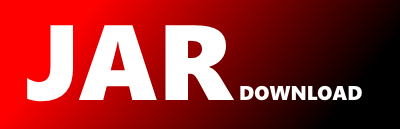
com.alogic.kube.scanner.FromLocalPath Maven / Gradle / Ivy
package com.alogic.kube.scanner;
import com.alogic.kube.KubeHandler;
import com.alogic.kube.handler.CommonHandler;
import com.alogic.load.Scanner;
import org.apache.commons.lang3.StringUtils;
import org.w3c.dom.Document;
import com.anysoft.util.*;
import java.io.File;
import java.io.FileInputStream;
import java.io.InputStream;
/**
* 从本地文件系统加载
*/
public class FromLocalPath extends Scanner.Abstract {
protected String home;
protected String suffix = ".xml";
@Override
public void configure(Properties p){
super.configure(p);
home = PropertiesConstants.getString(p, "path", "",true);
suffix = PropertiesConstants.getString(p,"suffix",suffix,true);
}
@Override
public void scan(Listener listener) {
if (listener != null){
Object cookie = listener.begin(this.getId());
if (StringUtils.isNotEmpty(home)){
LOG.info("Scan kube handler in {}",home);
File root = new File(home);
if (root.exists() && root.isDirectory()){
scanFileSystem(home,root,listener,cookie);
}
}
listener.end(cookie,this.getId());
}
}
/**
* 扫描文件系统
* @param path
* @param file
*/
protected void scanFileSystem(String path, File file, Listener listener, Object cookie) {
Factory f = new Factory();
File[] files = file.listFiles();
for (File item:files){
if (item.getName().endsWith(suffix)){
LOG.info("Found kube handler : {}",item.getName());
KubeHandler handler = loadFromFile(item,f);
if (handler != null){
if (listener != null){
listener.found(cookie,handler);
}
}
}
}
}
protected KubeHandler loadFromFile(File file, Factory f){
InputStream in = null;
try {
in = new FileInputStream(file);
Document doc = XmlTools.loadFromInputStream(in);
return f.newInstance(
doc.getDocumentElement(),Settings.get(),"module", CommonHandler.class.getName());
}catch (Exception ex){
LOG.error("Can not create kube handler :{}",file.getPath());
return null;
}finally{
IOTools.close(in);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy