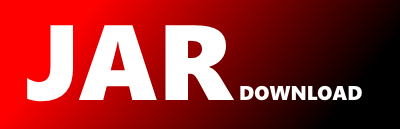
com.github.antelopeframework.mybatis.criterion.Criteria Maven / Gradle / Ivy
package com.github.antelopeframework.mybatis.criterion;
import java.io.Serializable;
import java.util.ArrayList;
import java.util.Iterator;
import java.util.List;
import org.apache.commons.lang3.StringUtils;
import lombok.Getter;
/**
* Criteria is a simplified API for retrieving entities by composing
* Criterion objects. This is a very convenient approach for
* functionality like "search" screens where there is a variable number of
* conditions to be placed upon the result set.
*
* The method Criteria.createCriteria() is a factory for
* Criteria. Criterion instances are usually obtained via the
* factory methods on Restrictions. eg.
*
*
* Criteria criteria = Criteria.createCriteria(Cat.class)
* .add(Restrictions.ilike("name", "Iz%"))
* .add(Restrictions.gt("weight", new Float(minWeight)))
* .addOrder(Order.asc("age"));
*
*
* @see Criteria#createCriteria()
* @see com.github.antelopeframework.mybatis.criterion.Restrictions
* @see com.github.antelopeframework.mybatis.criterion.Order
* @see com.github.antelopeframework.mybatis.criterion.Criterion
*
* @author yangzhi.yzh
*/
@Getter
public class Criteria implements Serializable {
private static final long serialVersionUID = 1L;
private final List criterionEntries = new ArrayList();
private final List orderEntries = new ArrayList();
private Integer maxResults = 0;
private Integer firstResult = 0;
private Criteria() {}
/**
* Create {@link Criteria} instance
*
* @return The criteria instance for manipulation and execution
*/
public static Criteria createCriteria() {
return new Criteria();
}
/**
* 是否为无条件查询; 无条件查询会对表进行全量查询, 极度损耗性能; 在findByCriteria方法时, 最好判断一下是否为无条件查询.
*
* @return
*/
public boolean isNoConditionQuery() {
return criterionEntries.size() == 0;
}
/**
* Add a {@link Criterion restriction} to constrain the results to be retrieved.
*
* @param criterion The {@link Criterion criterion} object representing the restriction to be applied.
* @return this (for method chaining)
*/
public Criteria add(Criterion expression) {
add(this, expression);
return this;
}
/**
* 如何理解hasValue:
*
* - String: {@link StringUtils#isNotBlank(CharSequence)}
* - Object: not
null
*
*
* @param expression
* @return
*/
public Criteria addIfHasValue(Criterion expression) {
if (isValueValid(expression)) {
this.add(expression);
}
return this;
}
/**
* Add an {@link Order ordering} to the result set.
*
* @param order The {@link Order order} object representing an ordering to be applied to the results.
* @return this (for method chaining)
*/
public Criteria addOrder(Order ordering) {
orderEntries.add(new OrderEntry(ordering, this));
return this;
}
/**
*
* @param maxResults
* @return
*/
public Criteria setMaxResults(int maxResults) {
if (maxResults > 0) {
this.maxResults = maxResults;
}
return this;
}
public Criteria setFirstResult(int firstResult) {
if (firstResult > 0) {
this.firstResult = firstResult;
}
return this;
}
public Iterator iterateExpressionEntries() {
return criterionEntries.iterator();
}
public Iterator iterateOrderings() {
return orderEntries.iterator();
}
private Criteria add(Criteria criteriaInst, Criterion expression) {
criterionEntries.add(new CriterionEntry(expression, criteriaInst));
return this;
}
@Getter
public static final class CriterionEntry implements Serializable {
private static final long serialVersionUID = 1L;
private final Criterion criterion;
private final Criteria criteria;
private CriterionEntry(Criterion criterion, Criteria criteria) {
this.criteria = criteria;
this.criterion = criterion;
}
@Override
public String toString() {
return criterion.toString();
}
}
@Getter
public static final class OrderEntry implements Serializable {
private static final long serialVersionUID = 1L;
private final Order order;
private final Criteria criteria;
private OrderEntry(Order order, Criteria criteria) {
this.criteria = criteria;
this.order = order;
}
@Override
public String toString() {
return order.toString();
}
}
/**
*
* @param criterion
* @return
*/
private static boolean isValueValid(Criterion expression) {
if (expression == null) {
return false;
}
if (expression instanceof Junction
|| expression instanceof LogicalExpression
|| expression instanceof SQLCriterion) {
throw new UnsupportedOperationException();
}
if (expression instanceof SimpleExpression) {
return isValueValid(((SimpleExpression) expression).getValue());
}
if (expression instanceof BetweenExpression) {
BetweenExpression be = (BetweenExpression) expression;
return isValueValid(be.getHi()) && isValueValid(be.getLo());
}
if (expression instanceof InExpression) {
return isValueValid(((InExpression) expression).getValues());
}
if (expression instanceof LikeExpression) {
return isValueValid(((LikeExpression) expression).getValue());
}
if (expression instanceof NotExpression) {
return isValueValid(((NotExpression) expression).getCriterion());
}
if (expression instanceof NotNullExpression || expression instanceof NullExpression) {
return true;
}
return true;
}
/**
*
* @param v
* @return
*/
private static boolean isValueValid(Object v) {
if (v == null) {
return false;
}
if (v instanceof String) {
StringUtils.isNotBlank((String) v);
}
return true;
}
/**
*
* @param values
* @return
*/
private static boolean isValueValid(Object[] values) {
if (values == null || values.length == 0) {
return false;
}
boolean hasValid = false;
for (Object v : values) {
if (isValueValid(v)) {
hasValid = true;
break;
}
}
return hasValid;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy