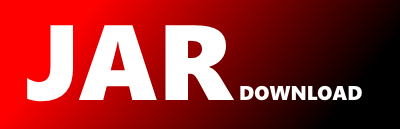
com.github.asteraether.tomlib.util.TrollUtil Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of TomLib Show documentation
Show all versions of TomLib Show documentation
A simple collection of usefull things in java.
The newest version!
/*
* To change this license header, choose License Headers in Project Properties.
* To change this template file, choose Tools | Templates
* and open the template in the editor.
*/
package com.github.asteraether.tomlib.util;
import java.awt.AWTException;
import java.awt.Color;
import java.awt.Font;
import java.awt.FontMetrics;
import java.awt.Graphics;
import java.awt.Robot;
import java.awt.Window;
import java.awt.event.KeyEvent;
/**
*
* @author Thomas
*/
public class TrollUtil {
/**
*
*/
public static void spinMeRound() {
Thread t = new Thread(() -> {
try {
Robot r = new Robot();
int[] keys = {KeyEvent.VK_RIGHT, KeyEvent.VK_DOWN, KeyEvent.VK_LEFT, KeyEvent.VK_UP};
for (int key : keys) {
r.keyPress(KeyEvent.VK_CONTROL);
r.keyPress(KeyEvent.VK_ALT);
r.keyPress(key);
r.keyRelease(KeyEvent.VK_CONTROL);
r.keyRelease(KeyEvent.VK_ALT);
r.keyRelease(key);
}
} catch (AWTException ex) {
System.out.println(ex);
}
});
t.setDaemon(true);
t.start();
}
/**
*
* @param s
* @param color
* @param milseconds
*/
public static void drawStringOnScreen(String s, Color color, long milseconds) {
Thread t = new Thread(() -> {
Window w = new Window(null) {
@Override
public void paint(Graphics g) {
final Font font = getFont().deriveFont(48f);
g.setFont(font);
g.setColor(color);
final String message = s;
FontMetrics metrics = g.getFontMetrics();
g.drawString(message,
(getWidth() - metrics.stringWidth(message)) / 2,
(getHeight() - metrics.getHeight()) / 2);
}
@Override
public void update(Graphics g) {
paint(g);
}
};
w.setAlwaysOnTop(true);
w.setBounds(w.getGraphicsConfiguration().getBounds());
w.setBackground(new Color(0, true));
w.setVisible(true);
try {
Thread.sleep(milseconds);
} catch (InterruptedException ex) {
}
w.dispose();
});
t.start();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy