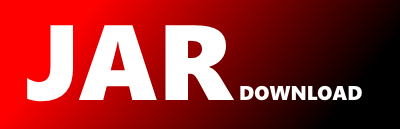
com.github.basking2.sdsai.itrex.functions.java.JavaFunction Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sdsai-itrex Show documentation
Show all versions of sdsai-itrex Show documentation
An S-Expression inspiried library focused on iterators.
package com.github.basking2.sdsai.itrex.functions.java;
import com.github.basking2.sdsai.itrex.EvaluationContext;
import com.github.basking2.sdsai.itrex.SExprRuntimeException;
import com.github.basking2.sdsai.itrex.functions.AbstractFunction2;
import java.lang.reflect.Method;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Iterator;
import java.util.List;
import static com.github.basking2.sdsai.itrex.util.Reflection.isAssignable;
/**
* Function that takes the name of a function, a target object, and a list or iterable of arguments.
*
* If the argument is a collection or an iterator, each element is marshaled as an argument.
* If the argument is neither an collection nor an iterator, it is marshaled as an argument itself.
*
* {@code
*
* [java new [classOf java.util.ArrayList]]
*
* }
*
* {@code
*
* [java add [get myArray] [list 1]]
*
* }
*
* {@code
*
* [java add [get myArray] 1]
*
* }
*/
public class JavaFunction extends AbstractFunction2 {
@Override
protected Object applyImpl(final String functionName, final Object target, final Iterator> rest, final EvaluationContext context) {
final List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy