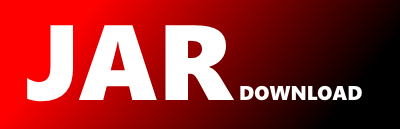
com.alogic.together2.xscript.QrCode Maven / Gradle / Ivy
package com.alogic.together2.xscript;
import java.awt.BasicStroke;
import java.awt.Graphics2D;
import java.awt.image.BufferedImage;
import java.io.OutputStream;
import java.io.UnsupportedEncodingException;
import java.net.URLEncoder;
import java.util.HashMap;
import java.util.Map;
import javax.imageio.ImageIO;
import org.apache.commons.lang3.StringUtils;
import com.alogic.xscript.AbstractLogiclet;
import com.alogic.xscript.ExecuteWatcher;
import com.alogic.xscript.Logiclet;
import com.alogic.xscript.LogicletContext;
import com.alogic.xscript.doc.XsObject;
import com.anysoft.util.BaseException;
import com.anysoft.util.Properties;
import com.anysoft.util.PropertiesConstants;
import com.anysoft.util.Settings;
import com.google.zxing.BarcodeFormat;
import com.google.zxing.EncodeHintType;
import com.google.zxing.common.BitMatrix;
import com.google.zxing.qrcode.QRCodeWriter;
import com.google.zxing.qrcode.decoder.ErrorCorrectionLevel;
import com.logicbus.backend.Context;
import com.logicbus.backend.server.http.HttpCacheTool;
/**
* 二维码插件
*
* @author yyduan
* @since 1.6.12.2
*/
public class QrCode extends AbstractLogiclet{
protected String pid = "$context";
protected String $content = "";
protected String $cacheEnable = "false";
protected String $filename = "";
protected String $contentType = "image/jpeg";
protected HttpCacheTool cacheTool = null;
protected String encoding = "utf-8";
protected String $width = "128";
protected String $height = "128";
protected String id;
protected Map hints = null;
/**
* 用于设置图案的颜色
*/
private static final int BLACK = 0xFF000000;
/**
* 用于背景色
*/
private static final int WHITE = 0xFFFFFFFF;
public QrCode(String tag, Logiclet p) {
super(tag, p);
}
@Override
public void configure(Properties p){
super.configure(p);
pid = PropertiesConstants.getString(p,"pid",pid,true);
$cacheEnable = PropertiesConstants.getRaw(p, "cacheEnable", $cacheEnable);
$width = PropertiesConstants.getRaw(p, "width", $width);
$height = PropertiesConstants.getRaw(p, "height", $height);
$filename = PropertiesConstants.getRaw(p, "filename", $filename);
$contentType = PropertiesConstants.getRaw(p, "contentType", $contentType);
$content = PropertiesConstants.getRaw(p, "content", $content);
cacheTool = Settings.get().getToolkit(HttpCacheTool.class);
encoding = PropertiesConstants.getString(p,"encoding",encoding);
id = PropertiesConstants.getString(p,"id","$" + getXmlTag(),true);
hints = new HashMap();
hints.put(EncodeHintType.ERROR_CORRECTION, ErrorCorrectionLevel.H);
hints.put(EncodeHintType.CHARACTER_SET, encoding);
hints.put(EncodeHintType.MARGIN, 1);
}
@Override
protected void onExecute(XsObject root, XsObject current,
final LogicletContext ctx, final ExecuteWatcher watcher) {
Context serviceContext = ctx.getObject(pid);
if (serviceContext == null) {
throw new BaseException("core.e1001",
"It must be in a DownloadTogetherServant servant,check your together script.");
}
if (PropertiesConstants.transform(ctx, $cacheEnable, true)) {
cacheTool.cacheEnable(serviceContext);
} else {
cacheTool.cacheDisable(serviceContext);
}
String filename = PropertiesConstants.transform(ctx, $filename, "");
if (StringUtils.isNotEmpty(filename)) {
try {
filename = URLEncoder.encode(filename, encoding);
} catch (UnsupportedEncodingException e) {
}
serviceContext.setResponseHeader("Content-Disposition", String
.format("attachment; filename=%s;filename*=%s''%s",
filename, encoding, filename));
}
String contentType = PropertiesConstants.transform(ctx, $contentType,
"");
if (StringUtils.isNotEmpty(contentType)) {
serviceContext.setResponseContentType(contentType);
}
String content = PropertiesConstants.transform(ctx, $content, "");
if (StringUtils.isEmpty(content)) {
throw new BaseException("core.e1003","The content of qrcode is null");
}
int width = PropertiesConstants.getInt(ctx, $width, 128);
int height = PropertiesConstants.getInt(ctx, $height, 128);
try {
OutputStream out = serviceContext.getOutputStream();
BitMatrix matrix = new QRCodeWriter().encode(content,
BarcodeFormat.QR_CODE, width, height, hints);
width = matrix.getWidth();
height = matrix.getHeight();
BufferedImage image = new BufferedImage(width, height,
BufferedImage.TYPE_INT_RGB);
for (int x = 0; x < width; x++) {
for (int y = 0; y < height; y++) {
image.setRGB(x, y, (matrix.get(x, y) ? BLACK : WHITE));
}
}
// 读取二维码图片,并构建绘图对象
Graphics2D g = image.createGraphics();
int matrixWidth = image.getWidth();
int matrixHeigh = image.getHeight();
// 开始绘制图片
g.drawImage(null, matrixWidth / 5 * 2, matrixHeigh / 5 * 2,
matrixWidth / 5, matrixHeigh / 5, null);
BasicStroke stroke = new BasicStroke(5, BasicStroke.CAP_ROUND,
BasicStroke.JOIN_ROUND);
g.setStroke(stroke);
g.dispose();
ImageIO.write(image, "JPEG", out);
out.flush();
ctx.SetValue(id, "true");
} catch (Exception ex) {
log("Error when writing data to outputstream:" + ex.getMessage(), LOG_ERROR,ctx);
ctx.SetValue(id, "false");
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy