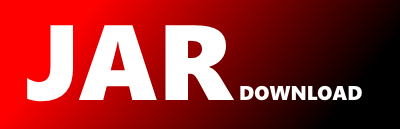
com.logicbus.backend.message.tools.GzipTool Maven / Gradle / Ivy
package com.logicbus.backend.message.tools;
import com.anysoft.util.Properties;
import com.anysoft.util.PropertiesConstants;
import com.anysoft.util.compress.Compressor;
import com.anysoft.util.compress.compressor.GZIP;
import com.logicbus.backend.Context;
import org.apache.commons.lang3.StringUtils;
import java.io.IOException;
import java.io.InputStream;
/**
* Gzip操作相关的工具
*/
public class GzipTool {
/**
* Gzip压缩工具
*/
private static Compressor gzipCompressor = new GZIP();
/**
* 是否开启gzip
*/
private static boolean gzipSupport = false;
/**
* gzip开启的报文长度
*/
private static int gzipEnableLength = 1024;
public GzipTool(Properties p){
gzipSupport = PropertiesConstants.getBoolean(p,"http.json.gzip",gzipSupport);
gzipEnableLength = PropertiesConstants.getInt(p,"http.gzip.length",gzipEnableLength);
}
public boolean isRequestGzipEnable(Context ctx){
if (!gzipSupport) {
return false;
}
String gzip = ctx.getRequestHeader("Content-Encoding");
return StringUtils.isNotEmpty(gzip)?gzip.equalsIgnoreCase("gzip"):false;
}
public boolean isResponseGzipEnable(Context ctx){
if (!gzipSupport) {
return false;
}
String gzip = ctx.getRequestHeader("Accept-Encoding");
return StringUtils.isNotEmpty(gzip)?gzip.equalsIgnoreCase("gzip"):false;
}
public byte[] decompress(Context ctx,byte[] input){
return input.length > 0 ? gzipCompressor.decompress(input):input;
}
public byte[] compress(Context ctx,byte[] input){
if (gzipEnableLength < input.length){
ctx.setResponseHeader("Content-Encoding","gzip");
return gzipCompressor.compress(input);
}
return input;
}
public InputStream getInputStream(Context ctx, InputStream in) throws IOException {
return in.available() > 0 ? gzipCompressor.getInputStream(in):in;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy