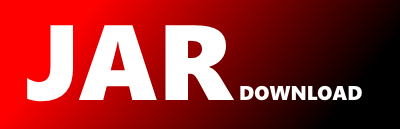
com.github.antelopeframework.mybatis.criterion.Junction Maven / Gradle / Ivy
package com.github.antelopeframework.mybatis.criterion;
import java.util.ArrayList;
import java.util.Collections;
import java.util.Iterator;
import java.util.List;
import lombok.Getter;
/**
* A sequence of a logical expressions combined by some
* associative logical operator
*
* @author yangzhi.yzh
*
*/
@Getter
public class Junction implements Criterion {
private static final long serialVersionUID = 1L;
private final Nature nature;
private final List conditions = new ArrayList();
protected Junction(Nature nature) {
this.nature = nature;
}
protected Junction(Nature nature, Criterion... criterion) {
this( nature );
Collections.addAll( conditions, criterion );
}
/**
* Adds a criterion to the junction (and/or)
*
* @param criterion The criterion to add
*
* @return {@code this}, for method chaining
*/
public Junction add(Criterion criterion) {
conditions.add( criterion );
return this;
}
@Override
public String toSqlString(CriteriaQuery criteriaQuery) {
if (conditions.size() == 0) {
return "1=1";
}
final StringBuilder buffer = new StringBuilder().append('(');
final Iterator itr = conditions.iterator();
while (itr.hasNext()) {
buffer.append(((Criterion) itr.next()).toSqlString(criteriaQuery));
if (itr.hasNext()) {
buffer.append(' ').append(nature.getOperator()).append(' ');
}
}
return buffer.append(')').toString();
}
@Override
public TypedValue[] getTypedValues(CriteriaQuery criteriaQuery) {
final ArrayList typedValues = new ArrayList();
for (Criterion condition : conditions) {
final TypedValue[] subValues = condition.getTypedValues(criteriaQuery);
Collections.addAll(typedValues, subValues);
}
return typedValues.toArray(new TypedValue[typedValues.size()]);
}
/**
* The type of junction
*/
public static enum Nature {
/**
* An AND
*/
AND,
/**
* An OR
*/
OR;
/**
* The corresponding SQL operator
*
* @return SQL operator
*/
public String getOperator() {
return name().toLowerCase();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy