle / Ivy
package com.github.antelopeframework.dynamicproperty;
import java.util.HashMap;
import java.util.Iterator;
import java.util.Map;
import java.util.Properties;
import java.util.concurrent.ConcurrentHashMap;
import lombok.Getter;
class MapConfiguration {
@Getter
protected Map map = new ConcurrentHashMap<>();
public MapConfiguration(Map map) {
this.map.putAll(map);
}
public MapConfiguration(Properties props) {
this.map.putAll(convertPropertiesToMap(props));
}
public void addProperty(String key, Object newValue) {
this.map.put(key, newValue);
}
public void setProperty(String key, Object newValue) {
this.map.put(key, newValue);
}
public void clearProperty(String key) {
this.map.remove(key);
}
public Object getProperty(String key) {
return this.map.get(key);
}
String getString(String key) {
Object value = this.map.get(key);
if (value == null) {
return null;
}
return value.toString();
}
public boolean isEmpty() {
return map.isEmpty();
}
public boolean containsKey(String key) {
return map.containsKey(key);
}
public Iterator getKeys() {
return map.keySet().iterator();
}
private static Map convertPropertiesToMap(final Properties props) {
Map result = new HashMap();
if (props == null || props.isEmpty()) {
return result;
}
for (Object key : props.keySet()) {
String _key = key.toString();
result.put(_key, props.getProperty(_key));
}
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy