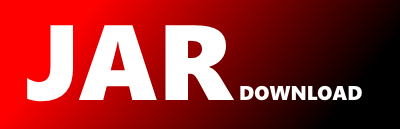
com.github.becausetesting.commonswindow.CommonsWindowSample Maven / Gradle / Ivy
package com.github.becausetesting.commonswindow;
import java.awt.BorderLayout;
import java.awt.Component;
/*-
* #%L
* commons-window
* $Id:$
* $HeadURL:$
* %%
* Copyright (C) 2016 Alter Hu
* %%
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
* #L%
*/
import java.awt.EventQueue;
import java.awt.Insets;
import java.awt.SplashScreen;
import java.awt.Toolkit;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.awt.event.InputEvent;
import java.awt.event.KeyEvent;
import java.awt.event.MouseAdapter;
import java.awt.event.MouseEvent;
import javax.swing.BoxLayout;
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JMenu;
import javax.swing.JMenuBar;
import javax.swing.JMenuItem;
import javax.swing.JPanel;
import javax.swing.JPopupMenu;
import javax.swing.JSeparator;
import javax.swing.JTabbedPane;
import javax.swing.JTextField;
import javax.swing.JTextPane;
import javax.swing.KeyStroke;
import org.apache.log4j.Logger;
import com.github.becausetesting.commonswindow.utils.LookAndFeelUtils;
import com.github.becausetesting.commonswindow.utils.ui.JMessageDialogUtils;
import com.github.becausetesting.commonswindow.utils.ui.JTextFieldUtils;
import com.github.becausetesting.commonswindow.utils.ui.JTextPaneUtils;
import com.github.becausetesting.commonswindow.utils.ui.SplashScreenUtils;
public class CommonsWindowSample {
private JFrame frame;
private static Logger log = Logger.getLogger(CommonsWindowSample.class);
private JMenuBar JMenuBarObject;
private JMenu JMenuFile;
private JMenuItem mntmOpen;
private JMenuItem mntmEdit;
private JMenu JMenuEdit;
private JMenu JMenuHelp;
private JSeparator separator;
private JMenuItem mntmCut;
private JMenuItem mntmCopy;
private JMenuItem mntmPaste;
private JMenuItem mntmWelcome;
private JSeparator separator_1;
private JMenuItem mntmAboutIt;
private JTabbedPane tabbedPane;
private JPanel panel;
private JPanel panel_1;
private JPanel panel_2;
private JButton btnOpenDialog;
private JPopupMenu popupMenu_1;
private JTextField textField_1;
private JTextPane textPane;
private JButton btnShowLog;
/**
* Launch the application.
*/
public static void main(String[] args) {
EventQueue.invokeLater(new Runnable() {
public void run() {
try {
SplashScreen splashScreen = SplashScreenUtils.show();
CommonsWindowSample window = new CommonsWindowSample();
SplashScreenUtils.updateSplashMessage(splashScreen, "Start window UI...");
LookAndFeelUtils.initLookAndFeel(window.frame);
window.frame.setVisible(true);
log.info("Start Windows..");
} catch (Exception e) {
e.printStackTrace();
}finally {
SplashScreenUtils.close();
}
}
});
}
/**
* Create the application.
*/
public CommonsWindowSample() {
initialize();
}
/**
* Initialize the contents of the frame.
*/
private void initialize() {
frame = new JFrame();
frame.setIconImage(Toolkit.getDefaultToolkit().getImage(CommonsWindowSample.class.getResource("/images/logo.ico")));
frame.setBounds(100, 100, 828, 622);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
JMenuBarObject = new JMenuBar();
frame.setJMenuBar(JMenuBarObject);
JMenuFile = new JMenu("File");
JMenuBarObject.add(JMenuFile);
mntmOpen = new JMenuItem("Open");
mntmOpen.setAccelerator(KeyStroke.getKeyStroke(KeyEvent.VK_O, InputEvent.CTRL_MASK));
JMenuFile.add(mntmOpen);
separator = new JSeparator();
JMenuFile.add(separator);
mntmEdit = new JMenuItem("Exit");
JMenuFile.add(mntmEdit);
JMenuEdit = new JMenu("Edit");
JMenuBarObject.add(JMenuEdit);
mntmCut = new JMenuItem("Cut");
mntmCut.setAccelerator(KeyStroke.getKeyStroke(KeyEvent.VK_X, InputEvent.CTRL_MASK));
JMenuEdit.add(mntmCut);
mntmCopy = new JMenuItem("Copy");
mntmCopy.setAccelerator(KeyStroke.getKeyStroke(KeyEvent.VK_C, InputEvent.CTRL_MASK));
JMenuEdit.add(mntmCopy);
mntmPaste = new JMenuItem("Paste");
mntmPaste.setAccelerator(KeyStroke.getKeyStroke(KeyEvent.VK_V, InputEvent.CTRL_MASK));
JMenuEdit.add(mntmPaste);
JMenuHelp = new JMenu("Help");
JMenuBarObject.add(JMenuHelp);
mntmWelcome = new JMenuItem("Welcome");
JMenuHelp.add(mntmWelcome);
separator_1 = new JSeparator();
JMenuHelp.add(separator_1);
mntmAboutIt = new JMenuItem("About it");
JMenuHelp.add(mntmAboutIt);
tabbedPane = new JTabbedPane(JTabbedPane.TOP);
tabbedPane.setSelectedIndex(tabbedPane.getTabCount()-1);
frame.getContentPane().add(tabbedPane, BorderLayout.CENTER);
panel = new JPanel();
tabbedPane.addTab("New tab", null, panel, null);
btnOpenDialog = new JButton("open dialog");
btnOpenDialog.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
int confirm = JMessageDialogUtils.confirm(frame, "sample title", "sample message");
log.info(confirm);
JMessageDialogUtils.info(frame,null,"info");
}
});
popupMenu_1 = new JPopupMenu();
addPopup(panel, popupMenu_1);
textField_1=new JTextFieldUtils().new HintTextField("5fdfdfdftrwterep");
//textField_1.setUI(new HintTextFieldUI("testing now",true));
panel.add(textField_1);
textField_1.setColumns(10);
panel.add(btnOpenDialog);
panel_1 = new JPanel();
tabbedPane.addTab("New tab", null, panel_1, null);
panel_1.setLayout(new BoxLayout(panel_1, BoxLayout.Y_AXIS));
btnShowLog = new JButton("show log");
btnShowLog.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
log.warn("show log 1");
}
});
panel_1.add(btnShowLog);
textPane = new JTextPane();
//textPane.setBorder(eb);
//tPane.setBorder(BorderFactory.createLineBorder(Color.DARK_GRAY));
textPane.setMargin(new Insets(5, 5, 5, 5));
panel_1.add(textPane);
JTextPaneUtils.createLog4jOutput(textPane);
panel_2 = new JPanel();
tabbedPane.addTab("New tab", null, panel_2, null);
tabbedPane.setSelectedIndex(1);
}
/**
* @param component the component object
* @param popup the menu object
*/
private static void addPopup(Component component, final JPopupMenu popup) {
component.addMouseListener(new MouseAdapter() {
public void mousePressed(MouseEvent e) {
if (e.isPopupTrigger()) {
showMenu(e);
}
}
public void mouseReleased(MouseEvent e) {
if (e.isPopupTrigger()) {
showMenu(e);
}
}
private void showMenu(MouseEvent e) {
popup.show(e.getComponent(), e.getX(), e.getY());
}
});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy