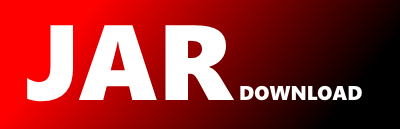
com.alogic.terminal.sshj.auth.WithPublicKey Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of alogic-vfs Show documentation
Show all versions of alogic-vfs Show documentation
虚拟文件系统(Virutal File System)框架
package com.alogic.terminal.sshj.auth;
import com.alogic.terminal.sshj.Authenticator;
import com.anysoft.util.*;
import com.anysoft.util.code.Coder;
import com.anysoft.util.code.CoderFactory;
import com.anysoft.util.resource.ResourceFactory;
import net.schmizz.sshj.SSHClient;
import net.schmizz.sshj.userauth.keyprovider.KeyProvider;
import net.schmizz.sshj.userauth.keyprovider.OpenSSHKeyFile;
import net.schmizz.sshj.userauth.password.PasswordFinder;
import net.schmizz.sshj.userauth.password.Resource;
import org.apache.commons.lang3.StringUtils;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.io.*;
/**
* 通过publicKey验证
*/
public class WithPublicKey implements Authenticator, Configurable {
private static final Logger LOG = LoggerFactory.getLogger(WithPublicKey.class);
private String username;
private String password;
private String keyContent;
private Coder coder = null;
private boolean isNullPassword(String pwd){
return StringUtils.isEmpty(password) || "none".equals(password);
}
@Override
public boolean authenticate(SSHClient conn) throws IOException {
if (StringUtils.isEmpty(keyContent)) {
conn.authPassword(username,password);
}else{
OpenSSHKeyFile kp = new OpenSSHKeyFile();
if (StringUtils.isNotEmpty(password)){
kp.init(new StringReader(keyContent), new PasswordFinder(){
@Override
public char[] reqPassword(Resource> resource) {
return password.toCharArray();
}
@Override
public boolean shouldRetry(Resource> resource) {
return false;
}
});
}else {
kp.init(new StringReader(keyContent));
}
conn.authPublickey(username,kp);
}
return true;
}
@Override
public void configure(Properties p) {
username = PropertiesConstants.getString(p,"username","");
password = PropertiesConstants.getString(p,"password","");
if (!isNullPassword(password)) {
try {
Coder coder = CoderFactory.newCoder(PropertiesConstants.getString(p, "coder", "DES3"));
password = coder.decode(password, username);
} catch (Exception ex) {
LOG.error("Can not find coder:" + coder);
}
}
keyContent = PropertiesConstants.getString(p,"keyContent","");
if (StringUtils.isEmpty(keyContent)){
String keyPath = PropertiesConstants.getString(p,"keyPath","");
keyContent = readKeyContent(keyPath,"");
}
}
protected static String readKeyContent(String keyPath,String dft) {
ResourceFactory rf = Settings.getResourceFactory();
InputStream in = null;
try {
in = rf.load(keyPath, null);
BufferedReader reader = new BufferedReader(new InputStreamReader(in));
StringBuffer content = new StringBuffer();
String line = null;
while ((line = reader.readLine()) != null) {
content.append(line).append("\n");
}
return content.toString();
}catch (Exception ex){
LOG.error("Can not read content from file: " + keyPath);
LOG.error(ex.getMessage());
return dft;
}finally{
IOTools.close(in);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy