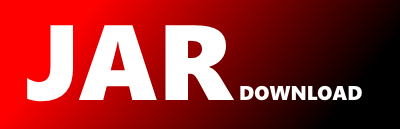
com.github.antelopeframework.remoting.client.HessianProxy Maven / Gradle / Ivy
The newest version!
package com.github.antelopeframework.remoting.client;
import java.lang.reflect.Method;
import java.net.InetAddress;
import java.net.URL;
import java.net.UnknownHostException;
import java.util.UUID;
import com.caucho.hessian.client.HessianConnection;
import com.caucho.hessian.client.HessianProxyFactory;
import com.github.antelopeframework.remoting.server.HessianServiceExporter;
import lombok.Setter;
import lombok.extern.slf4j.Slf4j;
@Slf4j
@Setter
public class HessianProxy extends com.caucho.hessian.client.HessianProxy {
private static final long serialVersionUID = 1L;
private static final String XID_PATTERN = "%s_%s_%s";
private static String LOCAL_ADDRESS = "";
private static ThreadLocal THREADLOCAL_XIDS = new ThreadLocal<>();
static {
try {
String hostName = InetAddress.getLocalHost().getHostName();
LOCAL_ADDRESS = InetAddress.getByName(hostName).getHostAddress();
} catch (UnknownHostException e) {
//DO-NOTHING
}
}
private String app;
protected HessianProxy(URL url, HessianProxyFactory factory) {
super(url, factory);
}
protected HessianProxy(URL url, HessianProxyFactory factory, Class> type) {
super(url, factory, type);
}
@Override
public Object invoke(Object proxy, Method method, Object []args) throws Throwable {
String xid = xid();
THREADLOCAL_XIDS.set(xid);
long start = System.currentTimeMillis();
Object result = super.invoke(proxy, method, args);
if (log.isInfoEnabled()) {
log.info("remoting call: {}.{}, execution time[msec]: {}, xid: {}",
method.getDeclaringClass().getName(), method.getName(), System.currentTimeMillis() - start, xid);
}
return result;
}
@Override
protected void addRequestHeaders(HessianConnection conn) {
super.addRequestHeaders(conn);
//~ 增加两个额外参数: 请求的应用名称和请求时间戳
conn.addHeader(HessianServiceExporter.HTTPINVOKER_PARAM_APP, this.app);
conn.addHeader(HessianServiceExporter.HTTPINVOKER_PARAM_XID, THREADLOCAL_XIDS.get());
}
private static String xid() {
return String.format(XID_PATTERN, LOCAL_ADDRESS, System.currentTimeMillis(), UUID.randomUUID().toString());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy