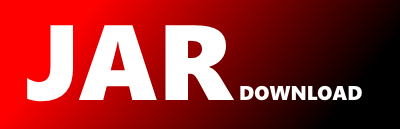
com.alogic.kube.handler.CommonHandler Maven / Gradle / Ivy
package com.alogic.kube.handler;
import com.alogic.kube.KubeHandler;
import com.alogic.kube.api.ListParams;
import com.alogic.kube.model.CommonObject;
import com.alogic.kube.model.CommonObjectList;
import com.alogic.xscript.Logiclet;
import com.alogic.xscript.LogicletContext;
import com.alogic.xscript.Script;
import com.alogic.xscript.doc.XsObject;
import com.alogic.xscript.doc.json.JsonObject;
import com.anysoft.util.*;
import io.kubernetes.client.openapi.ApiClient;
import io.kubernetes.client.openapi.ApiException;
import io.kubernetes.client.openapi.Pair;
import io.kubernetes.client.util.CallGenerator;
import io.kubernetes.client.util.CallGeneratorParams;
import okhttp3.Call;
import org.apache.commons.lang3.StringUtils;
import org.apache.commons.lang3.exception.ExceptionUtils;
import org.w3c.dom.Element;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
/**
* 通用处理器
*/
public class CommonHandler extends KubeHandler {
protected String servicePath;
protected Logiclet onAdd = null;
protected Logiclet onUpdate = null;
protected Logiclet onDelete = null;
@Override
public boolean isOk(){
return super.isOk() && StringUtils.isNotEmpty(servicePath);
}
@Override
public void configure(Properties p){
super.configure(p);
servicePath = PropertiesConstants.getString(p,"path","");
}
@Override
public void configure(Element e, Properties p) {
XmlElementProperties xmlElementProperties = new XmlElementProperties(e,p);
configure(xmlElementProperties);
Element elem = XmlTools.getFirstElementByPath(e, "on-add");
if (elem != null){
onAdd = Script.create(elem, xmlElementProperties);
}
elem = XmlTools.getFirstElementByPath(e, "on-update");
if (elem != null){
onUpdate = Script.create(elem, xmlElementProperties);
}
elem = XmlTools.getFirstElementByPath(e, "on-delete");
if (elem != null){
onDelete = Script.create(elem, xmlElementProperties);
}
}
@Override
protected CallGenerator buildCall(ApiClient apiClient) {
return (CallGeneratorParams params) -> listCall(apiClient,servicePath,listParams,params);
}
@Override
protected Class getObjClass() {
return CommonObject.class;
}
@Override
protected Class getListObjClass() {
return CommonObjectList.class;
}
@Override
public void onAdd(CommonObject obj) {
if (onAdd != null){
LogicletContext logicletContext = new LogicletContext(Settings.get());
try {
Map root = new HashMap();
root.put("$current",obj);
XsObject doc = new JsonObject("root",root);
onAdd.execute(doc,doc, logicletContext, null);
}catch (Exception ex){
LOG.info("Failed to process on_add event:" + ExceptionUtils.getStackTrace(ex));
}
}
}
@Override
public void onUpdate(CommonObject oldObj, CommonObject newObj) {
if (onUpdate != null){
LogicletContext logicletContext = new LogicletContext(Settings.get());
try {
Map root = new HashMap();
root.put("$current",newObj);
root.put("$old",oldObj);
XsObject doc = new JsonObject("root",root);
onUpdate.execute(doc,doc, logicletContext, null);
}catch (Exception ex){
LOG.info("Failed to process on_update event:" + ExceptionUtils.getStackTrace(ex));
}
}
}
@Override
public void onDelete(CommonObject obj, boolean deletedFinalStateUnknown) {
if (onDelete != null){
LogicletContext logicletContext = new LogicletContext(Settings.get());
try {
Map root = new HashMap();
root.put("$current",obj);
root.put("$deletedFinalStateUnknown",Boolean.toString(deletedFinalStateUnknown));
XsObject doc = new JsonObject("root",root);
onDelete.execute(doc,doc, logicletContext, null);
}catch (Exception ex){
LOG.info("Failed to process on_delete event:" + ExceptionUtils.getStackTrace(ex));
}
}
}
protected Call listCall(ApiClient apiClient, String path, ListParams params, CallGeneratorParams callGeneratorParams) throws ApiException {
Object localVarPostBody = null;
String localVarPath = path;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
if (params != null){
params.addParams(localVarQueryParams,apiClient);
}
if (StringUtils.isNotEmpty(callGeneratorParams.resourceVersion)) {
localVarQueryParams.add(new Pair("resourceVersion",
apiClient.parameterToString(callGeneratorParams.resourceVersion)));
}
if (callGeneratorParams.timeoutSeconds != null) {
localVarQueryParams.add(new Pair("timeoutSeconds",
apiClient.parameterToString(callGeneratorParams.timeoutSeconds)));
}
if (callGeneratorParams.watch != null) {
localVarQueryParams.add(new Pair("watch",
apiClient.parameterToString(callGeneratorParams.watch)));
}
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
String[] localVarAccepts = new String[]{"application/json", "application/yaml", "application/vnd.kubernetes.protobuf", "application/json;stream=watch", "application/vnd.kubernetes.protobuf;stream=watch"};
String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) {
localVarHeaderParams.put("Accept", localVarAccept);
}
String[] localVarContentTypes = new String[0];
String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
String[] localVarAuthNames = new String[]{"BearerToken"};
return apiClient.buildCall(localVarPath, "GET",
localVarQueryParams,
localVarCollectionQueryParams,
localVarPostBody,
localVarHeaderParams,
localVarCookieParams,
localVarFormParams,
localVarAuthNames, null);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy