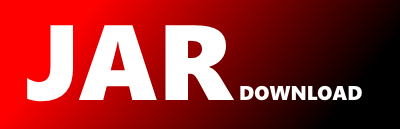
com.github.basking2.sdsai.itrex.iterators.FutureIterator Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sdsai-itrex Show documentation
Show all versions of sdsai-itrex Show documentation
An S-Expression inspiried library focused on iterators.
package com.github.basking2.sdsai.itrex.iterators;
import java.util.Iterator;
import java.util.concurrent.CompletableFuture;
import java.util.concurrent.Executor;
import java.util.concurrent.Future;
/**
* A class that wraps an iterator such that calls to {@link #next()} result in a {@link Future} being returned.
*
* The wrapped iterator must be thread-safe.
*/
public class FutureIterator implements Iterator> {
private final Iterator iterator;
private final Executor executor;
/**
* Create a new FutureIterator.
*
* @param iterator A thread-safe iterator.
* @param executor The executor {@link Future}s will be completed in.
*/
public FutureIterator(final Iterator iterator, final Executor executor) {
this.iterator = iterator;
this.executor = executor;
}
/**
* Return true if there are more elements in the source iterator.
*
* This call is not reliable as a call to {@link Iterator#next()} may be happening
* as this call returns.
*
* @return true if, at the moment {@link Iterator#hasNext()} is called on the wrapped
* iterator, it returns true. False otherwise.
*/
@Override
public boolean hasNext() {
return iterator.hasNext();
}
@Override
public Future next() {
final CompletableFuture f = new CompletableFuture<>();
executor.execute(() -> {
try {
f.complete(iterator.next());
}
catch (final Throwable t) {
f.completeExceptionally(t);
}
});
return f;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy