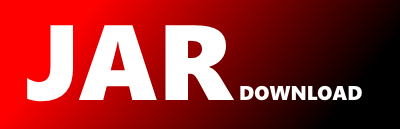
com.github.basking2.sdsai.itrex.iterators.Iterators Maven / Gradle / Ivy
package com.github.basking2.sdsai.itrex.iterators;
import com.github.basking2.sdsai.itrex.iterators.splitjoin.JoinUncertainIteratorsIterator;
import com.github.basking2.sdsai.itrex.iterators.splitjoin.SplitMapUncertainIterator;
import java.util.Arrays;
import java.util.Iterator;
import java.util.NoSuchElementException;
import java.util.List;
import java.util.ArrayList;
import java.util.concurrent.ExecutorService;
import java.util.function.Function;
import java.util.function.Predicate;
public class Iterators {
public static Iterator> EMPTY_ITERATOR = new Iterator
© 2015 - 2025 Weber Informatics LLC | Privacy Policy