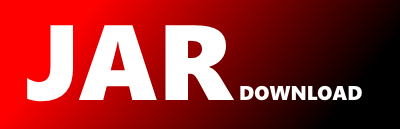
com.alogic.kube.xscript.api.KueListParams Maven / Gradle / Ivy
package com.alogic.kube.xscript.api;
import com.anysoft.util.Configurable;
import com.anysoft.util.Properties;
import com.anysoft.util.PropertiesConstants;
import io.kubernetes.client.openapi.ApiClient;
import io.kubernetes.client.openapi.Pair;
import org.apache.commons.lang3.StringUtils;
import java.util.List;
/**
* List参数
*
* @since 1.6.16.14
*/
public class KueListParams implements Configurable {
protected String pretty = null;
protected String allowWatchBookmarks = null;
protected String toContinue = null;
protected String fieldSelector = null;
protected String labelSelector = null;
protected String limit = null;
@Override
public void configure(Properties p) {
this.pretty = PropertiesConstants.getRaw(p,"pretty",pretty);
this.allowWatchBookmarks = PropertiesConstants.getRaw(p,"allowWatchBookmarks",allowWatchBookmarks);
this.toContinue = PropertiesConstants.getRaw(p,"continue",toContinue);
this.fieldSelector = PropertiesConstants.getRaw(p,"fieldSelector",fieldSelector);
this.labelSelector = PropertiesConstants.getRaw(p,"labelSelector",labelSelector);
this.limit = PropertiesConstants.getRaw(p,"limit",limit);
}
public String getPretty(Properties ctx){
return PropertiesConstants.transform(ctx,pretty,null);
}
public String getAllowWatchBookmarks(Properties ctx){
return PropertiesConstants.transform(ctx,allowWatchBookmarks,null);
}
public String getToContinue(Properties ctx){
return PropertiesConstants.transform(ctx,toContinue,null);
}
public String getFieldSelector(Properties ctx){
return PropertiesConstants.transform(ctx,fieldSelector,null);
}
public String getLabelSelector(Properties ctx){
return PropertiesConstants.transform(ctx,labelSelector,null);
}
public String getLimit(Properties ctx){
return PropertiesConstants.transform(ctx,limit,null);
}
public KueListParams setPretty(String pretty){
this.pretty = pretty;
return this;
}
public KueListParams setAllowWatchBookmarks(String allowWatchBookmarks){
this.allowWatchBookmarks = allowWatchBookmarks;
return this;
}
public KueListParams setToContinue(String toContinue){
this.toContinue = toContinue;
return this;
}
public KueListParams setLabelSelector(String labelSelector){
this.labelSelector = labelSelector;
return this;
}
public KueListParams setFieldSelector(String fieldSelector){
this.fieldSelector = fieldSelector;
return this;
}
public KueListParams setLimit(String limit){
this.limit = limit;
return this;
}
public List addParams(List list, ApiClient api,Properties ctx){
addParam(list,"pretty",getPretty(ctx));
addParam(list,"allowWatchBookmarks",getAllowWatchBookmarks(ctx));
addParam(list,"continue",getToContinue(ctx));
addParam(list,"fieldSelector",getFieldSelector(ctx));
addParam(list,"labelSelector",getLabelSelector(ctx));
addParam(list,"limit",getLimit(ctx));
return list;
}
private KueListParams addParam(List list,String key,String value){
if (StringUtils.isNotEmpty(value)){
list.add(new Pair(key,value));
}
return this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy