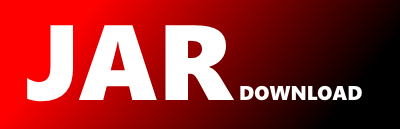
com.alogic.kube.KubeApiClients Maven / Gradle / Ivy
package com.alogic.kube;
import io.kubernetes.client.openapi.ApiClient;
import io.kubernetes.client.openapi.Configuration;
import io.kubernetes.client.util.ClientBuilder;
import io.kubernetes.client.util.KubeConfig;
import io.kubernetes.client.util.credentials.AccessTokenAuthentication;
import org.apache.commons.lang3.StringUtils;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.io.File;
import java.io.FileReader;
import java.io.IOException;
import java.util.Map;
import java.util.concurrent.ConcurrentHashMap;
/**
* API client tools
*
* @since 1.6.16.14
*/
public final class KubeApiClients {
/**
* the id of default api client
*/
public static final String DEFAULT = "default";
/**
* a logger of slf4j
*/
protected static final Logger LOG = LoggerFactory.getLogger(KubeApiClients.class);
/**
* cached api clients which can be get back by id
*/
protected static Map clients = new ConcurrentHashMap();
/**
* to get default api client
* @return api client
*/
public static ApiClient getDefaultApiClient(){
ApiClient client = clients.get(DEFAULT);
return client != null ? client : Configuration.getDefaultApiClient();
}
/**
* to registry a api client
* @param id id of the api client
* @param client client instance
* @return client instance
*/
public static ApiClient registryClient(String id,ApiClient client){
clients.put(id,client);
return client;
}
public static ApiClient unregistryClient(String id){
return clients.remove(id);
}
public static ApiClient getClient(String id,boolean defaultIsOk){
ApiClient client = clients.get(id);
if (client == null){
return defaultIsOk?getDefaultApiClient():null;
}else{
return client;
}
}
public static ApiClient getClientFromCluster(){
ApiClient apiClient = null;
try {
apiClient = ClientBuilder.oldCluster().build();
LOG.info("Load kube config from cluster..");
}catch (IOException ex){
LOG.error("Can not create api client from cluster..");
}
return apiClient;
}
public static ApiClient getClientFromCluster(String clientId){
ApiClient client = getClientFromCluster();
if (StringUtils.isNotEmpty(clientId) && client != null){
registryClient(clientId,client);
}
return client;
}
/**
* to get api client from kube-config file
* @param config file path of kube-config
* @return api client instance
*/
public static ApiClient getClientFromConfig(String config,boolean tryCluster){
ApiClient apiClient = null;
try {
File file = new File(config);
if (file.exists() && file.isFile() && file.canRead()) {
apiClient = ClientBuilder.kubeconfig(
KubeConfig.loadKubeConfig(new FileReader(file))
).build();
LOG.info("Load kube config from file:{}",config);
}else{
LOG.warn("Kube config file {} does not exist or can not be read.",config);
if (tryCluster){
apiClient = getClientFromCluster();
}
}
}catch (IOException ex){
LOG.error("Can not create api client from config file: {}",config);
}
return apiClient;
}
public static ApiClient getClientFromConfig(String config,boolean tryCluster,String clientId){
ApiClient client = getClientFromConfig(config,tryCluster);
if (StringUtils.isNotEmpty(clientId) && client != null){
registryClient(clientId,client);
}
return client;
}
/**
* to create an api client with url and access token
* @param url url like https://xxx.xxx.xxx.xxx
* @param token access token
* @return api client instance
*/
public static ApiClient getClient(String url,String token){
ClientBuilder builder = new ClientBuilder();
builder.setBasePath(url);
builder.setVerifyingSsl(false);
builder.setAuthentication(new AccessTokenAuthentication(token));
return builder.build();
}
public static ApiClient getClient(String url,String token,String clientId){
ApiClient client = getClient(url,token);
if (StringUtils.isNotEmpty(clientId) && client != null){
registryClient(clientId,client);
}
return client;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy