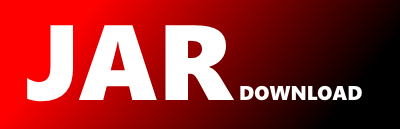
it.auties.whatsapp.model.message.server.ProtocolMessageSpec Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cobalt Show documentation
Show all versions of cobalt Show documentation
Standalone fully-featured Whatsapp Web API for Java and Kotlin
package it.auties.whatsapp.model.message.server;
import it.auties.whatsapp.model.message.server.ProtocolMessage;
import it.auties.protobuf.stream.ProtobufInputStream;
import it.auties.protobuf.stream.ProtobufOutputStream;
public class ProtocolMessageSpec {
public static byte[] encode(ProtocolMessage protoInputObject) {
if(protoInputObject == null) {
return null;
}
var outputStream = new ProtobufOutputStream();
var key = protoInputObject.key();
var key0 = key.orElse(null);
if(key0 != null) {
outputStream.writeBytes(1, it.auties.whatsapp.model.message.model.ChatMessageKeySpec.encode(key0));
}
outputStream.writeInt32(2, it.auties.whatsapp.model.message.server.ProtocolMessageTypeSpec.encode(protoInputObject.protocolType()));
outputStream.writeUInt64(4, protoInputObject.ephemeralExpiration());
outputStream.writeUInt64(5, protoInputObject.ephemeralSettingTimestampSeconds());
var historySyncNotification = protoInputObject.historySyncNotification();
var historySyncNotification0 = historySyncNotification.orElse(null);
if(historySyncNotification0 != null) {
outputStream.writeBytes(6, it.auties.whatsapp.model.sync.HistorySyncNotificationSpec.encode(historySyncNotification0));
}
var appStateSyncKeyShare = protoInputObject.appStateSyncKeyShare();
var appStateSyncKeyShare0 = appStateSyncKeyShare.orElse(null);
if(appStateSyncKeyShare0 != null) {
outputStream.writeBytes(7, it.auties.whatsapp.model.sync.AppStateSyncKeyShareSpec.encode(appStateSyncKeyShare0));
}
var appStateSyncKeyRequest = protoInputObject.appStateSyncKeyRequest();
var appStateSyncKeyRequest0 = appStateSyncKeyRequest.orElse(null);
if(appStateSyncKeyRequest0 != null) {
outputStream.writeBytes(8, it.auties.whatsapp.model.sync.AppStateSyncKeyRequestSpec.encode(appStateSyncKeyRequest0));
}
var initialSecurityNotificationSettingSync = protoInputObject.initialSecurityNotificationSettingSync();
var initialSecurityNotificationSettingSync0 = initialSecurityNotificationSettingSync.orElse(null);
if(initialSecurityNotificationSettingSync0 != null) {
outputStream.writeBytes(9, it.auties.whatsapp.model.sync.InitialSecurityNotificationSettingSyncSpec.encode(initialSecurityNotificationSettingSync0));
}
var appStateFatalExceptionNotification = protoInputObject.appStateFatalExceptionNotification();
var appStateFatalExceptionNotification0 = appStateFatalExceptionNotification.orElse(null);
if(appStateFatalExceptionNotification0 != null) {
outputStream.writeBytes(10, it.auties.whatsapp.model.sync.AppStateFatalExceptionNotificationSpec.encode(appStateFatalExceptionNotification0));
}
var disappearingMode = protoInputObject.disappearingMode();
var disappearingMode0 = disappearingMode.orElse(null);
if(disappearingMode0 != null) {
outputStream.writeBytes(11, it.auties.whatsapp.model.chat.ChatDisappearSpec.encode(disappearingMode0));
}
var editedMessage = protoInputObject.editedMessage();
var editedMessage0 = editedMessage.orElse(null);
if(editedMessage0 != null) {
outputStream.writeBytes(14, it.auties.whatsapp.model.message.model.MessageContainerSpec.encode(editedMessage0));
}
outputStream.writeInt64(15, protoInputObject.timestampMilliseconds());
return outputStream.toByteArray();
}
public static ProtocolMessage decode(byte[] input) {
if(input == null) {
return null;
}
var inputStream = new ProtobufInputStream(input);
java.util.Optional key = java.util.Optional.empty();
it.auties.whatsapp.model.message.server.ProtocolMessage.Type protocolType = null;
long ephemeralExpiration = 0l;
long ephemeralSettingTimestampSeconds = 0l;
java.util.Optional historySyncNotification = java.util.Optional.empty();
java.util.Optional appStateSyncKeyShare = java.util.Optional.empty();
java.util.Optional appStateSyncKeyRequest = java.util.Optional.empty();
java.util.Optional initialSecurityNotificationSettingSync = java.util.Optional.empty();
java.util.Optional appStateFatalExceptionNotification = java.util.Optional.empty();
java.util.Optional disappearingMode = java.util.Optional.empty();
java.util.Optional editedMessage = java.util.Optional.empty();
long timestampMilliseconds = 0l;
while(inputStream.readTag()) {
switch(inputStream.index()) {
case 1 -> key = java.util.Optional.ofNullable(it.auties.whatsapp.model.message.model.ChatMessageKeySpec.decode(inputStream.readBytes()));
case 2 -> protocolType = it.auties.whatsapp.model.message.server.ProtocolMessageTypeSpec.decode(inputStream.readInt32()).orElse(null);
case 4 -> ephemeralExpiration = inputStream.readInt64();
case 5 -> ephemeralSettingTimestampSeconds = inputStream.readInt64();
case 6 -> historySyncNotification = java.util.Optional.ofNullable(it.auties.whatsapp.model.sync.HistorySyncNotificationSpec.decode(inputStream.readBytes()));
case 7 -> appStateSyncKeyShare = java.util.Optional.ofNullable(it.auties.whatsapp.model.sync.AppStateSyncKeyShareSpec.decode(inputStream.readBytes()));
case 8 -> appStateSyncKeyRequest = java.util.Optional.ofNullable(it.auties.whatsapp.model.sync.AppStateSyncKeyRequestSpec.decode(inputStream.readBytes()));
case 9 -> initialSecurityNotificationSettingSync = java.util.Optional.ofNullable(it.auties.whatsapp.model.sync.InitialSecurityNotificationSettingSyncSpec.decode(inputStream.readBytes()));
case 10 -> appStateFatalExceptionNotification = java.util.Optional.ofNullable(it.auties.whatsapp.model.sync.AppStateFatalExceptionNotificationSpec.decode(inputStream.readBytes()));
case 11 -> disappearingMode = java.util.Optional.ofNullable(it.auties.whatsapp.model.chat.ChatDisappearSpec.decode(inputStream.readBytes()));
case 14 -> editedMessage = java.util.Optional.ofNullable(it.auties.whatsapp.model.message.model.MessageContainerSpec.decode(inputStream.readBytes()));
case 15 -> timestampMilliseconds = inputStream.readInt64();
default -> inputStream.skipBytes();
}
}
return new it.auties.whatsapp.model.message.server.ProtocolMessage(key, protocolType, ephemeralExpiration, ephemeralSettingTimestampSeconds, historySyncNotification, appStateSyncKeyShare, appStateSyncKeyRequest, initialSecurityNotificationSettingSync, appStateFatalExceptionNotification, disappearingMode, editedMessage, timestampMilliseconds);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy