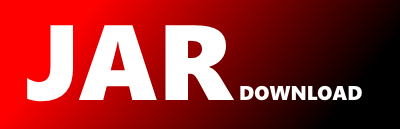
com.github.asteraether.tomlib.util.StringUtil Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of TomLib Show documentation
Show all versions of TomLib Show documentation
A simple collection of usefull things in java.
The newest version!
/*
* To change this license header, choose License Headers in Project Properties.
* To change this template file, choose Tools | Templates
* and open the template in the editor.
*/
package com.github.asteraether.tomlib.util;
/**
*
* @author Thomas
*/
public class StringUtil {
/**
*
* @param string
* @return
*/
public static String getFirstLettersToUpperCase(String string) {
String[] parts = string.split(" ");
for (int i = 0; i < parts.length; i++) {
if (parts[i].length() <= 0) {
continue;
}
parts[i] = parts[i].substring(0, 1).toUpperCase() + parts[i].substring(1).toLowerCase();
}
String s = "";
for (String part : parts) {
if (part.length() > 0) {
s += part + " ";
}
}
s = s.trim();
return s;
}
/**
*
* @param in
* @param occ
* @return
*/
public static int countOccurrencesOf(String in, char occ) {
int count = 0;
for (int i = 0; i < in.length(); i++) {
if (in.charAt(i) == occ) {
count++;
}
}
return count;
}
/**
*
* @param in
* @param occ
* @param unique
* @return
*/
public static int countOccurrencesOf(String in, String occ, boolean unique) {
boolean cool = false;
int l1 = in.length() + 1, l2 = occ.length(), l3 = l1 - l2, count = 0;
for (int i = 0; i < (l3); i += (unique) ? ((cool) ? l2 : 1) : 1) {
if ((cool = in.substring(i, i + l2).equals(occ))) {
count++;
}
}
return count;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy