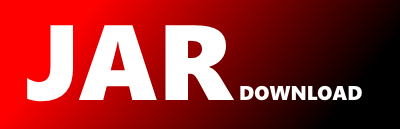
com.github.becausetesting.commonswindow.RowDataModel Maven / Gradle / Ivy
package com.github.becausetesting.commonswindow;
/*-
* #%L
* commons-window
* $Id:$
* $HeadURL:$
* %%
* Copyright (C) 2016 Alter Hu
* %%
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
* #L%
*/
import java.sql.ResultSet;
import java.sql.ResultSetMetaData;
import java.sql.SQLException;
import java.util.ArrayList;
import java.util.List;
import java.util.Vector;
import javax.swing.table.DefaultTableModel;
import org.apache.log4j.Logger;
public class RowDataModel extends DefaultTableModel {
/**
*
*/
private static final long serialVersionUID = 4766011826162808583L;
private static Logger log = Logger.getLogger(RowDataModel.class);
/**
*
*/
public RowDataModel() {
// TODO Auto-generated constructor stub
super();
}
/**
* @param columns
* columns
* @param data
* the data list
*/
public RowDataModel(List columns, List data) {
// TODO Auto-generated constructor stub
super(convertToVectorData(data), convertToVector(columns));
}
/**
* @param rowCount
* the row count
* @param columnCount
* the column count
*/
public RowDataModel(int rowCount, int columnCount) {
// TODO Auto-generated constructor stub
super(rowCount, columnCount);
}
/**
* @param columnNames
* the column name
* @param rowCount
* the row count
*/
public RowDataModel(Vector columnNames, int rowCount) {
// TODO Auto-generated constructor stub
super(columnNames, rowCount);
}
/**
* @param columnNames
* the column array
* @param rowCount
* th row count
*/
public RowDataModel(Object[] columnNames, int rowCount) {
// TODO Auto-generated constructor stub
super(columnNames, rowCount);
}
/**
* @param columnNames
* the column vector
* @param data
* the data vector
*/
public RowDataModel(Vector columnNames, Vector data) {
// TODO Auto-generated constructor stub
super(data, columnNames);
}
/**
* @param columnNames
* column array
* @param data
* the data array
*/
public RowDataModel(Object[] columnNames, Object[][] data) {
// TODO Auto-generated constructor stub
super(data, columnNames);
}
/*
* (non-Javadoc)
*
* @see javax.swing.table.AbstractTableModel#getColumnClass(int)
*/
@Override
public Class> getColumnClass(int columnIndex) {
// TODO Auto-generated method stub,used to customized the boolean value
// to default jtable checkbox
for (int rowIndex = 0; rowIndex < getRowCount(); rowIndex++) {
Object row = getValueAt(rowIndex, columnIndex);
if (row != null) {
return row.getClass();
}
}
return String.class;
}
/*
* (non-Javadoc)
*
* @see javax.swing.table.DefaultTableModel#isCellEditable(int, int)
*/
@Override
public boolean isCellEditable(int row, int column) {
// TODO Auto-generated method stub
return true;
}
/**
* @param rows
* the row array
* @return a List of Objects for the requested rows.
*/
@SuppressWarnings("unchecked")
public List getRowsDataAsList(int... rows) {
ArrayList rowData = new ArrayList(rows.length);
Vector data = getDataVector();
for (int i = 0; i < rows.length; i++) {
rowData.add(data.elementAt(rows[i]));
}
return rowData;
}
/**
* Adds a row of data to the end of the model. Notification of the row being
* added will be generated.
*
* @param rowData
* data of the row being added
*/
public void addRows(Object[][] rowData) {
for (Object[] eachRow : rowData) {
addRow(eachRow);
}
}
/**
* Adds a row of data to the end of the model. Notification of the row being
* added will be generated.
*
* @param rowData
* data of the row being added
*/
public void addRows(List rowData) {
for (Object eachRow : rowData) {
List eachData = (List) eachRow;
addRow(eachData);
}
}
/**
* Adds a row of data to the end of the model. Notification of the row being
* added will be generated.
*
* @param rowData
* data of the row being added
*/
public void addRow(List rowData) {
addRow(convertToVectorData(rowData));
}
/**
* remove row's data
*
* @param rows
* the row array
*/
public void removeRow(int... rows) {
for (int i = rows.length - 1; i >= 0; i--) {
removeRow(i);
}
}
public void Clear() {
removeRow(new int[getRowCount()]);
}
/**
* set the data for specified row
*
* @param row
* the row number
* @param data
* the row data list
*/
@SuppressWarnings("unchecked")
public void setValueAt(int row, List data) {
Vector oldVector = getDataVector();
Object object = oldVector.elementAt(row);
oldVector.setElementAt(object, row);
fireTableRowsUpdated(row, row);
}
/**
* Returns an attribute value for the cell at row
and
* column
.
*
* @param row
* the row whose value is to be queried
* @return the value Object at the specified cell
* @exception ArrayIndexOutOfBoundsException
* if an invalid row or column was given
*/
public List getValueAt(int row) {
Vector rowVector = (Vector) dataVector.elementAt(row);
return rowVector;
}
/**
* create the table model from resultset
*
* @param resultSet
* the resultobject
* @return the RowDataModel object
*/
public static RowDataModel createModelFromResultSet(ResultSet resultSet) {
ArrayList columnNames = new ArrayList();
ArrayList data = new ArrayList();
try {
ResultSetMetaData metaData = resultSet.getMetaData();
int columns = metaData.getColumnCount();
columnNames.add("");
for (int k = 1; k <= columns; k++) {
String columnName = metaData.getColumnName(k);
String columnLabel = metaData.getColumnLabel(k);
if (columnLabel.equals(columnName))
columnNames.add(formatColumnName(columnName));
else
columnNames.add(columnLabel);
}
while (resultSet.next()) {
ArrayList
© 2015 - 2025 Weber Informatics LLC | Privacy Policy