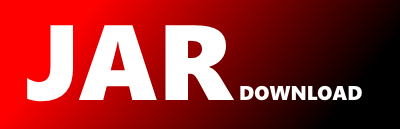
com.github.asteraether.tomlib.sqlib.SQLCallableStatementDatabase Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of TomLib Show documentation
Show all versions of TomLib Show documentation
A simple collection of usefull things in java.
The newest version!
/*
* To change this license header, choose License Headers in Project Properties.
* To change this template file, choose Tools | Templates
* and open the template in the editor.
*/
package com.github.asteraether.tomlib.sqlib;
import com.github.asteraether.tomlib.sqlib.exceptions.PropertyNotFoundException;
import com.github.asteraether.tomlib.sqlib.exceptions.SQLConnectionPresentException;
import com.github.asteraether.tomlib.sqlib.exceptions.SQLNoConnectionException;
import com.github.asteraether.tomlib.sqlib.exceptions.SQLPreparedQueryNotDefinedException;
import java.sql.CallableStatement;
import java.sql.Connection;
import java.sql.SQLException;
import java.util.LinkedList;
import java.util.List;
import java.util.Map;
import java.util.Set;
/**
*
* @author neumann6
* @param
*/
public class SQLCallableStatementDatabase extends SQLDatabase {
private final CallableStatementConnection callConn;
/**
*
* @param driverClass
* @param driver
* @param queryStatements
* @throws ClassNotFoundException
*/
public SQLCallableStatementDatabase(String driverClass, String driver, List queryStatements) throws ClassNotFoundException {
super(driverClass, driver);
List l = new LinkedList<>();
queryStatements.stream().forEach((s) -> l.add(s));
callConn = new CallableStatementConnection<>(null, l);
}
/**
*
* @param prop
* @param queryStatements
* @throws ClassNotFoundException
*/
public SQLCallableStatementDatabase(SQLDatabaseProperties prop, List queryStatements) throws ClassNotFoundException {
super(prop);
List l = new LinkedList<>();
queryStatements.stream().forEach((s) -> l.add(s));
callConn = new CallableStatementConnection<>(null, l);
}
/**
*
* @param driverClass
* @param driver
* @param queryStatements
* @throws ClassNotFoundException
*/
public SQLCallableStatementDatabase(String driverClass, String driver, T... queryStatements) throws ClassNotFoundException {
super(driverClass, driver);
callConn = new CallableStatementConnection<>(null, queryStatements);
}
/**
*
* @param prop
* @param queryStatements
* @throws ClassNotFoundException
*/
public SQLCallableStatementDatabase(SQLDatabaseProperties prop, T... queryStatements) throws ClassNotFoundException {
super(prop);
callConn = new CallableStatementConnection<>(null, queryStatements);
}
/**
*
* @param key
* @return
* @throws SQLNoConnectionException
* @throws SQLPreparedQueryNotDefinedException
* @throws SQLException
*/
public CallableStatement getCallableStatement(ISQLKey key) throws SQLNoConnectionException, SQLPreparedQueryNotDefinedException, SQLException {
return callConn.getCallableStatement(key);
}
/**
*
* @param connection
* @throws SQLException
*/
public void setConnection(Connection connection) throws SQLException {
callConn.setConnection(connection);
}
/**
*
* @param key
* @param outType
* @param params
* @return
* @throws SQLNoConnectionException
* @throws SQLPreparedQueryNotDefinedException
* @throws SQLException
*/
public Object executeCall(ISQLKey key, int outType, Object... params) throws SQLNoConnectionException, SQLPreparedQueryNotDefinedException, SQLException {
return callConn.executeCall(key, outType, params);
}
/**
*
* @param key
* @param outputtype
* @param params
* @return
* @throws SQLNoConnectionException
* @throws SQLPreparedQueryNotDefinedException
* @throws SQLException
*/
public Object executeTypeSpecificCall(ISQLKey key, int outputtype, Map.Entry... params) throws SQLNoConnectionException, SQLPreparedQueryNotDefinedException, SQLException {
return callConn.executeTypeSpecificCall(key, outputtype, params);
}
/**
*
* @return
*/
public Set getStatementKeySet() {
return callConn.getStatementKeySet();
}
/**
*
* @throws SQLException
*/
@Override
public void disconnect() throws SQLException {
callConn.closeStatements();
callConn.setConnection(null);
super.disconnect();
}
/**
*
* @throws SQLException
* @throws PropertyNotFoundException
* @throws SQLConnectionPresentException
*/
@Override
public void connect() throws SQLException, PropertyNotFoundException, SQLConnectionPresentException {
super.connect();
callConn.setConnection(getConnection());
}
/**
*
* @param prop
* @throws SQLException
* @throws PropertyNotFoundException
* @throws SQLConnectionPresentException
*/
@Override
public void connect(SQLDatabaseProperties prop) throws SQLException, PropertyNotFoundException, SQLConnectionPresentException {
super.connect(prop);
callConn.setConnection(getConnection());
}
/**
*
* @param host
* @param username
* @param password
* @throws SQLException
* @throws SQLConnectionPresentException
*/
@Override
public void connect(String host, String username, String password) throws SQLException, SQLConnectionPresentException {
super.connect(host, username, password);
callConn.setConnection(getConnection());
}
/**
*
* @param hostname
* @param dbName
* @param username
* @param password
* @throws SQLException
* @throws SQLConnectionPresentException
*/
@Override
public void connect(String hostname, String dbName, String username, String password) throws SQLException, SQLConnectionPresentException {
super.connect(hostname, dbName, username, password);
callConn.setConnection(getConnection());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy