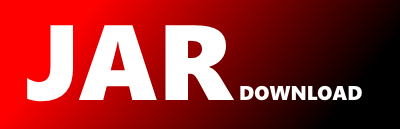
com.alogic.terminal.sshj.SSH Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of alogic-vfs Show documentation
Show all versions of alogic-vfs Show documentation
虚拟文件系统(Virutal File System)框架
package com.alogic.terminal.sshj;
import com.alogic.terminal.Command;
import com.alogic.terminal.Resolver;
import com.alogic.terminal.Terminal;
import com.anysoft.util.*;
import net.schmizz.sshj.connection.channel.direct.Session;
import net.schmizz.sshj.transport.verification.PromiscuousVerifier;
import java.io.*;
import net.schmizz.sshj.SSHClient;
import org.apache.commons.lang3.exception.ExceptionUtils;
public class SSH extends Terminal.Abstract{
/**
* 端口
*/
protected int port = 22;
/**
* 主机IP
*/
protected String host;
/**
* sshclient
*/
protected SSHClient sshClient = null;
/**
* timeout
*/
protected int timeout = 20000;
protected String encoding = "utf-8";
protected Authenticator authenticator = null;
@Override
public void configure(Properties p) {
host = PropertiesConstants.getString(p,"host",host,true);
port = PropertiesConstants.getInt(p,"port",port,true);
timeout = PropertiesConstants.getInt(p,"timeout",timeout,true);
encoding = PropertiesConstants.getString(p,"encoding",encoding,true);
String auth = PropertiesConstants.getString(p, "auth", "WithPassword");
Authenticator.TheFactory factory = new Authenticator.TheFactory();
try{
authenticator = factory.newInstance(auth,p);
}catch (Exception ex){
LOG.error("Can not create authenticator: {}",auth);
}
}
@Override
public void connect() {
try {
sshClient = new SSHClient();
sshClient.addHostKeyVerifier(new PromiscuousVerifier());
sshClient.connect(this.host,this.port);
if (authenticator != null){
authenticator.authenticate(sshClient);
}
} catch (Exception e) {
e.printStackTrace();
throw new BaseException("core.e1004","Can not connect to ssh server",e);
}
}
@Override
public void disconnect() {
if (sshClient != null){
try {
sshClient.disconnect();
}catch (Exception ex){
}
sshClient = null;
}
}
@Override
public int exec(Resolver resolver, String... cmd) {
Command simple = new Command.Simple(resolver,cmd);
return exec(simple);
}
@Override
public int exec(Command command) {
if (command.isShellMode()){
return execShell(command);
}
Session sshSession = null;
int ret = 0;
try {
sshSession = sshClient.startSession();
sshSession.allocateDefaultPTY();
Session.Command exec = sshSession.exec(command.getCommand());
resolveResult(command,command.getCommand(),exec.getInputStream());
ret = exec.getExitStatus();
} catch (Exception e) {
LOG.error(ExceptionUtils.getStackTrace(e));
throw new BaseException("core.e1004", "Error occurs:" + e.getMessage(), e);
} finally {
if (sshSession != null) {
IOTools.close(sshSession);
}
return ret;
}
}
protected int execShell(final Command command) {
Session sshSession = null;
PrintWriter writer = null;
try {
sshSession = sshClient.startSession();
sshSession.allocateDefaultPTY();
Session.Shell shell = sshSession.startShell();
writer = new PrintWriter(shell.getOutputStream(),true);
String[] cmds = command.getCommands();
for (final String cmd:cmds){
writer.println(cmd);
String random = KeyGen.uuid();
writer.println("echo " + random);
resolveResult(command,cmd,shell.getInputStream(),random);
}
return 0;
} catch (Exception e) {
LOG.error(ExceptionUtils.getStackTrace(e));
throw new BaseException("core.e1004", "Error occurs:" + e.getMessage(), e);
} finally {
if (sshSession != null) {
IOTools.close(sshSession);
}
}
}
protected void resolveResult(Command command, String cmd, InputStream in, String marker) throws IOException {
BufferedReader br = new BufferedReader(new InputStreamReader(in,encoding));
Object cookies = command.resolveBegin(cmd);
while (true)
{
String line = br.readLine();
if (line == null || line.equals(marker)){
break;
}
if (line.equals(cmd) || line.contains(marker)){
continue;
}
command.resolveLine(cookies, line);
}
command.resolveEnd(cookies);
}
protected void resolveResult(Command command,String cmd,InputStream in) throws IOException{
BufferedReader br = new BufferedReader(new InputStreamReader(in,encoding));
Object cookies = command.resolveBegin(cmd);
while (true)
{
String line = br.readLine();
if (line == null) {
break;
}
command.resolveLine(cookies, line);
}
command.resolveEnd(cookies);
}
@Override
public void close() {
disconnect();
}
private void waitForEcho(long timeout){
try {
Thread.sleep(timeout);
} catch (InterruptedException e) {
}
}
@Override
public boolean changePassword(String oldPwd,String newPwd,Resolver resolver) {
Session sshSession = null;
PrintWriter writer = null;
String cmd = "LANG=\"en_US.UTF-8\" && passwd";
Object cookies = resolver.resolveBegin(cmd);
try{
sshSession = sshClient.startSession();
sshSession.allocateDefaultPTY();
Session.Shell channel = sshSession.startShell();
InputStream in = channel.getInputStream();
InputStream err = channel.getErrorStream();
writer = new PrintWriter(channel.getOutputStream(),true);
byte[] buffer = new byte[500];
int length = 0;
length = in.read(buffer);
if (length > 0 && resolver != null){
resolver.resolveLine(cookies, new String(buffer, 0, length));
}
writer.println(cmd);
waitForEcho(500);
length = in.read(buffer);
if (length > 0 && resolver != null){
resolver.resolveLine(cookies, new String(buffer, 0, length));
}
writer.println(oldPwd);
waitForEcho(500);
length = in.read(buffer);
if (length > 0 && resolver != null){
resolver.resolveLine(cookies, new String(buffer, 0, length));
}
writer.println(newPwd);
waitForEcho(500);
length = in.read(buffer);
if (length > 0) {
String rs = new String(buffer, 0, length);
resolver.resolveLine(cookies, rs);
if(rs.indexOf("error") > -1){
return false;
}
}
writer.println(newPwd);
waitForEcho(1000);
length = in.read(buffer);
if (length > 0) {
String rs = new String(buffer, 0, length);
resolver.resolveLine(cookies, rs);
if(rs.indexOf("successfully") < 0){
return false;
}
}
} catch (Exception e) {
LOG.error(ExceptionUtils.getStackTrace(e));
throw new BaseException("core.e1004", "Error occurs:" + e.getMessage(), e);
}finally{
resolver.resolveEnd(cookies);
if (sshSession != null){
IOTools.close(sshSession);
}
}
return true;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy