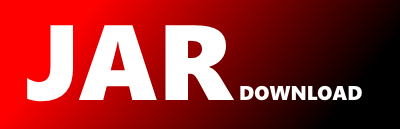
aquality.selenium.elements.ComboBox Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aquality-selenium Show documentation
Show all versions of aquality-selenium Show documentation
Library around Selenium WebDriver
package aquality.selenium.elements;
import aquality.selenium.core.elements.ElementState;
import aquality.selenium.elements.actions.ComboBoxJsActions;
import aquality.selenium.elements.interfaces.IComboBox;
import org.openqa.selenium.By;
import org.openqa.selenium.InvalidElementStateException;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.support.ui.Select;
import java.util.List;
import java.util.function.BiConsumer;
import java.util.function.Function;
import java.util.stream.Collectors;
/**
* Class describing the ComboBox (dropdown list)
*/
public class ComboBox extends Element implements IComboBox {
private static final String LOG_SELECTING_VALUE = "loc.selecting.value";
protected ComboBox(final By locator, final String name, final ElementState state) {
super(locator, name, state);
}
protected String getElementType() {
return getLocalizationManager().getLocalizedMessage("loc.combobox");
}
@Override
public void selectByIndex(int index) {
logElementAction(LOG_SELECTING_VALUE, String.format("#%s", index));
doWithRetry(() -> new Select(getElement()).selectByIndex(index));
}
@Override
public void selectByText(String value) {
logElementAction("loc.combobox.select.by.text", value);
doWithRetry(() -> new Select(getElement()).selectByVisibleText(value));
}
@Override
public void clickAndSelectByText(String value) {
click();
selectByText(value);
}
@Override
public void selectByContainingText(String text) {
logElementAction("loc.combobox.select.by.text", text);
applyFunctionToOptionsThatContain(WebElement::getText,
Select::selectByVisibleText,
text);
}
@Override
public void selectByContainingValue(String value) {
logElementAction(LOG_SELECTING_VALUE, value);
applyFunctionToOptionsThatContain(element -> element.getAttribute(Attributes.VALUE.toString()),
Select::selectByValue,
value);
}
protected void applyFunctionToOptionsThatContain(Function getValueFunc, BiConsumer
© 2015 - 2024 Weber Informatics LLC | Privacy Policy