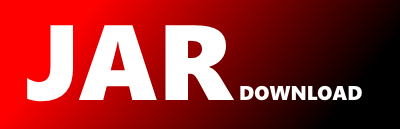
com.github.basking2.sdsai.itrex.SimpleExpressionParser Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sdsai-itrex Show documentation
Show all versions of sdsai-itrex Show documentation
An S-Expression inspiried library focused on iterators.
package com.github.basking2.sdsai.itrex;
import java.util.ArrayList;
import java.util.List;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
/**
* A parser that is JSON-esque, but removes some commas and quotes to better suite the particulars of this language.
*
* The JSON
*
*
* [ "map", ["curry", "f"], ["list", 1, 2, 3]]
*
*
* may be parsed to the same expression
*
*
* [map [curry f] [list 1 2 3]]
*
*
* String may still be used, but we allow for unquoted tokens.
*/
public class SimpleExpressionParser {
public static final Pattern SKIP_WS = Pattern.compile("^\\s*");
public static final Pattern COMMA = Pattern.compile("^\\s*,");
public static final Pattern OPEN_BRACKET = Pattern.compile("^\\s*\\[");
public static final Pattern CLOSE_BRACKET = Pattern.compile("^\\s*\\]");
public static final Pattern BLOCK_COMMENT = Pattern.compile(
"^\\s*\\[\\*" +
"([^*]|\\*[^\\]])*" +
"\\*\\]"
);
public static final Pattern FIRST_QUOTE = Pattern.compile("^\\s*\"");
public static final Pattern QUOTED_STRING = Pattern.compile("^\"((?:\\\\\\\\|\\\\\"|[^\"])*)\"");
public static final Pattern INTEGER = Pattern.compile("^(?:-?\\d+)");
public static final Pattern LONG = Pattern.compile("^(?:-?\\d+)[lL]");
public static final Pattern DOUBLE = Pattern.compile("^(?:-?\\d+\\.\\d+[dD]?|\\d+[dD])");
public static final Pattern WORD = Pattern.compile("^(?:[\\w\\.\\-:|]+)");
private final String expression;
private int position;
/**
* Construct the parser, but do not parse.
* @see #parse()
* @param expression An expression to parse.
*/
public SimpleExpressionParser(final String expression) {
this.expression = expression;
this.position = 0;
}
private static int matchOrNeg1(final String expression, final Pattern pattern, int start) {
final Matcher m = pattern.matcher(expression).region(start, expression.length());
if (m.find()) {
return m.group().length();
}
return -1;
}
private static int firstQuote(final String expression, final int start) {
return matchOrNeg1(expression, FIRST_QUOTE, start);
}
private static int openBracket(final String expression, final int start) {
return matchOrNeg1(expression, OPEN_BRACKET, start);
}
private static int closeBracket(final String expression, final int start) {
return matchOrNeg1(expression, CLOSE_BRACKET, start);
}
private static int blockComment(final String expression, final int start) {
return matchOrNeg1(expression, BLOCK_COMMENT, start);
}
private void skipWs() {
final Matcher m = SKIP_WS.matcher(expression).region(position, expression.length());
if (m.find()) {
position += m.group().length();
};
}
/**
* Skip past whitespace and a comma. Use in list construction.
*
* Lists do not require a comma, but one is tolerated.
*/
private void skipComma() {
final Matcher m = COMMA.matcher(expression).region(position, expression.length());
if (m.find()) {
position += m.group().length();
};
}
final public Object parse() {
skipWs();
for (
int i = blockComment(expression, position);
i >= 0;
i = blockComment(expression, position)
) {
position += i;
}
int i = openBracket(expression, position);
// This is a bracket expression.
if (i > 0) {
// Move the position up to the opening [ and parse that list.
position += i;
return parseList();
}
// At end of expression, return null.
if (position >= expression.length()) {
return null;
}
return parseLiteral();
}
/**
* When the position points after an opening [, this parses that list.
* @return
*/
final private List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy