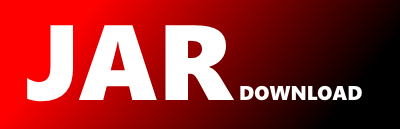
com.github.becausetesting.commonswindow.utils.LookAndFeelUtils Maven / Gradle / Ivy
package com.github.becausetesting.commonswindow.utils;
import java.awt.Component;
/*-
* #%L
* commons-window
* $Id:$
* $HeadURL:$
* %%
* Copyright (C) 2016 Alter Hu
* %%
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
* #L%
*/
import java.awt.Font;
import javax.swing.JDialog;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.LookAndFeel;
import javax.swing.SwingUtilities;
import javax.swing.UIManager;
import javax.swing.UIManager.LookAndFeelInfo;
import javax.swing.UnsupportedLookAndFeelException;
import javax.swing.plaf.metal.MetalLookAndFeel;
import com.github.becausetesting.commonswindow.LightOceanTheme;
import com.jgoodies.looks.plastic.Plastic3DLookAndFeel;
import com.jgoodies.looks.plastic.theme.SkyBlue;
import com.jgoodies.looks.windows.WindowsLookAndFeel;
import com.sun.jna.Platform;
public class LookAndFeelUtils {
private static final String DARCULA_LAF_CLASS = "com.bulenkov.darcula.DarculaLaf";
public static void initLookAndFeel(Component frame) {
try {
installLnfs();
//WindowsLookAndFeel windowsLookAndFeel=new WindowsLookAndFeel();
UIManager.setLookAndFeel(UIManager.getSystemLookAndFeelClassName());
JFrame.setDefaultLookAndFeelDecorated(true);
JDialog.setDefaultLookAndFeelDecorated(true);
updateLookAndFeel(frame);
} catch (UnsupportedLookAndFeelException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} catch (ClassNotFoundException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} catch (InstantiationException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} catch (IllegalAccessException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
public static void updateLookAndFeel(Component frame){
SwingUtilities.updateComponentTreeUI(frame);
//log.info("Use Windows platform UI Feel.");
}
public static String getSystemLookAndFeelClassName(JFrame frame) {
return UIManager.getSystemLookAndFeelClassName();
}
/**
* Do the supplied object and info object refer to the same ?
*
* @param lookAndFeel
* LF object
* @param lookAndFeelInfo
* LF info object
* @return True if they do
*/
public static boolean matchLnf(LookAndFeel lookAndFeel, LookAndFeelInfo lookAndFeelInfo) {
return lookAndFeel.getClass().getName().equals(lookAndFeelInfo.getClassName());
}
/**
* Install LFs.
*/
public static void installLnfs() {
UIManager.installLookAndFeel("JGoodies Plastic 3D", Plastic3DLookAndFeel.class.getName());
if (Platform.isWindows()) {
UIManager.installLookAndFeel("JGoodies Windows",
com.jgoodies.looks.windows.WindowsLookAndFeel.class.getName());
}
// Darcula is optional
if (isDarculaAvailable()) {
UIManager.installLookAndFeel("Darcula", DARCULA_LAF_CLASS);
}
}
/**
* Use supplied lf.
*
* @param lnfClassName
* Lf class name
*/
public static void useLnf(String lnfClassName) {
try {
// Make l&f specific settings - required before setting the l&f
if (lnfClassName.equals(Plastic3DLookAndFeel.class.getName())) {
MetalLookAndFeel.setCurrentTheme(new SkyBlue());
UIManager.put("jgoodies.useNarrowButtons", Boolean.FALSE);
} else if (lnfClassName.equals(com.jgoodies.looks.windows.WindowsLookAndFeel.class.getName())) {
UIManager.put("jgoodies.useNarrowButtons", Boolean.FALSE);
} else if (lnfClassName.equals(MetalLookAndFeel.class.getName())) {
// Lighten metal/ocean
MetalLookAndFeel.setCurrentTheme(new LightOceanTheme());
}
UIManager.setLookAndFeel(lnfClassName);
} catch (UnsupportedLookAndFeelException e) {
} catch (ClassNotFoundException e) {
} catch (InstantiationException e) {
} catch (IllegalAccessException e) {
}
}
/**
* Use the appropriate look and feel for the current platform.
*
* @return Look and feel class name used
*/
public static String useLnfForPlatform() {
String lnfClassName = null;
if (Platform.isMac()) {
lnfClassName = UIManager.getSystemLookAndFeelClassName();
} else if (Platform.isWindows()) {
lnfClassName = WindowsLookAndFeel.class.getName();
} else {
String xdgCurrentDesktop = System.getenv("XDG_CURRENT_DESKTOP");
if ("Unity".equalsIgnoreCase(xdgCurrentDesktop) || "XFCE".equalsIgnoreCase(xdgCurrentDesktop)
|| "GNOME".equalsIgnoreCase(xdgCurrentDesktop) || "X-Cinnamon".equalsIgnoreCase(xdgCurrentDesktop)
|| "LXDE".equalsIgnoreCase(xdgCurrentDesktop)) {
lnfClassName = UIManager.getSystemLookAndFeelClassName();
} else {
lnfClassName = Plastic3DLookAndFeel.class.getName();
}
}
useLnf(lnfClassName);
return lnfClassName;
}
/**
* Is a Mac lf (Aqua) currently being used?
*
* @return True if it is
*/
public static boolean usingMacLnf() {
String lnfClass = UIManager.getLookAndFeel().getClass().getName();
return Platform.isMac() && UIManager.getSystemLookAndFeelClassName().equals(lnfClass);
}
/**
* Is the JGoodies Plastic 3D lf currently being used?
*
* @return True if it is
*/
public static boolean usingPlastic3DLnf() {
return usingLnf(Plastic3DLookAndFeel.class);
}
/**
* Is the Metal lf currently being used?
*
* @return True if it is
*/
public static boolean usingMetalLnf() {
return usingLnf(MetalLookAndFeel.class);
}
/**
* Is the Windows lf currently being used?
*
* @return True if it is
*/
public static boolean usingWindowsLnf() {
return usingLnf(WindowsLookAndFeel.class);
}
/**
* Is the Windows Classic currently being used?
*
* @return True if it is
*/
public static boolean usingWindowsClassicLnf() {
return usingLnf(WindowsLookAndFeel.class);
}
/**
* Is the supplied lf currently being used?
*
* @return l&f class
*/
private static boolean usingLnf(Class lnfClass) {
String currentLnfClass = UIManager.getLookAndFeel().getClass().getName();
return currentLnfClass.equals(lnfClass.getName());
}
/**
* Does the currently active lf use a dark color scheme?
*/
/**
* @return is dark or not
*/
public static boolean isDarkLnf() {
return UIManager.getLookAndFeel().getClass().getName().equals(DARCULA_LAF_CLASS);
}
/**
* Is optional Darcula LaF available?
*/
/**
* @return darcula available or not
*/
public static boolean isDarculaAvailable() {
try {
Class.forName(DARCULA_LAF_CLASS);
// Darcula has some issues in Java 6
String javaVersion = System.getProperty("java.specification.version");
if (javaVersion.startsWith("1.6")) {
return true;
}
} catch (ClassNotFoundException e) {
// Darcula jar not included
}
return false;
}
/**
* Get default font size for a label.
*
* @return Font size
*/
public static int getDefaultFontSize() {
Font defaultFont = new JLabel().getFont();
return defaultFont.getSize();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy