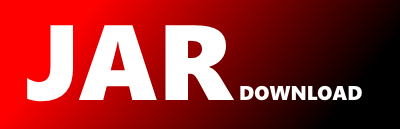
aquality.selenium.core.elements.Element Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aquality-selenium-core Show documentation
Show all versions of aquality-selenium-core Show documentation
Library with core functions simplifying work with Selenium-controlled applications.
package aquality.selenium.core.elements;
import aquality.selenium.core.applications.IApplication;
import aquality.selenium.core.configurations.IElementCacheConfiguration;
import aquality.selenium.core.configurations.ILoggerConfiguration;
import aquality.selenium.core.elements.interfaces.*;
import aquality.selenium.core.localization.ILocalizationManager;
import aquality.selenium.core.localization.ILocalizedLogger;
import aquality.selenium.core.logging.Logger;
import aquality.selenium.core.utilities.IElementActionRetrier;
import aquality.selenium.core.waitings.IConditionalWait;
import org.openqa.selenium.By;
import org.openqa.selenium.NoSuchElementException;
import org.openqa.selenium.WebDriverException;
import org.openqa.selenium.remote.RemoteWebElement;
import java.time.Duration;
import java.util.List;
import java.util.function.Supplier;
/**
* Abstract Element class.
*
* @author lenovo
* @version $Id: $Id
*/
public abstract class Element implements IElement {
private final String name;
private final ElementState elementState;
private final By locator;
private IElementCacheHandler elementCacheHandler;
/**
* Constructor for Element.
*
* @param loc a {@link org.openqa.selenium.By} object.
* @param name a {@link java.lang.String} object.
* @param state a {@link aquality.selenium.core.elements.ElementState} object.
*/
protected Element(final By loc, final String name, final ElementState state) {
locator = loc;
this.name = name;
elementState = state;
}
/**
* getApplication.
*
* @return a {@link aquality.selenium.core.applications.IApplication} object.
*/
protected abstract IApplication getApplication();
/**
* getElementFactory.
*
* @return a {@link aquality.selenium.core.elements.interfaces.IElementFactory} object.
*/
protected abstract IElementFactory getElementFactory();
/**
* getElementFinder.
*
* @return a {@link aquality.selenium.core.elements.interfaces.IElementFinder} object.
*/
protected abstract IElementFinder getElementFinder();
/**
* getElementCacheConfiguration.
*
* @return a {@link aquality.selenium.core.configurations.IElementCacheConfiguration} object.
*/
protected abstract IElementCacheConfiguration getElementCacheConfiguration();
/**
* getElementActionRetrier.
*
* @return a {@link aquality.selenium.core.utilities.IElementActionRetrier} object.
*/
protected abstract IElementActionRetrier getElementActionRetrier();
/**
* getLocalizedLogger.
*
* @return a {@link aquality.selenium.core.localization.ILocalizedLogger} object.
*/
protected abstract ILocalizedLogger getLocalizedLogger();
/**
* getLocalizationManager.
*
* @return a {@link aquality.selenium.core.localization.ILocalizationManager} object.
*/
protected abstract ILocalizationManager getLocalizationManager();
/**
* getConditionalWait.
*
* @return a {@link aquality.selenium.core.waitings.IConditionalWait} object.
*/
protected abstract IConditionalWait getConditionalWait();
/**
* getElementType.
*
* @return a {@link java.lang.String} object.
*/
protected abstract String getElementType();
/**
* getCache.
*
* @return a {@link aquality.selenium.core.elements.interfaces.IElementCacheHandler} object.
*/
protected IElementCacheHandler getCache() {
if (elementCacheHandler == null) {
elementCacheHandler = new ElementCacheHandler(locator, elementState, getElementFinder());
}
return elementCacheHandler;
}
/**
* getLoggerConfiguration.
*
* @return a {@link aquality.selenium.core.configurations.ILoggerConfiguration} object.
*/
protected ILoggerConfiguration getLoggerConfiguration() {
return getLocalizedLogger().getConfiguration();
}
/**
* getLogger.
*
* @return a {@link aquality.selenium.core.logging.Logger} object.
*/
protected Logger getLogger() {
return Logger.getInstance();
}
/**
* logElementState.
*
* @return a {@link aquality.selenium.core.elements.interfaces.ILogElementState} object.
*/
protected ILogElementState logElementState() {
return ((messageKey, stateKey) -> getLocalizedLogger().infoElementAction(getElementType(), getName(), messageKey,
getLocalizationManager().getLocalizedMessage(stateKey)));
}
/** {@inheritDoc} */
@Override
public By getLocator() {
return locator;
}
/** {@inheritDoc} */
@Override
public String getName() {
return name;
}
/** {@inheritDoc} */
@Override
public IElementStateProvider state() {
return getElementCacheConfiguration().isEnabled()
? new CachedElementStateProvider(locator, getConditionalWait(), getCache(), logElementState())
: new DefaultElementStateProvider(locator, getConditionalWait(), getElementFinder(), logElementState());
}
/** {@inheritDoc} */
@Override
public RemoteWebElement getElement(Duration timeout) {
try {
return getElementCacheConfiguration().isEnabled()
? getCache().getElement(timeout)
: (RemoteWebElement) getElementFinder().findElement(locator, elementState, timeout);
} catch (NoSuchElementException e) {
if (getLoggerConfiguration().logPageSource()) {
logPageSource(e);
}
throw e;
}
}
/**
* logPageSource.
*
* @param exception a {@link org.openqa.selenium.WebDriverException} object.
*/
protected void logPageSource(WebDriverException exception) {
try {
getLogger().debug("Page source:".concat(System.lineSeparator()).concat(getApplication().getDriver().getPageSource()), exception);
} catch (WebDriverException e) {
getLogger().error(exception.getMessage());
getLocalizedLogger().fatal("loc.get.page.source.failed", e);
}
}
/** {@inheritDoc} */
@Override
public String getText() {
logElementAction("loc.get.text");
String value = doWithRetry(() -> getElement().getText());
logElementAction("loc.text.value", value);
return value;
}
/** {@inheritDoc} */
@Override
public String getAttribute(String attr) {
logElementAction("loc.el.getattr", attr);
String value = doWithRetry(() -> getElement().getAttribute(attr));
logElementAction("loc.el.attr.value", attr, value);
return value;
}
/** {@inheritDoc} */
@Override
public void sendKeys(String keys) {
logElementAction("loc.text.sending.keys", keys);
doWithRetry(() -> getElement().sendKeys(keys));
}
/** {@inheritDoc} */
@Override
public void click() {
logElementAction("loc.clicking");
doWithRetry(() -> getElement().click());
}
/** {@inheritDoc} */
@Override
public T findChildElement(By childLoc, String name, Class clazz, ElementState state) {
return getElementFactory().findChildElement(this, childLoc, name, clazz, state);
}
/** {@inheritDoc} */
@Override
public T findChildElement(By childLoc, String name, IElementSupplier supplier, ElementState state) {
return getElementFactory().findChildElement(this, childLoc, name, supplier, state);
}
/** {@inheritDoc} */
@Override
public List findChildElements(By childLoc, String name, Class clazz, ElementState state, ElementsCount count) {
return getElementFactory().findChildElements(this, childLoc, name, clazz, count, state);
}
/** {@inheritDoc} */
@Override
public List findChildElements(By childLoc, String name, IElementSupplier supplier, ElementState state, ElementsCount count) {
return getElementFactory().findChildElements(this, childLoc, name, supplier, count, state);
}
/**
* doWithRetry.
*
* @param action a {@link java.util.function.Supplier} object.
* @param a T object.
* @return a T object.
*/
protected T doWithRetry(Supplier action) {
return getElementActionRetrier().doWithRetry(action);
}
/**
* doWithRetry.
*
* @param action a {@link java.lang.Runnable} object.
*/
protected void doWithRetry(Runnable action) {
getElementActionRetrier().doWithRetry(action);
}
/**
* logElementAction.
*
* @param messageKey a {@link java.lang.String} object.
* @param args a {@link java.lang.Object} object.
*/
protected void logElementAction(String messageKey, Object... args) {
getLocalizedLogger().infoElementAction(getElementType(), name, messageKey, args);
}
/**
* Getter for the field elementState
.
*
* @return a {@link aquality.selenium.core.elements.ElementState} object.
*/
protected ElementState getElementState() {
return elementState;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy