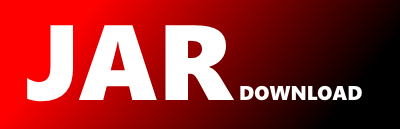
com.github.basking2.sdsai.itrex.functions.functional.FoldLeftFunction Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sdsai-itrex Show documentation
Show all versions of sdsai-itrex Show documentation
An S-Expression inspiried library focused on iterators.
package com.github.basking2.sdsai.itrex.functions.functional;
import com.github.basking2.sdsai.itrex.EvaluationContext;
import com.github.basking2.sdsai.itrex.SExprRuntimeException;
import com.github.basking2.sdsai.itrex.functions.AbstractFunction2;
import com.github.basking2.sdsai.itrex.functions.FunctionInterface;
import java.util.Iterator;
import static com.github.basking2.sdsai.itrex.iterators.Iterators.toIterator;
import static java.util.Arrays.asList;
/**
* Fold a given value over all elements.
*
*
* {@code
* [* Assume the add function exists. *]
*
* [foldLeft [curry add] 0 [1,2,3,4]]
*
* }
*
*
* This is much like the foldLeft function common in Scala or ML languages.
*
* There is no {@code foldRight} as iterators make it expensive to materialize to a list and then foldRight.
* If such an algorithm is necessary, operate on the list in a reversed projection.
*/
public class FoldLeftFunction extends AbstractFunction2, Object, Object> {
@Override
protected Object applyImpl(
final FunctionInterface
© 2015 - 2024 Weber Informatics LLC | Privacy Policy