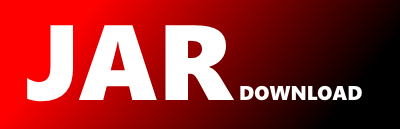
com.github.antelopeframework.mybatis.criterion.SimpleExpression Maven / Gradle / Ivy
package com.github.antelopeframework.mybatis.criterion;
import java.sql.Types;
import lombok.Getter;
/**
* superclass for "simple" comparisons (with SQL binary operators)
*
* @author yangzhi.yzh
*/
@Getter
public class SimpleExpression implements Criterion {
private static final long serialVersionUID = 1L;
private final String column;
private final int sqlType;
private final Object value;
private boolean ignoreCase;
private final String op;
protected enum Op {
eq("="), ge(">="), gt(">"), le("<="), lt("<"), ne("<>");
final String value;
private Op(String value) {
this.value = value;
}
}
protected SimpleExpression(String column, Object value, Op op) {
this.column = column;
this.value = value;
this.op = op.value;
this.sqlType = sqlType(value);
}
protected SimpleExpression(String propertyName, Object value, Op op, boolean ignoreCase) {
this.column = propertyName;
this.value = value;
this.ignoreCase = ignoreCase;
this.op = op.value;
this.sqlType = sqlType(value);
}
private static int sqlType(Object value) {
int sqlType = Types.OTHER;
if (value instanceof Character) {
sqlType = Types.CHAR;
} else if (value instanceof String) {
sqlType = Types.VARCHAR;
}
return sqlType;
}
/**
* Make case insensitive. No effect for non-String values
*
* @return {@code this}, for method chaining
*/
public SimpleExpression ignoreCase() {
ignoreCase = true;
return this;
}
@Override
public String toSqlString(CriteriaQuery criteriaQuery) {
final StringBuilder fragment = new StringBuilder();
final boolean lower = ignoreCase && sqlType == Types.VARCHAR || sqlType == Types.CHAR;
if (lower) {
fragment.append("lower").append('(');
}
fragment.append(column);
if (lower) {
fragment.append(')');
}
fragment.append(getOp()).append("?");
return fragment.toString();
}
@Override
public TypedValue[] getTypedValues(CriteriaQuery criteriaQuery) {
return new TypedValue[]{new TypedValue(null, value)};
}
@Override
public String toString() {
return column + getOp() + value;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy