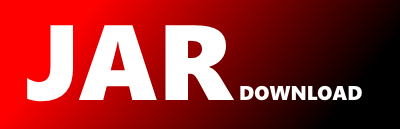
com.github.antelopeframework.remoting.server.HessianServiceGroupFactory Maven / Gradle / Ivy
The newest version!
package com.github.antelopeframework.remoting.server;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Map;
import java.util.Map.Entry;
import java.util.Set;
import org.springframework.beans.BeansException;
import org.springframework.beans.factory.config.BeanDefinition;
import org.springframework.beans.factory.config.BeanFactoryPostProcessor;
import org.springframework.beans.factory.config.ConfigurableListableBeanFactory;
import org.springframework.beans.factory.support.BeanDefinitionBuilder;
import org.springframework.beans.factory.support.DefaultListableBeanFactory;
import org.springframework.core.Ordered;
import org.springframework.core.PriorityOrdered;
import com.github.antelopeframework.remoting.server.auth.RemoteCallAuthenticate;
import lombok.Getter;
import lombok.Setter;
public class HessianServiceGroupFactory implements BeanFactoryPostProcessor, PriorityOrdered {
private Set types = new HashSet<>();
@Setter
private Map, Object> services = new HashMap<>();
@Setter
@Getter
private int order = Ordered.LOWEST_PRECEDENCE;
// @Setter
// private String[] apiPackagesToScan;
@Setter
private RemoteCallAuthenticate remoteCallAuthenticate;
// @Setter
// private ApplicationContext applicationContext;
// public Object postProcessAfterInitialization(Object bean, String beanName) throws BeansException {
// DefaultListableBeanFactory listableBeanFactory = (DefaultListableBeanFactory) beanFactory;
//
// if (apiPackagesToScan != null && apiPackagesToScan.length > 0) {
// Class>[] serviceInterfaces = bean.getClass().getInterfaces();
// if (serviceInterfaces != null && serviceInterfaces.length != 0) {
// for (Class> type : serviceInterfaces) {
// if (types.contains(type)) {
// continue;
// }
//
// if (StringUtils.startsWithAny(type.getName(), apiPackagesToScan)) {
// String[] names = listableBeanFactory.getBeanNamesForType(type);
// if (names != null && names.length > 1) {
// log.warn("more than one bean defined for type: {}, not auto exported to remote service; please use 'services' to expote.",
// type.getClass().getName());
//
// continue;
// }
//
// listableBeanFactory.registerBeanDefinition("/" + type.getName(), createServiceExporter(type, bean));
// types.add(type.getName());
// }
// }
// }
// }
//
// List> serviceInterfaces = findServiceInterfaceWithRemoteService(bean);
// if (serviceInterfaces != null && serviceInterfaces.size() != 0) {
// for (Class> type : serviceInterfaces) {
// if (types.contains(type)) {
// continue;
// }
//
// String[] names = listableBeanFactory.getBeanNamesForType(type);
// if (names != null && names.length > 1) {
// log.warn("more than one bean defined for type: {}, not auto exported to remote service; please use 'services' to expote.",
// type.getClass().getName());
//
// continue;
// }
//
// listableBeanFactory.registerBeanDefinition("/" + type.getName(), createServiceExporter(type, bean));
// types.add(type.getName());
// }
// }
//
// return bean;
// }
/*
* (non-Javadoc)
* @see org.springframework.beans.factory.config.BeanFactoryPostProcessor#postProcessBeanFactory(org.springframework.beans.factory.config.ConfigurableListableBeanFactory)
*/
@Override
public void postProcessBeanFactory(ConfigurableListableBeanFactory beanFactory) throws BeansException {
DefaultListableBeanFactory listableBeanFactory = (DefaultListableBeanFactory) beanFactory;
if (services != null) {
for (Entry, Object> entry : services.entrySet()) {
String _beanName = "/" + entry.getKey().getName();
listableBeanFactory.registerBeanDefinition(_beanName, createServiceExporter(entry.getKey(), entry.getValue()));
types.add(entry.getKey().getName());
}
}
//~ 处理完成后, 清理掉缓存的配置信息
services = null;
types = null;
}
// private List> findServiceInterfaceWithRemoteService(Object service) {
// List> serviceInterfaces = new ArrayList>();
// for (Class> interfaceClass : service.getClass().getInterfaces()) {
// if (AnnotationUtils.isAnnotationDeclaredLocally(RemoteService.class, interfaceClass)) {
// serviceInterfaces.add(interfaceClass);
// }
// }
//
// return serviceInterfaces;
// }
//
private BeanDefinition createServiceExporter(Class> serviceInterface, Object service) {
BeanDefinitionBuilder beanDefinitionBuilder = BeanDefinitionBuilder.rootBeanDefinition(HessianServiceExporter.class);
beanDefinitionBuilder.addPropertyValue("serviceInterface", serviceInterface);
beanDefinitionBuilder.addPropertyValue("service", service);
beanDefinitionBuilder.addPropertyValue("remoteCallAuthenticate", remoteCallAuthenticate);
return beanDefinitionBuilder.getBeanDefinition();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy