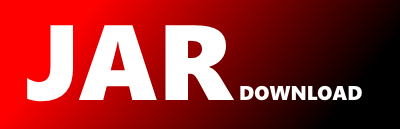
com.alogic.rabbitmq.producer.Fanout Maven / Gradle / Ivy
package com.alogic.rabbitmq.producer;
import java.io.IOException;
import java.util.concurrent.TimeoutException;
import org.w3c.dom.Element;
import com.alogic.pool.impl.Queued;
import com.alogic.rabbitmq.MQProducer;
import com.alogic.rabbitmq.MQServer;
import com.anysoft.util.BaseException;
import com.anysoft.util.Properties;
import com.anysoft.util.PropertiesConstants;
import com.anysoft.util.XmlElementProperties;
import com.rabbitmq.client.Channel;
import com.rabbitmq.client.Connection;
import com.rabbitmq.client.ConnectionFactory;
/**
* 消息发送者(广播模式)
* @author yyduan
*
*/
public class Fanout extends Queued implements MQProducer{
/**
* server
*/
protected MQServer server;
/**
* MQ连接
*/
protected Connection conn = null;
/**
* exchange
*/
protected String exchange = "default";
protected boolean durable = true;
protected boolean exclusive = false;
protected boolean autoDelete = false;
public Fanout(){
}
@Override
public void configure(Properties p) {
super.configure(p);
exchange = PropertiesConstants.getString(p,"exchange",exchange);
durable = PropertiesConstants.getBoolean(p,"durable",durable);
exclusive = PropertiesConstants.getBoolean(p,"exclusive",exclusive);
autoDelete = PropertiesConstants.getBoolean(p,"autoDelete",autoDelete);
}
@Override
public void close(){
super.close();
if (conn != null){
try {
conn.close();
} catch (IOException e) {
}
}
}
protected String getIdOfMaxQueueLength() {
return "maxActive";
}
protected String getIdOfIdleQueueLength() {
return "maxIdle";
}
@Override
public void configure(Element e, Properties p) {
Properties props = new XmlElementProperties(e,p);
configure(props);
}
@SuppressWarnings("unchecked")
@Override
protected pooled createObject() {
try {
if (conn == null || !conn.isOpen()){
ConnectionFactory cf = server.getConnectionFactory();
conn = cf.newConnection();
}
return (pooled) conn.createChannel();
}catch (IOException ex){
throw new BaseException("core.e1004","Can not create mq connection or channel");
} catch (TimeoutException e) {
throw new BaseException("core.e1011","Can not create mq connection or channel");
}
}
public void send(String q,byte[] data){
Channel channel = null;
boolean error = false;
try {
channel = this.borrowObject(0, 10000);
channel.exchangeDeclare(exchange, "fanout",durable,exclusive,autoDelete,null);
channel.basicPublish(exchange, "", null, data);
}catch (IOException ex){
error = true;
throw new BaseException("core.e1004","Failed to send data to mq.");
}finally{
if (channel != null){
returnObject(channel, error);
}
}
}
@Override
public void start(MQServer server) {
this.server = server;
}
@Override
public void stop(MQServer server) {
this.close();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy