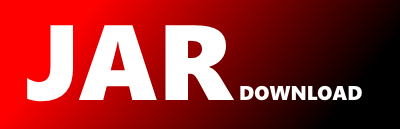
com.github.aoreshin.allure.rest.assured.ApiRequestSteps Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of allure-rest-assured Show documentation
Show all versions of allure-rest-assured Show documentation
Project to ease pain of test automation
package com.github.aoreshin.allure.rest.assured;
import static io.restassured.RestAssured.given;
import com.github.aoreshin.junit5.allure.steps.StepWrapperSteps;
import io.qameta.allure.Step;
import io.restassured.mapper.ObjectMapper;
import io.restassured.response.Response;
import io.restassured.specification.RequestSpecification;
import java.util.Collection;
import java.util.List;
import java.util.Map;
import java.util.concurrent.Callable;
import org.awaitility.Awaitility;
import org.hamcrest.Matcher;
/** Steps for building request and sending it */
public class ApiRequestSteps extends StepWrapperSteps {
private RequestSpecification requestSpecification = given();
private ApiRequestSteps() {}
public static ApiRequestSteps apiRequest() {
return new ApiRequestSteps();
}
@Step("Добавление заголовка {name}={value}")
public ApiRequestSteps header(String name, String value) {
requestSpecification.header(name, value);
return this;
}
@Step("Добавление заголовков {headers}")
public ApiRequestSteps headers(Map headers) {
requestSpecification.headers(headers);
return this;
}
@Step("Добавление cookie {cookieName}")
public ApiRequestSteps cookie(String cookieName) {
requestSpecification.cookie(cookieName);
return this;
}
@Step("Добавление cookie {cookieName}={value}, {additionalValues}")
public ApiRequestSteps cookie(String cookieName, Object value, Object... additionalValues) {
requestSpecification.cookie(cookieName, value, additionalValues);
return this;
}
@Step("Добавление cookies {cookies}")
public ApiRequestSteps cookies(Map cookies) {
requestSpecification.cookies(cookies);
return this;
}
@Step("Добавление cookie {firstCookieName}={firstCookieValue}, {cookieNameValuePairs}")
public ApiRequestSteps cookies(
String firstCookieName, Object firstCookieValue, Object... cookieNameValuePairs) {
requestSpecification.cookies(firstCookieName, firstCookieValue, cookieNameValuePairs);
return this;
}
@Step("Добавление параметра {name}={value}")
public ApiRequestSteps param(String name, String value) {
requestSpecification.param(name, value);
return this;
}
@Step("Добавление списка параметров {name}={paramList}")
public ApiRequestSteps param(String name, List paramList) {
requestSpecification.param(name, paramList);
return this;
}
@Step("Добавление мапы параметров {paramMap}")
public ApiRequestSteps params(Map paramMap) {
requestSpecification.params(paramMap);
return this;
}
@Step("Добавление query параметров {name}={parameterValues}")
public ApiRequestSteps queryParam(String name, Object... parameterValues) {
requestSpecification.queryParam(name, parameterValues);
return this;
}
@Step("Добавление query параметров {name}={parameterValues}")
public ApiRequestSteps queryParam(String name, Collection> parameterValues) {
requestSpecification.queryParam(name, parameterValues);
return this;
}
@Step("Добавление мапы query параметров {paramMap}")
public ApiRequestSteps queryParams(Map paramMap) {
requestSpecification.queryParams(paramMap);
return this;
}
@Step("Добавление form параметра {parameterName}={parameterValues}")
public ApiRequestSteps formParam(String parameterName, Object... parameterValues) {
requestSpecification.formParam(parameterName, parameterValues);
return this;
}
@Step("Добавление form параметра {parameterName}={parameterValues}")
public ApiRequestSteps formParam(String parameterName, Collection> parameterValues) {
requestSpecification.formParam(parameterName, parameterValues);
return this;
}
@Step("Добавление мапы form параметров {paramMap}")
public ApiRequestSteps formParams(Map paramMap) {
requestSpecification.formParams(paramMap);
return this;
}
@Step("Добавление тела запроса")
public ApiRequestSteps body(Object body) {
requestSpecification.body(body);
return this;
}
@Step("Обработка и добавление тела запроса с {objectMapper}")
public ApiRequestSteps body(Object body, ObjectMapper objectMapper) {
requestSpecification.body(body, objectMapper);
return this;
}
@Step("Отправка POST запроса на {url}")
public ApiValidationSteps post(String url) {
return new ApiValidationSteps(requestSpecification.post(url));
}
@Step("Отправка POST запроса на {url} пока не выполнится условие: {conditionMessage}")
public ApiValidationSteps post(String url, Matcher matcher, String conditionMessage) {
return getValidationSteps(() -> requestSpecification.post(url), matcher);
}
@Step("Отправка PUT запроса на {url}")
public ApiValidationSteps put(String url) {
return new ApiValidationSteps(requestSpecification.put(url));
}
@Step("Отправка PUT запроса на {url} пока не выполнится условие: {conditionMessage}")
public ApiValidationSteps put(String url, Matcher matcher, String conditionMessage) {
return getValidationSteps(() -> requestSpecification.put(url), matcher);
}
@Step("Отправка GET запроса на {url}")
public ApiValidationSteps get(String url) {
return new ApiValidationSteps(requestSpecification.get(url));
}
@Step("Отправка GET запроса на {url} пока не выполнится условие: {conditionMessage}")
public ApiValidationSteps get(String url, Matcher matcher, String conditionMessage) {
return getValidationSteps(() -> requestSpecification.get(url), matcher);
}
@Step("Отправка DELETE запроса на {url}")
public ApiValidationSteps delete(String url) {
return new ApiValidationSteps(requestSpecification.delete(url));
}
@Step("Отправка DELETE запроса на {url} пока не выполнится условие: {conditionMessage}")
public ApiValidationSteps delete(String url, Matcher matcher, String conditionMessage) {
return getValidationSteps(() -> requestSpecification.delete(url), matcher);
}
@Step("Отправка HEAD запроса на {url}")
public ApiValidationSteps head(String url) {
return new ApiValidationSteps(requestSpecification.head(url));
}
@Step("Отправка HEAD запроса на {url} пока не выполнится условие: {conditionMessage}")
public ApiValidationSteps head(String url, Matcher matcher, String conditionMessage) {
return getValidationSteps(() -> requestSpecification.head(url), matcher);
}
@Step("Отправка PATCH запроса на {url}")
public ApiValidationSteps patch(String url) {
return new ApiValidationSteps(requestSpecification.patch(url));
}
@Step("Отправка PATCH запроса на {url} пока не выполнится условие: {conditionMessage}")
public ApiValidationSteps patch(String url, Matcher matcher, String conditionMessage) {
return getValidationSteps(() -> requestSpecification.patch(url), matcher);
}
/** Only for testing */
void setRequestSpecification(RequestSpecification requestSpecification) {
this.requestSpecification = requestSpecification;
}
private ApiValidationSteps getValidationSteps(
Callable callable, Matcher matcher) {
Response response = Awaitility.given().until(callable, matcher);
return new ApiValidationSteps(response);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy