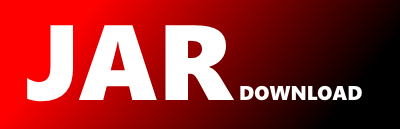
com.github.asteraether.tomlib.sqlib.SQLCachedStatementDatabase Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of TomLib Show documentation
Show all versions of TomLib Show documentation
A simple collection of usefull things in java.
The newest version!
/*
* To change this license header, choose License Headers in Project Properties.
* To change this template file, choose Tools | Templates
* and open the template in the editor.
*/
package com.github.asteraether.tomlib.sqlib;
import com.github.asteraether.tomlib.sqlib.exceptions.PropertyNotFoundException;
import com.github.asteraether.tomlib.sqlib.exceptions.SQLConnectionPresentException;
import com.github.asteraether.tomlib.sqlib.exceptions.SQLNoConnectionException;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.sql.Statement;
/**
*
* @author Thomas
*/
public class SQLCachedStatementDatabase extends SQLDatabase {
private CachedStatementConnection cachConn;
/**
*
* @param driverClass
* @param driver
* @throws ClassNotFoundException
*/
public SQLCachedStatementDatabase(String driverClass, String driver) throws ClassNotFoundException {
super(driverClass, driver);
}
/**
*
* @param prop
* @throws ClassNotFoundException
*/
public SQLCachedStatementDatabase(SQLDatabaseProperties prop) throws ClassNotFoundException {
super(prop);
}
/**
*
* @throws SQLException
*/
@Override
public void disconnect() throws SQLException {
super.disconnect();
if (cachConn != null) {
cachConn.closeStatements();
cachConn = null;
}
}
/**
*
* @throws SQLException
* @throws PropertyNotFoundException
* @throws SQLConnectionPresentException
*/
@Override
public void connect() throws SQLException, PropertyNotFoundException, SQLConnectionPresentException {
super.connect();
cachConn = new CachedStatementConnection(conn);
}
/**
*
* @param prop
* @throws SQLException
* @throws PropertyNotFoundException
* @throws SQLConnectionPresentException
*/
@Override
public void connect(SQLDatabaseProperties prop) throws SQLException, PropertyNotFoundException, SQLConnectionPresentException {
super.connect(prop);
cachConn = new CachedStatementConnection(conn);
}
/**
*
* @param host
* @param username
* @param password
* @throws SQLException
* @throws SQLConnectionPresentException
*/
@Override
public void connect(String host, String username, String password) throws SQLException, SQLConnectionPresentException {
super.connect(host, username, password);
cachConn = new CachedStatementConnection(conn);
}
/**
*
* @param hostname
* @param dbName
* @param username
* @param password
* @throws SQLException
* @throws SQLConnectionPresentException
*/
@Override
public void connect(String hostname, String dbName, String username, String password) throws SQLException, SQLConnectionPresentException {
super.connect(hostname, dbName, username, password);
cachConn = new CachedStatementConnection(conn);
}
/**
*
* @return @throws SQLNoConnectionException
* @throws SQLException
*/
public Statement getStatement() throws SQLNoConnectionException, SQLException {
if (cachConn == null || conn.isClosed()) {
throw new SQLNoConnectionException("No connection present");
}
return cachConn.getStatement();
}
/**
*
* @param stat
* @throws SQLNoConnectionException
* @throws SQLException
*/
public void releaseStatement(Statement stat) throws SQLNoConnectionException, SQLException {
if (cachConn == null || conn.isClosed()) {
throw new SQLNoConnectionException("No connection present");
}
cachConn.releaseStatement(stat);
}
/**
*
* @param key
* @return
* @throws SQLException
*/
@Override
public boolean execute(ISQLKey key) throws SQLException {
Statement s = getStatement();
boolean b = s.execute(key.getSQL());
releaseStatement(s);
return b;
}
/**
*
* @param key
* @return
* @throws SQLException
*/
@Override
public ResultSet executeQuery(ISQLKey key) throws SQLException {
Statement s = getStatement();
ResultSet b = s.executeQuery(key.getSQL());
releaseStatement(s);
return b;
}
/**
*
* @param key
* @return
* @throws SQLException
*/
@Override
public int executeUpdate(ISQLKey key) throws SQLException {
Statement s = getStatement();
int b = s.executeUpdate(key.getSQL());
releaseStatement(s);
return b;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy