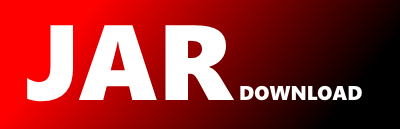
com.github.becausetesting.commonswindow.utils.ui.JESCDialog Maven / Gradle / Ivy
package com.github.becausetesting.commonswindow.utils.ui;
import java.awt.Dialog;
import java.awt.Frame;
import java.awt.GraphicsConfiguration;
import java.awt.Window;
import java.awt.event.ActionEvent;
import java.awt.event.KeyEvent;
import javax.swing.AbstractAction;
import javax.swing.JComponent;
import javax.swing.JDialog;
import javax.swing.JRootPane;
import javax.swing.KeyStroke;
/**
* Extended dialog class that closes itself when escape key was pressed.
*
* This is the usual behavior under Windows and Mac OS.
*
*/
public class JESCDialog extends JDialog {
private static final long serialVersionUID = -3773740513817678414L;
public JESCDialog() {
this((Frame) null, false);
}
public JESCDialog(Frame owner) {
this(owner, false);
}
public JESCDialog(Frame owner, boolean modal) {
this(owner, null, modal);
}
public JESCDialog(Frame owner, String title) {
this(owner, title, false);
}
public JESCDialog(Frame owner, String title, boolean modal) {
super(owner, title, modal);
}
public JESCDialog(Frame owner, String title, boolean modal, GraphicsConfiguration gc) {
super(owner, title, modal, gc);
}
public JESCDialog(Dialog owner) {
this(owner, false);
}
public JESCDialog(Dialog owner, boolean modal) {
this(owner, null, modal);
}
public JESCDialog(Dialog owner, String title) {
this(owner, title, false);
}
public JESCDialog(Dialog owner, String title, boolean modal) {
super(owner, title, modal);
}
public JESCDialog(Dialog owner, String title, boolean modal, GraphicsConfiguration gc) {
super(owner, title, modal, gc);
}
public JESCDialog(Window owner, ModalityType modalityType) {
this(owner, "", modalityType);
}
public JESCDialog(Window owner, String title, Dialog.ModalityType modalityType) {
super(owner, title, modalityType);
}
public JESCDialog(Window owner, String title, Dialog.ModalityType modalityType, GraphicsConfiguration gc) {
super(owner, title, modalityType, gc);
}
public void closeDialog() {
// TODO Auto-generated method stub
setVisible(false);
dispose();
}
@Override
protected JRootPane createRootPane() {
JRootPane rootPane = new JRootPane();
// Escape key closes dialogs
KeyStroke stroke = KeyStroke.getKeyStroke(KeyEvent.VK_ESCAPE, 0);
rootPane.getInputMap(JComponent.WHEN_IN_FOCUSED_WINDOW).put(stroke, "escapeKey");
rootPane.getActionMap().put("escapeKey", new AbstractActionExtension());
return rootPane;
}
@SuppressWarnings("serial")
private final class AbstractActionExtension extends AbstractAction {
@Override
public void actionPerformed(ActionEvent e) {
setVisible(false);
dispose();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy