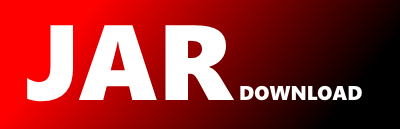
com.github.asteraether.tomlib.sqlib.PreparedStatementConnection Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of TomLib Show documentation
Show all versions of TomLib Show documentation
A simple collection of usefull things in java.
The newest version!
/*
* To change this license header, choose License Headers in Project Properties.
* To change this template file, choose Tools | Templates
* and open the template in the editor.
*/
package com.github.asteraether.tomlib.sqlib;
import com.github.asteraether.tomlib.sqlib.exceptions.ArrayNotSameSizeException;
import com.github.asteraether.tomlib.sqlib.exceptions.SQLNoConnectionException;
import com.github.asteraether.tomlib.sqlib.exceptions.SQLPreparedQueryNotDefinedException;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.util.LinkedHashMap;
import java.util.List;
import java.util.Map;
import java.util.Map.Entry;
import java.util.Set;
/**
*
* @author Thomas
* @param
*/
public class PreparedStatementConnection {
/**
*
*/
protected final Map statements;
/**
*
*/
protected Connection connection;
/**
*
* @param conn
* @param queryStatements
*/
public PreparedStatementConnection(Connection conn, List queryStatements) {
this.connection = conn;
statements = new LinkedHashMap<>();
queryStatements.forEach((T s) -> statements.put(s, null));
}
/**
*
* @param conn
* @param queryStatements
*/
public PreparedStatementConnection(Connection conn, T... queryStatements) {
this.connection = conn;
statements = new LinkedHashMap<>();
for (T queryStatement : queryStatements) {
statements.put(queryStatement, null);
}
}
/**
*
* @param key
* @return
* @throws SQLNoConnectionException
* @throws SQLPreparedQueryNotDefinedException
* @throws SQLException
*/
public PreparedStatement getPreparedStatement(T key) throws SQLNoConnectionException, SQLPreparedQueryNotDefinedException, SQLException {
if (connection == null || connection.isClosed()) {
throw new SQLNoConnectionException("No connection present");
}
if (statements.containsKey(key) && statements.get(key) == null) {
PreparedStatement s = connection.prepareStatement(key.getSQL());
statements.put(key, s);
return s;
} else if (statements.containsKey(key)) {
return statements.get(key);
} else {
throw new SQLPreparedQueryNotDefinedException(key + " was not defined in this Connection");
}
}
/**
*
* @param connection
* @throws SQLException
*/
public void setConnection(Connection connection) throws SQLException {
if (connection != null) {
closeStatements();
}
this.connection = connection;
}
/**
*
* @param key
* @return
* @throws SQLNoConnectionException
* @throws SQLPreparedQueryNotDefinedException
* @throws SQLException
*/
public ResultSet executeQuery(T key) throws SQLNoConnectionException, SQLPreparedQueryNotDefinedException, SQLException {
return getPreparedStatement(key).executeQuery();
}
/**
*
* @param key
* @return
* @throws SQLNoConnectionException
* @throws SQLPreparedQueryNotDefinedException
* @throws SQLException
*/
public int executeUpdate(T key) throws SQLNoConnectionException, SQLPreparedQueryNotDefinedException, SQLException {
return getPreparedStatement(key).executeUpdate();
}
/**
*
* @param key
* @param index
* @param values
* @return
* @throws SQLNoConnectionException
* @throws SQLPreparedQueryNotDefinedException
* @throws SQLException
*/
public int executeUpdate(T key, int[] index, Object[] values) throws SQLNoConnectionException, SQLPreparedQueryNotDefinedException, SQLException {
if (index.length != values.length) {
throw new ArrayNotSameSizeException("The given arrays are not the same size, but must be: index[]:" + index.length + "; values[]:" + values.length);
}
PreparedStatement p = getPreparedStatement(key);
for (int i = 0; i < index.length; i++) {
p.setObject(index[i], values[i]);
}
int changed = p.executeUpdate();
p.clearParameters();
return changed;
}
/**
*
* @param key
* @param values
* @return
* @throws SQLNoConnectionException
* @throws SQLPreparedQueryNotDefinedException
* @throws SQLException
*/
public int executeUpdate(T key, Object... values) throws SQLNoConnectionException, SQLPreparedQueryNotDefinedException, SQLException {
PreparedStatement p = getPreparedStatement(key);
for (int i = 1; i <= values.length; i++) {
p.setObject(i, values[i - 1]);
}
int changed = p.executeUpdate();
p.clearParameters();
return changed;
}
/**
*
* @param key
* @param values
* @return
* @throws SQLNoConnectionException
* @throws SQLPreparedQueryNotDefinedException
* @throws SQLException
*/
public ResultSet executeQuery(T key, Map values) throws SQLNoConnectionException, SQLPreparedQueryNotDefinedException, SQLException {
PreparedStatement p = getPreparedStatement(key);
for (Map.Entry e : values.entrySet()) {
p.setObject(e.getKey(), e.getValue());
}
ResultSet set = p.executeQuery();
p.clearParameters();
return set;
}
/**
*
* @param key
* @param index
* @param values
* @return
* @throws SQLNoConnectionException
* @throws SQLPreparedQueryNotDefinedException
* @throws SQLException
*/
public ResultSet executeQuery(T key, int[] index, Object[] values) throws SQLNoConnectionException, SQLPreparedQueryNotDefinedException, SQLException {
if (index.length != values.length) {
throw new ArrayNotSameSizeException("The given arrays are not the same size, but must be: index[]:" + index.length + "; values[]:" + values.length);
}
PreparedStatement p = getPreparedStatement(key);
for (int i = 0; i < index.length; i++) {
p.setObject(index[i], values[i]);
}
ResultSet set = p.executeQuery();
p.clearParameters();
return set;
}
/**
*
* @param key
* @param values
* @return
* @throws SQLNoConnectionException
* @throws SQLPreparedQueryNotDefinedException
* @throws SQLException
*/
public ResultSet executeQuery(T key, Object... values) throws SQLNoConnectionException, SQLPreparedQueryNotDefinedException, SQLException {
PreparedStatement p = getPreparedStatement(key);
for (int i = 1; i <= values.length; i++) {
p.setObject(i, values[i - 1]);
}
ResultSet set = p.executeQuery();
p.clearParameters();
return set;
}
/**
*
* @param key
* @return
* @throws SQLNoConnectionException
* @throws SQLPreparedQueryNotDefinedException
* @throws SQLException
*/
public boolean execute(T key) throws SQLNoConnectionException, SQLPreparedQueryNotDefinedException, SQLException {
return getPreparedStatement(key).execute();
}
/**
*
* @return
*/
public Set getStatementKeySet() {
return statements.keySet();
}
/**
*
* @throws SQLException
*/
public void closeStatements() throws SQLException {
for (Entry e : statements.entrySet()) {
if (e.getValue() != null) {
e.getValue().close();
statements.put(e.getKey(), null);
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy