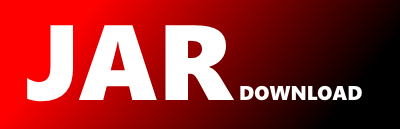
com.alogic.kube.scanner.FromClasspath Maven / Gradle / Ivy
package com.alogic.kube.scanner;
import com.alogic.kube.KubeHandler;
import com.alogic.kube.handler.CommonHandler;
import com.alogic.load.Scanner;
import org.apache.commons.lang3.StringUtils;
import org.apache.commons.lang3.exception.ExceptionUtils;
import org.w3c.dom.Document;
import com.anysoft.util.*;
import java.io.File;
import java.io.FileInputStream;
import java.io.IOException;
import java.io.InputStream;
import java.net.URISyntaxException;
import java.net.URL;
import java.util.Enumeration;
import java.util.jar.JarEntry;
import java.util.jar.JarFile;
/**
* 从classpath加载
*/
public class FromClasspath extends Scanner.Abstract {
/**
* 类路径
*/
protected String path;
/**
* 引导类
*/
protected String bootstrap;
protected String suffix = ".xml";
@Override
public void configure(Properties p){
super.configure(p);
path = PropertiesConstants.getString(p, "path", "",true);
bootstrap = PropertiesConstants.getString(p, "bootstrap", "",true);
suffix = PropertiesConstants.getString(p,"suffix",suffix,true);
}
@Override
public void scan(Listener listener) {
if (listener != null){
Object cookie = listener.begin(this.getId());
if (StringUtils.isNotEmpty(path) && StringUtils.isNotEmpty(bootstrap)){
ClassLoader cl = Settings.getClassLoader();
try {
Class> clazz = cl.loadClass(bootstrap);
scanResource(path,clazz,listener,cookie);
}catch (Exception ex){
LOG.error("Can not load class {}",bootstrap);
LOG.error(ExceptionUtils.getStackTrace(ex));
}
}else{
LOG.error("Can not scan class path,because the path or the bootstap is null");
}
listener.end(cookie,this.getId());
}
}
protected void scanResource(String home, Class> clazz, Listener listener, Object cookie) {
URL url = clazz.getResource(home);
if (url == null){
LOG.warn("Can not find resource path: {}",home);
return ;
}
if(url.toString().startsWith("file:")){
File file;
try {
file = new File(url.toURI());
} catch (URISyntaxException e) {
LOG.error("Can not scan home: {}",path);
LOG.error(ExceptionUtils.getStackTrace(e));
return;
}
LOG.info("Scan kube handler in {}",url.toString());
scanFileSystem(home,file,clazz,listener,cookie);
}
else{
if (url.toString().startsWith("jar:")){
JarFile jfile;
try {
String jarUrl = url.toString();
jarUrl = jarUrl.substring(9, jarUrl.indexOf('!'));
jfile = new JarFile(jarUrl);
} catch (IOException e) {
LOG.error("Can not scan home: {}",path);
LOG.error(ExceptionUtils.getStackTrace(e));
return;
}
LOG.info("Scan kube handler in {}",url.toString());
scanJar(home,jfile,clazz,listener,cookie);
}
}
}
/**
* 扫描指定的Jar
* @param home Jar中的路径
* @param jarFile jarFile
*/
protected void scanJar(String home, JarFile jarFile, Class> clazz, Listener listener, Object cookie) {
Enumeration files = jarFile.entries();
while (files.hasMoreElements()) {
JarEntry entry = files.nextElement();
String name = entry.getName();
if (name.endsWith(suffix)) {
int end = name.lastIndexOf('/');
if (end > 0) {
String path = '/' + name.substring(0, end);
if (path.equals(home)) {
LOG.info("Found kube handler : {}", entry.getName());
String classPath = "/" + name;
;
InputStream in = null;
try {
in = clazz.getResourceAsStream(classPath);
Factory f = new Factory();
Document doc = XmlTools.loadFromInputStream(in);
KubeHandler handler = f.newInstance(
doc.getDocumentElement(),Settings.get(),"module", CommonHandler.class.getName());
listener.found(cookie,handler);
} catch (Exception ex) {
LOG.error("Can not load file:" + classPath);
LOG.error(ExceptionUtils.getStackTrace(ex));
} finally {
IOTools.close(in);
}
}
}
}
}
}
/**
* 扫描文件系统
* @param path
* @param file
* @param clazz
*/
protected void scanFileSystem(String path, File file, Class> clazz, Listener listener, Object cookie) {
File[] files = file.listFiles();
for (File item:files){
if (item.getName().endsWith(suffix)){
LOG.info("Found kube handler : {}",item.getName());
InputStream in = null;
try {
in = new FileInputStream(item);
Factory f = new Factory();
Document doc = XmlTools.loadFromInputStream(in);
KubeHandler handler = f.newInstance(
doc.getDocumentElement(),Settings.get(),"module", CommonHandler.class.getName());
listener.found(cookie,handler);
}catch (Exception ex){
LOG.error("Can not load file:" + item.getPath());
LOG.error(ExceptionUtils.getStackTrace(ex));
}finally{
IOTools.close(in);
}
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy