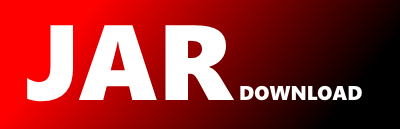
com.alogic.timer.core.Task Maven / Gradle / Ivy
package com.alogic.timer.core;
import java.util.HashMap;
import java.util.Iterator;
import java.util.Map;
import java.util.Map.Entry;
import org.w3c.dom.Document;
import org.w3c.dom.Element;
import com.anysoft.util.DefaultProperties;
import com.anysoft.util.JsonSerializer;
import com.anysoft.util.JsonTools;
import com.anysoft.util.Reportable;
/**
* 任务
*
* @author duanyy
* @since 1.6.3.37
*/
public interface Task extends JsonSerializer,Reportable{
/**
* 任务状态
*
* @author duanyy
*
*/
public static enum State{
/**
* 新建
*/
New,
/**
* 已进入队列
*/
Queued,
/**
* 已从队列中领取
*/
Polled,
/**
* 正在运行
*/
Running,
/**
* 运行失败
*/
Failed,
/**
* 已完成
*/
Done
}
/**
* 获取任务ID
* @return id
*/
public String id();
/**
* 获取任务队列
* @return 队列ID
*/
public String queue();
/**
* 任务参数
* @return 任务参数
*/
public DefaultProperties getParameters();
/**
* 缺省实现
*
* @author duanyy
*
*/
public static class Default implements Task{
protected String id;
protected String queue;
protected DefaultProperties p;
public Default(String _id,String _queue){
this(_id,_queue,(DefaultProperties)null);
}
public Default(String _id,String _queue,Map _p){
id = _id;
queue = _queue;
p = new DefaultProperties();
if (_p != null){
Iterator> iter = _p.entrySet().iterator();
while (iter.hasNext()){
Entry entry = iter.next();
p.SetValue(entry.getKey(), entry.getValue());
}
}
}
public Default(String _id,String _queue,DefaultProperties _p){
id = _id;
queue = _queue;
p = _p;
}
public Default(Map json){
fromJson(json);
}
public void toJson(Map json) {
if (json != null){
json.put("id", id);
json.put("queue", queue);
Map parameters = new HashMap();
p.toJson(parameters);
json.put("parameters", parameters);
}
}
@SuppressWarnings("unchecked")
public void fromJson(Map json) {
if (json != null){
id = JsonTools.getString(json, "id", "");
queue = JsonTools.getString(json, "queue", id);
if (p == null){
p = new DefaultProperties();
}
Object parameters = json.get("parameters");
if (parameters instanceof Map){
p.fromJson((Map)parameters);
}
}
}
public void toXML(Element root) {
if (root != null){
root.setAttribute("id", id);
root.setAttribute("queue", queue);
if (p != null){
Document doc = root.getOwnerDocument();
Element _parameters = doc.createElement("parameters");
p.toXML(_parameters);
root.appendChild(_parameters);
}
}
}
public void report(Element xml) {
toXML(xml);
}
public void report(Map json) {
toJson(json);
}
public String id() {
return id;
}
public String queue() {
return queue;
}
public DefaultProperties getParameters() {
return p;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy