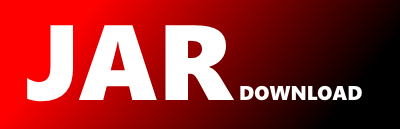
com.alogic.kube.xscript.api.KubeQuery Maven / Gradle / Ivy
package com.alogic.kube.xscript.api;
import com.alogic.kube.KubeApiClients;
import com.alogic.xscript.ExecuteWatcher;
import com.alogic.xscript.Logiclet;
import com.alogic.xscript.LogicletContext;
import com.alogic.xscript.doc.XsObject;
import com.alogic.xscript.plugins.Segment;
import com.anysoft.util.Properties;
import com.anysoft.util.PropertiesConstants;
import io.kubernetes.client.openapi.ApiClient;
import okhttp3.Response;
import org.apache.commons.lang3.StringUtils;
/**
* 查询操作
*
* @since 1.6.16.14
*/
public abstract class KubeQuery extends Segment {
protected String pid = KubeUtil.DFT_CONTEXT_ID;
protected String $result = KubeUtil.DFT_RESULT;
protected KueListParams listParams = new KueListParams();
protected String $path = null;
protected String $kubeClientId = KubeApiClients.DEFAULT;
public KubeQuery(String tag, Logiclet p) {
super(tag, p);
}
public KueListParams getListParams(){
return listParams;
}
@Override
public void configure(Properties p){
super.configure(p);
pid = PropertiesConstants.getString(p, "pid", pid,true);
$path = PropertiesConstants.getRaw(p,"path",$path);
$result = PropertiesConstants.getRaw(p,"id",$result);
$kubeClientId = PropertiesConstants.getRaw(p,"kubeId",$kubeClientId);
listParams.configure(p);
}
public String getApiPath(Properties ctx){
return PropertiesConstants.transform(ctx,$path,null);
}
public String getResultId(Properties ctx){
return PropertiesConstants.transform(ctx,$result,KubeUtil.DFT_RESULT);
}
public String getCodeId(String resultId){
return String.format("%s.code",resultId);
}
public String getReasonId(String resultId){
return String.format("%s.reason",resultId);
}
protected void handleResult(Properties ctx, Response response){
String resultId = getResultId(ctx);
if (StringUtils.isNotEmpty(resultId)){
PropertiesConstants.setBoolean(ctx,resultId,response.isSuccessful());
PropertiesConstants.setInt(ctx,getCodeId(resultId),response.code());
PropertiesConstants.setString(ctx,getReasonId(resultId),response.message());
}
}
@Override
protected void onExecute(XsObject root, XsObject current, LogicletContext ctx, ExecuteWatcher watcher) {
ApiClient client = ctx.getObject(pid);
if (client == null){
String clientId = PropertiesConstants.transform(ctx,$kubeClientId,KubeApiClients.DEFAULT);
log("Api client is null,api client [{}] is using.",clientId);
try {
client = KubeApiClients.getClient(clientId,false);
}catch (Exception ex){
log("Can not get default api client.");
}
}
if (client != null) {
onExecute(client, root, current, ctx, watcher);
}
}
protected void onSuperExecute(ApiClient client,XsObject root, XsObject current, LogicletContext ctx, ExecuteWatcher watcher){
super.onExecute(root,current,ctx,watcher);
}
abstract protected void onExecute(ApiClient client,XsObject root, XsObject current, LogicletContext ctx, ExecuteWatcher watcher);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy