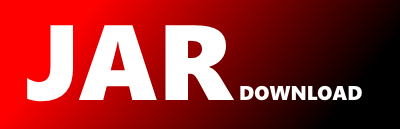
com.github.antelopeframework.dynamicproperty.zk.ZkConfigurationSource Maven / Gradle / Ivy
package com.github.antelopeframework.dynamicproperty.zk;
import java.nio.charset.Charset;
import org.apache.curator.framework.CuratorFramework;
import org.apache.zookeeper.KeeperException.NoNodeException;
import org.apache.zookeeper.KeeperException.NodeExistsException;
import com.netflix.config.source.ZooKeeperConfigurationSource;
import lombok.extern.slf4j.Slf4j;
@Slf4j
public class ZkConfigurationSource extends ZooKeeperConfigurationSource {
private final Charset charset = Charset.forName("UTF-8");
private final CuratorFramework client;
private final String configRootPath;
public ZkConfigurationSource(CuratorFramework client, String configRootPath) {
super(client, configRootPath);
this.client = client;
this.configRootPath = configRootPath;
}
public synchronized void setProperty(String key, String value) throws Exception {
final String path = configRootPath + "/" + key;
byte[] data = value.getBytes(charset);
try {
// attempt to create (intentionally doing this instead of checkExists())
client.create().creatingParentsIfNeeded().forPath(path, data);
} catch (NodeExistsException exc) {
// key already exists - update the data instead
client.setData().forPath(path, data);
}
}
public synchronized String getProperty(String key) throws Exception {
final String path = configRootPath + "/" + key;
byte[] bytes = client.getData().forPath(path);
return new String(bytes, charset);
}
public synchronized void deleteProperty(String key) throws Exception {
final String path = configRootPath + "/" + key;
try {
client.delete().forPath(path);
} catch (NoNodeException exc) {
// Node doesn't exist - NoOp
log.warn("Node doesn't exist", exc);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy