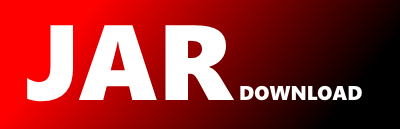
com.github.basking2.sdsai.itrex.functions.IfFunction Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sdsai-itrex Show documentation
Show all versions of sdsai-itrex Show documentation
An S-Expression inspiried library focused on iterators.
package com.github.basking2.sdsai.itrex.functions;
import java.util.Iterator;
import com.github.basking2.sdsai.itrex.EvaluationContext;
import com.github.basking2.sdsai.itrex.SExprRuntimeException;
import com.github.basking2.sdsai.itrex.iterators.EvaluatingIterator;
public class IfFunction implements FunctionInterface
© 2015 - 2024 Weber Informatics LLC | Privacy Policy