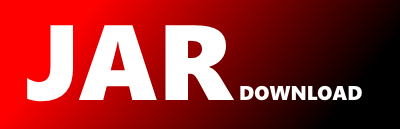
com.asher_stern.crf.utilities.MiscellaneousUtilities Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of CRF Show documentation
Show all versions of CRF Show documentation
Implementation of linear-chain Conditional Random Fields (CRF) in pure Java
The newest version!
package com.asher_stern.crf.utilities;
import java.util.ArrayList;
import java.util.Comparator;
import java.util.LinkedHashMap;
import java.util.LinkedHashSet;
import java.util.List;
import java.util.Map;
import java.util.Map.Entry;
import java.util.Random;
import java.util.Set;
/**
* A collection of static helper functions for pos-tagger.
*
* @author Asher Stern
* Date: Nov 10, 2014
*
*/
public class MiscellaneousUtilities
{
public static final long RANDOM_SELECTION_RANDOM_SEED = 101112L; // Just a number. Make it deterministic.
/**
* Finds all the tags that exist in the given corpus.
* @param corpus A corpus of POS-tagged sentences.
* @return All the tags in the given corpus.
*/
public static Set extractAllTagsFromCorpus(Iterable extends List extends TaggedToken>> corpus)
{
Set allTags = new LinkedHashSet();
for (List extends TaggedToken > sentence : corpus)
{
for (TaggedToken taggedToken : sentence)
{
allTags.add(taggedToken.getTag());
}
}
return allTags;
}
/**
* Tests whether the given two objects are equal. Null objects are permitted here.
* @param t1
* @param t2
* @return
*/
public static boolean equalObjects(T t1, T t2)
{
if (t1==t2) return true;
if ( (t1==null) || (t2==null) ) return false;
return t1.equals(t2);
}
/**
* Selects equally-distributed numbers from the given range.
*
* @param howMany
* @param size specifies that the range of selection is 0..size (not including size
)
* @return
*/
public static LinkedHashSet selectRandomlyFromRange(int howMany, int size)
{
Random random = new Random(RANDOM_SELECTION_RANDOM_SEED);
Map replacements = new LinkedHashMap<>();
LinkedHashSet ret = new LinkedHashSet<>();
for (int counter = 0; counter List selectRandomlyFromList(int howMany, List list)
{
if (list.size()"+list.size());
Set selectedIndexes = selectRandomlyFromRange(howMany, list.size());
List ret = new ArrayList<>(howMany);
int index=0;
for (E element : list)
{
if (selectedIndexes.contains(index))
{
ret.add(element);
}
++index;
}
return ret;
}
/**
* Creates and returns a new map from Integer to V, where the keys are the position of the items in the list.
* For example, for a List {"abc", "def", "ghi"}, the returned map would be:
* {1 --> "abc"}, {2 --> "def"}, {3 --> "ghi"}
* @param list A list
* @return A map whose values are the list items, and the keys are their positions (indexes) in the list.
*/
public static Map listToMap(List list)
{
Map map = new LinkedHashMap();
int index=0;
for (V v : list)
{
map.put(index, v);
++index;
}
return map;
}
/**
* Creates and returns a list of the map's keys, sorted by their values, according to their natural ordering.
*/
public static > List sortByValue(Map map)
{
return sortByValue(map, new NaturalComparator());
}
/**
* Creates and returns a list of the map's keys, sorted by their values, according to the given comparator.
*
* @param map A map.
* @param valueComparator Defines ordering relation of the map's values
* @return List of the map's keys, sorted by their values.
*/
public static List sortByValue(Map map, Comparator valueComparator)
{
List> list = new ArrayList>(map.entrySet().size());
for (Map.Entry entry : map.entrySet())
{
list.add(entry);
}
Comparator> comparator = new Comparator>()
{
@Override
public int compare(Entry o1, Entry o2)
{
if (o1.getValue()==o2.getValue()) return 0;
if (o1.getValue()==null) return -1;
if (o2.getValue()==null) return 1;
return valueComparator.compare(o1.getValue(), o2.getValue());
}
};
list.sort(comparator);
List ret = new ArrayList(list.size());
for (Map.Entry entry : list)
{
ret.add(entry.getKey());
}
return ret;
}
/**
* A comparator which compares to objects according to their natural ordering.
*
* @param
*/
private static class NaturalComparator> implements Comparator
{
@Override
public int compare(T o1, T o2)
{
return o1.compareTo(o2);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy