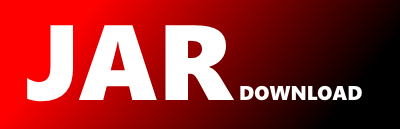
com.github.antelopeframework.mybatis.criterion.Restrictions Maven / Gradle / Ivy
package com.github.antelopeframework.mybatis.criterion;
import java.util.Collection;
import java.util.Map;
import com.github.antelopeframework.mybatis.criterion.SimpleExpression.Op;
/**
* The criterion package may be used by applications as a framework for building
* new kinds of Criterion. However, it is intended that most applications will
* simply use the built-in criterion types via the static factory methods of this class.
*
* @author yangzhi.yzh
*
* @see com.github.antelopeframework.mybatis.criterion.Criterion
*/
public class Restrictions {
/**
* Apply an "equal" constraint to the named property
*
* @param column
* @param value
* @return
*/
public static SimpleExpression eq(String column, Object value) {
return new SimpleExpression(column, value, Op.eq );
}
/**
* Apply an "equal" constraint to the named property. If the value
* is null, instead apply "is null".
*
* @param column The name of the property
* @param value The value to use in comparison
*
* @return The Criterion
*
* @see #eq
* @see #isNull
*/
public static Criterion eqOrIsNull(String column, Object value) {
return value == null ? isNull(column) : eq(column, value);
}
/**
* Apply an "is null" constraint to the named property
*
* @param column The name of the property
*
* @return Criterion
*
* @see NullExpression
*/
public static Criterion isNull(String column) {
return new NullExpression(column);
}
/**
* Apply an "is not null" constraint to the named property
*
* @param column The property name
*
* @return The Criterion
*
* @see NotNullExpression
*/
public static Criterion isNotNull(String column) {
return new NotNullExpression(column);
}
/**
* Apply a "not equal" constraint to the named property
*
* @param column The name of the property
* @param value The value to use in comparison
*
* @return The Criterion
* @see SimpleExpression
*/
public static SimpleExpression ne(String column, Object value) {
return new SimpleExpression(column, value, Op.ne);
}
/**
* Apply a "not equal" constraint to the named property. If the value
* is null, instead apply "is not null".
*
* @param column The name of the property
* @param value The value to use in comparison
*
* @return The Criterion
*
* @see #ne
* @see #isNotNull
*/
public static Criterion neOrIsNotNull(String column, Object value) {
return value == null ? isNotNull(column) : ne(column, value);
}
/**
* Apply a "greater than" constraint to the named property
*
* @param column The name of the property
* @param value The value to use in comparison
*
* @return The Criterion
*
* @see SimpleExpression
*/
public static SimpleExpression gt(String column, Object value) {
return new SimpleExpression(column, value, Op.gt);
}
/**
* Apply a "less than" constraint to the named property
*
* @param column The name of the property
* @param value The value to use in comparison
*
* @return The Criterion
*
* @see SimpleExpression
*/
public static SimpleExpression lt(String column, Object value) {
return new SimpleExpression(column, value, Op.lt);
}
/**
* Apply a "less than or equal" constraint to the named property
*
* @param column The name of the property
* @param value The value to use in comparison
*
* @return The Criterion
*
* @see SimpleExpression
*/
public static SimpleExpression le(String column, Object value) {
return new SimpleExpression(column, value, Op.le);
}
/**
* Apply a "greater than or equal" constraint to the named property
*
* @param column The name of the property
* @param value The value to use in comparison
*
* @return The Criterion
*
* @see SimpleExpression
*/
public static SimpleExpression ge(String column, Object value) {
return new SimpleExpression(column, value, Op.ge);
}
/**
* Apply a "between" constraint to the named property
*
* @param column The name of the property
* @param lo The low value
* @param hi The high value
*
* @return The Criterion
*
* @see BetweenExpression
*/
public static Criterion between(String column, Object lo, Object hi) {
return new BetweenExpression(column, lo, hi);
}
/**
* Apply an "in" constraint to the named property.
*
* @param column The name of the property
* @param values The literal values to use in the IN restriction
*
* @return The Criterion
*
* @see InExpression
*/
public static Criterion in(String column, Object[] values) {
return new InExpression( column, values);
}
/**
* Apply an "in" constraint to the named property.
*
* @param column The name of the property
* @param values The literal values to use in the IN restriction
*
* @return The Criterion
*
* @see InExpression
*/
public static Criterion in(String column, Collection> values) {
return new InExpression( column, values.toArray() );
}
/**
* Return the conjuction of two expressions
*
* @param lhs One expression
* @param rhs The other expression
*
* @return The Criterion
*/
public static LogicalExpression and(Criterion lhs, Criterion rhs) {
return new LogicalExpression(lhs, rhs, "and");
}
/**
* Return the conjuction of multiple expressions
*
* @param predicates The predicates making up the initial junction
*
* @return The conjunction
*/
public static Conjunction and(Criterion... predicates) {
return conjunction( predicates );
}
/**
* Return the disjuction of two expressions
*
* @param lhs One expression
* @param rhs The other expression
*
* @return The Criterion
*/
public static LogicalExpression or(Criterion lhs, Criterion rhs) {
return new LogicalExpression( lhs, rhs, "or" );
}
/**
* Return the disjuction of multiple expressions
*
* @param predicates The predicates making up the initial junction
*
* @return The conjunction
*/
public static Disjunction or(Criterion... predicates) {
return disjunction( predicates );
}
/**
* Return the negation of an expression
*
* @param expression The expression to be negated
*
* @return Criterion
*
* @see NotExpression
*/
public static Criterion not(Criterion expression) {
return new NotExpression( expression );
}
/**
* Group expressions together in a single conjunction (A and B and C...).
*
* This form creates an empty conjunction. See {@link Conjunction#add(Criterion)}
*
* @return Conjunction
*/
public static Conjunction conjunction() {
return new Conjunction();
}
/**
* Group expressions together in a single conjunction (A and B and C...).
*
* @param conditions The initial set of conditions to put into the Conjunction
*
* @return Conjunction
*/
public static Conjunction conjunction(Criterion... conditions) {
return new Conjunction( conditions );
}
/**
* Group expressions together in a single disjunction (A or B or C...).
*
* This form creates an empty disjunction. See {@link Disjunction#add(Criterion)}
*
* @return Conjunction
*/
public static Disjunction disjunction() {
return new Disjunction();
}
/**
* Group expressions together in a single disjunction (A or B or C...).
*
* @param conditions The initial set of conditions to put into the Disjunction
*
* @return Conjunction
*/
public static Disjunction disjunction(Criterion... conditions) {
return new Disjunction( conditions );
}
/**
* Apply an "equals" constraint to each property in the key set of a Map
*
* @param columnValues a map from property names to values
*
* @return Criterion
*
* @see Conjunction
*/
public static Criterion allEq(Map columnValues) {
final Conjunction conj = conjunction();
for (Map.Entry entry : columnValues.entrySet()) {
String column = entry.getKey();
TypedValue value = entry.getValue();
conj.add(eq(column, value.getValue()));
}
return conj;
}
/**
* A case-insensitive "like" (similar to Postgres ilike operator)
*
* @param column The name of the property
* @param value The value to use in comparison
*
* @return The Criterion
*
* @see LikeExpression
*/
public static Criterion like(String column, Object value) {
if (value == null) {
throw new IllegalArgumentException("Comparison value passed to ilike cannot be null");
}
return like(column, value.toString(), MatchMode.ANYWHERE);
}
/**
* A case-insensitive "like" (similar to Postgres ilike operator)
*
* @param column The name of the property
* @param value The value to use in comparison
*
* @return The Criterion
*
* @see LikeExpression
*/
public static Criterion like(String column, Object value, boolean ignoreCase) {
if (value == null) {
throw new IllegalArgumentException("Comparison value passed to ilike cannot be null");
}
return like(column, value.toString(), MatchMode.ANYWHERE, ignoreCase);
}
/**
* A case-insensitive "like" (similar to Postgres ilike operator) using the provided match mode
*
* @param column The name of the property
* @param value The value to use in comparison
* @param matchMode The match mode to use in comparison
*
* @return The Criterion
*
* @see LikeExpression
*/
public static Criterion like(String column, String value, MatchMode matchMode) {
if (value == null) {
throw new IllegalArgumentException("Comparison value passed to ilike cannot be null");
}
return new LikeExpression(column, value, matchMode, null, true);
}
/**
* A case-insensitive "like" (similar to Postgres ilike operator) using the provided match mode
*
* @param column The name of the property
* @param value The value to use in comparison
* @param matchMode The match mode to use in comparison
* @param ignoreCase
*
* @return The Criterion
*
* @see LikeExpression
*/
public static Criterion like(String column, String value, MatchMode matchMode, boolean ignoreCase) {
if (value == null) {
throw new IllegalArgumentException("Comparison value passed to ilike cannot be null");
}
return new LikeExpression(column, value, matchMode, null, ignoreCase);
}
/**
* Create a restriction expressed in SQL with JDBC parameters. Any occurrences of {alias} will be
* replaced by the table alias.
*
* @param sql The SQL restriction
* @param values The parameter values
* @param sqlTypes The parameter types
*
* @return The Criterion
*
* @see SQLCriterion
*/
public static Criterion sqlRestriction(String sql, Object[] values, int[] sqlTypes) {
return new SQLCriterion(sql, values, sqlTypes);
}
/**
* Create a restriction expressed in SQL with one JDBC parameter. Any occurrences of {alias} will be
* replaced by the table alias.
*
* @param sql The SQL restriction
* @param value The parameter value
* @param sqlType The parameter type
*
* @return The Criterion
*
* @see SQLCriterion
*/
public static Criterion sqlRestriction(String sql, Object value, int sqlType) {
return new SQLCriterion(sql, value, sqlType);
}
/**
* Apply a constraint expressed in SQL with no JDBC parameters. Any occurrences of {alias} will be
* replaced by the table alias.
*
* @param sql The SQL restriction
*
* @return The Criterion
*
* @see SQLCriterion
*/
public static Criterion sqlRestriction(String sql) {
return new SQLCriterion(sql);
}
protected Restrictions() {
// cannot be instantiated, but needs to be protected so Expression can extend it
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy