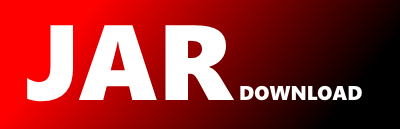
com.github.athi.athifx.gui.application.ApplicationConfigurationUtils Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of athi-fx-gui Show documentation
Show all versions of athi-fx-gui Show documentation
AthiFX project for creating JavaFX simple application GUI.
The newest version!
package com.github.athi.athifx.gui.application;
import com.github.athi.athifx.gui.configuration.ApplicationConfiguration;
import com.github.athi.athifx.gui.configuration.ApplicationConfigurationException;
import com.github.athi.athifx.gui.navigation.view.View;
import com.github.athi.athifx.gui.notification.Notification;
import com.github.athi.athifx.gui.security.Security;
import com.github.athi.athifx.injector.injection.AthiFXInjector;
import com.github.athi.athifx.injector.log.Log;
import com.google.common.collect.LinkedHashMultiset;
import org.reflections.Reflections;
import javax.enterprise.inject.Instance;
import java.util.Iterator;
import java.util.List;
import java.util.Set;
import java.util.stream.Collectors;
import static com.github.athi.athifx.gui.configuration.ApplicationConfiguration.UNCAUGHT_EXCEPTION_HANDLER_NOTIFICATION_MESSAGE;
/**
* Created by Athi
*/
class ApplicationConfigurationUtils {
private static final Log LOGGER = Log.getLogger(AthiFXApplication.class);
static void initUncaughtExceptionHandler() {
Thread.currentThread().setUncaughtExceptionHandler((thread, throwable) -> {
LOGGER.error(throwable.getMessage(), throwable);
Notification.error(UNCAUGHT_EXCEPTION_HANDLER_NOTIFICATION_MESSAGE, throwable.getMessage());
});
}
static void initDefaultApplicationConfiguration() {
try {
Reflections reflections = AthiFXInjector.getReflections();
Set> configuration = reflections.getSubTypesOf(ApplicationConfiguration.class);
if (configuration.size() == 1) {
configuration.iterator().next().newInstance().init();
} else if (configuration.isEmpty()) {
throw new ApplicationConfigurationException("To many application configuration implementations!!");
} else {
throw new ApplicationConfigurationException("No application configuration implementations!!");
}
} catch (Exception e) {
throw new ApplicationConfigurationException("Application configuration exception: " + e.getMessage(), e);
}
}
static void validateSecurityDefinition(Instance securityInstance) {
Iterator iterator = securityInstance.iterator();
if (iterator.hasNext()) {
iterator.next();
if (iterator.hasNext()) {
throw new ApplicationConfigurationException("To many Security implementations!!");
}
}
}
static void validateViewDefinitions() {
Reflections reflections = AthiFXInjector.getReflections();
List itemIds = reflections.getTypesAnnotatedWith(View.class)
.stream()
.map(type -> type.getAnnotation(View.class))
.map(View::itemId)
.collect(Collectors.toList());
LinkedHashMultiset itemIdsMultiset = LinkedHashMultiset.create(itemIds);
itemIdsMultiset.entrySet().removeIf(entry -> entry.getCount() == 1);
Set duplicates = itemIdsMultiset.elementSet();
if (!duplicates.isEmpty()) {
throw new ApplicationConfigurationException("Duplicate View id's: " + duplicates);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy