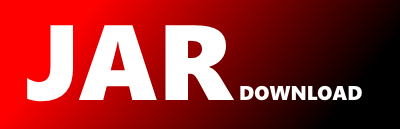
com.github.asteraether.tomlib.sqlib.SQLPreparedStatementDatabase Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of TomLib Show documentation
Show all versions of TomLib Show documentation
A simple collection of usefull things in java.
The newest version!
/*
* To change this license header, choose License Headers in Project Properties.
* To change this template file, choose Tools | Templates
* and open the template in the editor.
*/
package com.github.asteraether.tomlib.sqlib;
import com.github.asteraether.tomlib.sqlib.exceptions.PropertyNotFoundException;
import com.github.asteraether.tomlib.sqlib.exceptions.SQLConnectionPresentException;
import com.github.asteraether.tomlib.sqlib.exceptions.SQLNoConnectionException;
import com.github.asteraether.tomlib.sqlib.exceptions.SQLPreparedQueryNotDefinedException;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.util.LinkedList;
import java.util.List;
import java.util.Map;
import java.util.Set;
/**
*
* @author Thomas
* @param
*/
public class SQLPreparedStatementDatabase extends SQLDatabase {
private final PreparedStatementConnection prepConn;
/**
*
* @param driverClass
* @param driver
* @param queryStatements
* @throws ClassNotFoundException
*/
public SQLPreparedStatementDatabase(String driverClass, String driver, List queryStatements) throws ClassNotFoundException {
super(driverClass, driver);
List l = new LinkedList<>();
queryStatements.stream().forEach((s) -> l.add(s));
prepConn = new PreparedStatementConnection<>(null, l);
}
/**
*
* @param prop
* @param queryStatements
* @throws ClassNotFoundException
*/
public SQLPreparedStatementDatabase(SQLDatabaseProperties prop, List queryStatements) throws ClassNotFoundException {
super(prop);
List l = new LinkedList<>();
queryStatements.stream().forEach((s) -> l.add(s));
prepConn = new PreparedStatementConnection<>(null, l);
}
/**
*
* @param driverClass
* @param driver
* @param queryStatements
* @throws ClassNotFoundException
*/
public SQLPreparedStatementDatabase(String driverClass, String driver, T... queryStatements) throws ClassNotFoundException {
super(driverClass, driver);
prepConn = new PreparedStatementConnection<>(null, queryStatements);
}
/**
*
* @param prop
* @param queryStatements
* @throws ClassNotFoundException
*/
public SQLPreparedStatementDatabase(SQLDatabaseProperties prop, T... queryStatements) throws ClassNotFoundException {
super(prop);
prepConn = new PreparedStatementConnection<>(null, queryStatements);
}
/**
*
* @param key
* @return
* @throws SQLNoConnectionException
* @throws SQLPreparedQueryNotDefinedException
* @throws SQLException
*/
public PreparedStatement getPreparedStatement(ISQLKey key) throws SQLNoConnectionException, SQLPreparedQueryNotDefinedException, SQLException {
return prepConn.getPreparedStatement(key);
}
/**
*
* @param key
* @return
* @throws SQLNoConnectionException
* @throws SQLPreparedQueryNotDefinedException
* @throws SQLException
*/
public ResultSet executeQuery(ISQLKey key) throws SQLNoConnectionException, SQLPreparedQueryNotDefinedException, SQLException {
return prepConn.executeQuery(key);
}
/**
*
* @param key
* @return
* @throws SQLNoConnectionException
* @throws SQLPreparedQueryNotDefinedException
* @throws SQLException
*/
public int executeUpdate(ISQLKey key) throws SQLNoConnectionException, SQLPreparedQueryNotDefinedException, SQLException {
return prepConn.executeUpdate(key);
}
/**
*
* @param key
* @param index
* @param values
* @return
* @throws SQLNoConnectionException
* @throws SQLPreparedQueryNotDefinedException
* @throws SQLException
*/
public int executeUpdate(ISQLKey key, int[] index, Object[] values) throws SQLNoConnectionException, SQLPreparedQueryNotDefinedException, SQLException {
return prepConn.executeUpdate(key, index, values);
}
/**
*
* @param key
* @param values
* @return
* @throws SQLNoConnectionException
* @throws SQLPreparedQueryNotDefinedException
* @throws SQLException
*/
public int executeUpdate(ISQLKey key, Object... values) throws SQLNoConnectionException, SQLPreparedQueryNotDefinedException, SQLException {
return prepConn.executeUpdate(key, values);
}
/**
*
* @param key
* @param values
* @return
* @throws SQLNoConnectionException
* @throws SQLPreparedQueryNotDefinedException
* @throws SQLException
*/
public ResultSet executeQuery(ISQLKey key, Map values) throws SQLNoConnectionException, SQLPreparedQueryNotDefinedException, SQLException {
return prepConn.executeQuery(key, values);
}
/**
*
* @param key
* @param index
* @param values
* @return
* @throws SQLNoConnectionException
* @throws SQLPreparedQueryNotDefinedException
* @throws SQLException
*/
public ResultSet executeQuery(ISQLKey key, int[] index, Object[] values) throws SQLNoConnectionException, SQLPreparedQueryNotDefinedException, SQLException {
return prepConn.executeQuery(key, index, values);
}
/**
*
* @param key
* @param values
* @return
* @throws SQLNoConnectionException
* @throws SQLPreparedQueryNotDefinedException
* @throws SQLException
*/
public ResultSet executeQuery(ISQLKey key, Object... values) throws SQLNoConnectionException, SQLPreparedQueryNotDefinedException, SQLException {
return prepConn.executeQuery(key, values);
}
/**
*
* @param key
* @return
* @throws SQLNoConnectionException
* @throws SQLPreparedQueryNotDefinedException
* @throws SQLException
*/
public boolean execute(ISQLKey key) throws SQLNoConnectionException, SQLPreparedQueryNotDefinedException, SQLException {
return prepConn.execute(key);
}
/**
*
* @return
*/
public Set getStatementKeySet() {
return prepConn.getStatementKeySet();
}
/**
*
* @throws SQLException
*/
@Override
public void disconnect() throws SQLException {
prepConn.closeStatements();
prepConn.setConnection(null);
super.disconnect();
}
/**
*
* @throws SQLException
* @throws PropertyNotFoundException
* @throws SQLConnectionPresentException
*/
@Override
public void connect() throws SQLException, PropertyNotFoundException, SQLConnectionPresentException {
super.connect();
prepConn.setConnection(getConnection());
}
/**
*
* @param prop
* @throws SQLException
* @throws PropertyNotFoundException
* @throws SQLConnectionPresentException
*/
@Override
public void connect(SQLDatabaseProperties prop) throws SQLException, PropertyNotFoundException, SQLConnectionPresentException {
super.connect(prop);
prepConn.setConnection(getConnection());
}
/**
*
* @param host
* @param username
* @param password
* @throws SQLException
* @throws SQLConnectionPresentException
*/
@Override
public void connect(String host, String username, String password) throws SQLException, SQLConnectionPresentException {
super.connect(host, username, password);
prepConn.setConnection(getConnection());
}
/**
*
* @param hostname
* @param dbName
* @param username
* @param password
* @throws SQLException
* @throws SQLConnectionPresentException
*/
@Override
public void connect(String hostname, String dbName, String username, String password) throws SQLException, SQLConnectionPresentException {
super.connect(hostname, dbName, username, password);
prepConn.setConnection(getConnection());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy