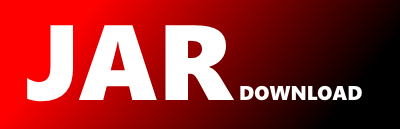
com.github.asteraether.tomlib.util.PowerShellVoice Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of TomLib Show documentation
Show all versions of TomLib Show documentation
A simple collection of usefull things in java.
The newest version!
/*
* To change this license header, choose License Headers in Project Properties.
* To change this template file, choose Tools | Templates
* and open the template in the editor.
*/
package com.github.asteraether.tomlib.util;
import java.io.BufferedWriter;
import java.io.IOException;
import java.io.OutputStreamWriter;
import java.util.AbstractMap;
import java.util.Map.Entry;
import java.util.concurrent.ConcurrentLinkedQueue;
/**
*
* @author Thomas
*/
public class PowerShellVoice {
private final ConcurrentLinkedQueue> texts;
private final ProcessBuilder builder;
private Thread sayThread;
private final Process shell;
private final BufferedWriter commandWriter;
/**
*
* @throws IOException
*/
public PowerShellVoice() throws IOException {
texts = new ConcurrentLinkedQueue<>();
builder = new ProcessBuilder("powershell.exe", "-NoExit", "-");
shell = builder.start();
commandWriter = new BufferedWriter(new OutputStreamWriter(shell.getOutputStream()));
commandWriter.write("$voice = New-Object -ComObject SAPI.SPVoice;");
commandWriter.newLine();
commandWriter.flush();
}
/**
*
* @param text
* @param speed
*/
public synchronized void say(String text, int speed) {
texts.add(new AbstractMap.SimpleEntry<>(text, speed));
if (sayThread == null || !sayThread.isAlive()) {
sayThread = new Thread(()
-> {
while (!texts.isEmpty()) {
String command = "$voice.Rate = " + texts.peek().getValue() + ";"
+ "$voice.Speak(\"" + texts.peek().getKey() + "\") | out-null;";
try {
commandWriter.write(command);
commandWriter.newLine();
commandWriter.flush();
texts.poll();
} catch (IOException ex) {
ex.printStackTrace();
}
}
});
sayThread.start();
}
}
/**
*
* @throws IOException
*/
public void close() throws IOException {
commandWriter.write("exit");
commandWriter.newLine();
commandWriter.flush();
}
/**
*
* @param text
*/
public synchronized void say(String text) {
say(text, 2);
}
/**
*
* @param text
*/
public synchronized void say(Object text) {
say(text.toString(), 2);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy